


What are the advantages and application scenarios of polymorphism in Golang?
Golang is a statically typed programming language with powerful polymorphic features. Polymorphism can be achieved through the use of interfaces. Polymorphism is one of the important features of object-oriented programming. It allows different types of objects to operate through the same interface, improving code reusability and scalability. In Golang, polymorphism can be achieved through interfaces. The following will introduce the advantages and common application scenarios of polymorphism in Golang, and give specific code examples.
Advantages of polymorphism:
- Code reuse: Through polymorphism, different types can be abstracted, so that the same operation can be applied to different types of objects. This can reduce the need to write the same code repeatedly and improve code reusability.
- Extensibility: Implementing polymorphism through interfaces can easily extend program functions. When a program needs to introduce a new type, it only needs to implement the corresponding interface without modifying the existing code. This extensibility allows the code to be more flexible in response to changes in requirements.
- Substitutability: Polymorphism makes objects substitutable, that is, one object can be replaced by another object as long as they share the same interface. This fungibility makes program design more flexible and able to cope with different object contexts.
Polymorphic application scenarios:
- Interface implementation: In Golang, polymorphism can be achieved through interfaces. Define an interface, then have multiple types implement the interface, and provide different concrete implementations for each type. By referencing objects of different types through interfaces, you can call their common methods. This allows you to use the same code logic for different types of objects.
The following is an example showing code that implements polymorphism through interfaces:
package main import "fmt" // 定义一个接口 type Programmer interface { Work() } // 定义两个结构体,分别实现Programmer接口 type GolangProgrammer struct{} func (g *GolangProgrammer) Work() { fmt.Println("I am a Golang programmer.") } type PythonProgrammer struct{} func (p *PythonProgrammer) Work() { fmt.Println("I am a Python programmer.") } func main() { // 使用Programmer接口引用不同类型的对象 var p Programmer p = &GolangProgrammer{} p.Work() p = &PythonProgrammer{} p.Work() }
