Solving data loss issues in Go language Websocket applications
In Go language Websocket applications, data loss is a common problem. Since Websocket uses an asynchronous transmission method, data packets may be lost or damaged during transmission. In this case, how to solve the problem of data loss is a challenge that every developer needs to face.
This article will introduce some methods to solve the problem of data loss in Go language Websocket applications, and provide specific code examples so that readers can quickly understand how to deal with these problems.
- Using the cache area
In Websocket applications, you can use the cache area to save data so that if the data is lost during transmission, it can be retransmitted in time. In Go language, you can use channels as buffer areas. The following is a sample code that uses channel as the buffer area:
func readPump(conn *websocket.Conn, ch chan []byte) { for { _, message, err := conn.ReadMessage() if err != nil { break } ch <- message } close(ch) } func writePump(conn *websocket.Conn, ch chan []byte) { for message := range ch { err := conn.WriteMessage(websocket.TextMessage, message) if err != nil { break } } conn.Close() } func main() { ch := make(chan []byte, 10) // 启动读取协程 go readPump(conn, ch) // 启动写入协程 go writePump(conn, ch) }
In this code, the readPump function will save the read message to the buffer area ch; the writePump function will read from the buffer area ch. message and written to the connection. If a message is lost during transmission, it is saved in the buffer for retransmission.
- Implementing data fragmentation
In Websocket applications, the size of data packets is limited. If the size of the data packet exceeds the limit, it will be cut into Multiple small packets are transmitted. In order to avoid data loss, data fragmentation can be implemented in the application to cut the data packet into multiple small data fragments for transmission. The following is a sample code to implement data fragmentation:
func writeMessage(conn *websocket.Conn, message []byte) error { messageSize := len(message) if messageSize > maxMessageSize { return errors.New("Message too large") } // 计算分片数量 fragmentSize := (messageSize / maxFragmentSize) + 1 for i := 0; i < fragmentSize; i++ { start := i * maxFragmentSize end := start + maxFragmentSize // 切割数据片段 if end > messageSize { end = messageSize } fragment := message[start:end] // 写入分片 err := conn.WriteMessage(websocket.TextMessage, fragment) if err != nil { return err } } return nil } func main() { message := []byte("Hello, world!") err := writeMessage(conn, message) if err != nil { log.Println(err) } }
In this code, the writeMessage function will cut the message into multiple data fragments for transmission. If a message is lost in transit, only partial data fragments are lost, not the entire message.
- Implementing data packet verification
In Websocket applications, in order to avoid errors in data packets during transmission, data packets can be verified. Verification information can be added to the data packet. After receiving the data packet, the receiver will verify it based on the verification information. If the verification fails, the sender will be asked to resend the data packet. The following is a sample code to implement data packet verification:
type Message struct { ID int `json:"id"` Data []byte `json:"data"` Checksum uint16 `json:"checksum"` } func writeMessage(conn *websocket.Conn, message Message) error { // 计算校验和 checksum := calculateChecksum(message.Data) // 添加校验和信息 message.Checksum = checksum // 序列化消息 body, err := json.Marshal(message) if err != nil { return err } // 发送消息 err = conn.WriteMessage(websocket.TextMessage, body) if err != nil { return err } return nil } func readMessage(conn *websocket.Conn) (Message, error) { var message Message // 接收消息 _, body, err := conn.ReadMessage() if err != nil { return message, err } // 反序列化消息 err = json.Unmarshal(body, &message) if err != nil { return message, err } // 校验消息 if message.Checksum != calculateChecksum(message.Data) { return message, errors.New("Checksum error") } return message, nil } func calculateChecksum(data []byte) uint16 { checksum := uint16(0) for i := 0; i < len(data); i++ { checksum += uint16(data[i]) } return checksum } func main() { message := Message{ ID: 1, Data: []byte("Hello, world!"), } err := writeMessage(conn, message) if err != nil { log.Println(err) } rcvMessage, err := readMessage(conn) if err != nil { log.Println(err) } else { log.Println(rcvMessage) } }
In this code, the writeMessage function will add the checksum information to the data packet, and the readMessage function will after receiving the data packet, based on the verification and verify. If the checksums do not match, the packet was lost or altered during transmission.
The above is the detailed content of Solving data loss issues in Go language Websocket applications. For more information, please follow other related articles on the PHP Chinese website!
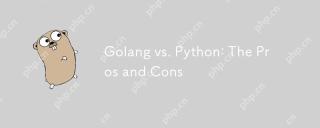
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
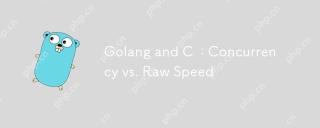
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
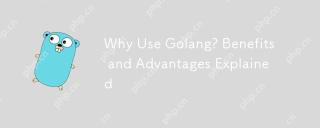
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
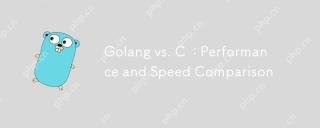
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
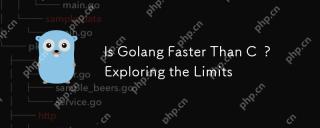
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
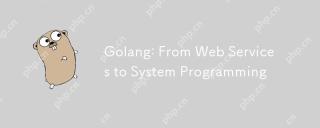
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
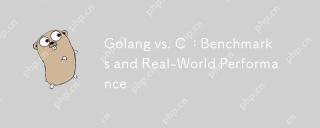
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
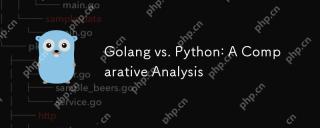
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