- Interface arguments: In Golang, functions can accept arguments of interface type , to process different types of objects. Through interface arguments, you can pass objects of different types to functions and call the same method through the interface inside the function. This allows you to use the same function processing logic for different types of objects.
The following is an example showing code that implements polymorphism through interface parameters:
package main import "fmt" // 定义一个接口 type Programmer interface { Work() } // 定义一个函数,接受Programmer接口类型的实参 func DoWork(p Programmer) { p.Work() } type GolangProgrammer struct{} func (g *GolangProgrammer) Work() { fmt.Println("I am a Golang programmer.") } type PythonProgrammer struct{} func (p *PythonProgrammer) Work() { fmt.Println("I am a Python programmer.") } func main() { // 创建不同类型的对象 goProg := &GolangProgrammer{} pythonProg := &PythonProgrammer{} // 调用DoWork函数,并传递不同类型的对象 DoWork(goProg) DoWork(pythonProg) }
The above example code demonstrates the advantages and application scenarios of polymorphism in Golang. Through the use of interfaces, we can easily reuse and expand code, making the program more flexible and scalable. In actual development, rational application of polymorphism can improve the readability and maintainability of code, and can also improve development efficiency.
The above is the detailed content of What are the advantages and application scenarios of polymorphism in Golang?. For more information, please follow other related articles on the PHP Chinese website!
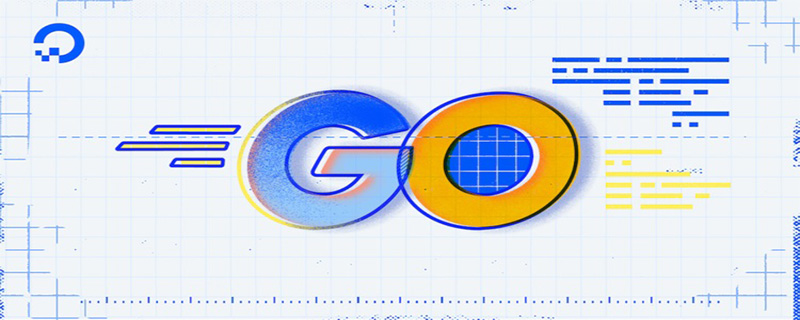
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
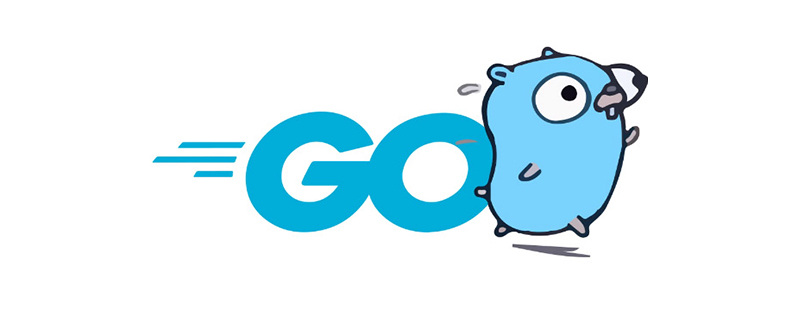
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
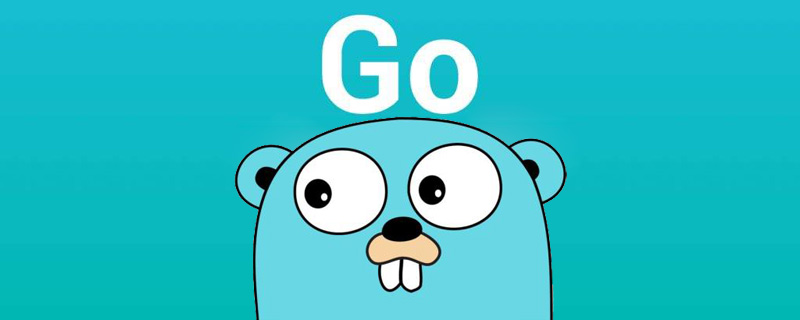
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
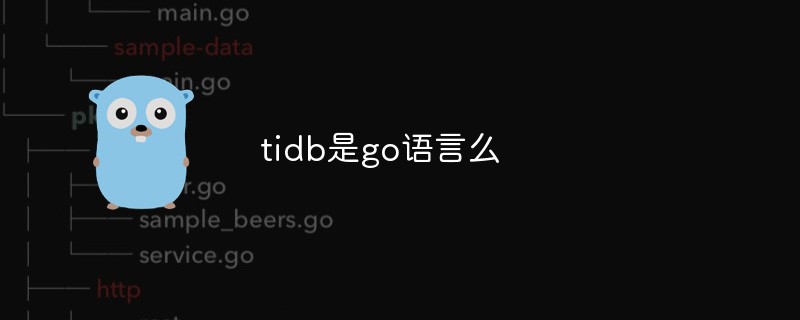
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
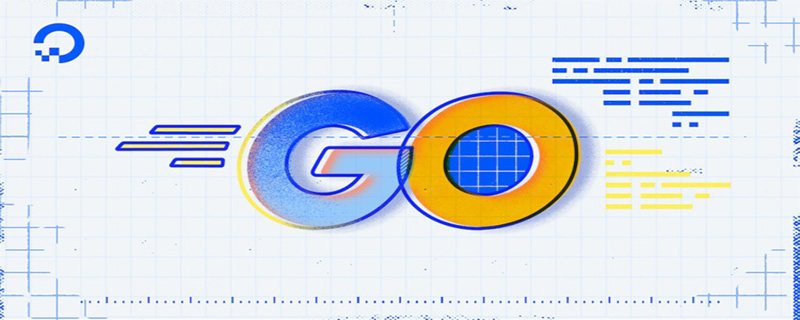
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
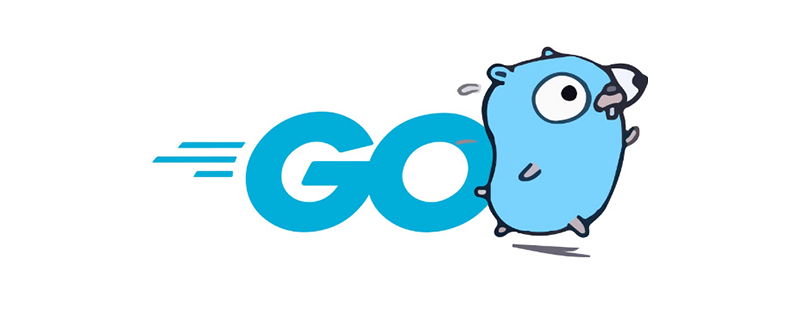
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
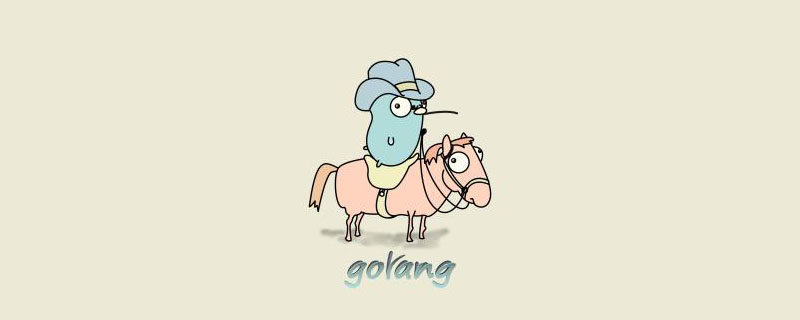
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
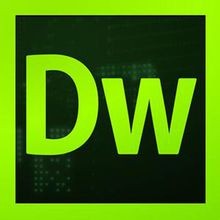
Dreamweaver CS6
Visual web development tools
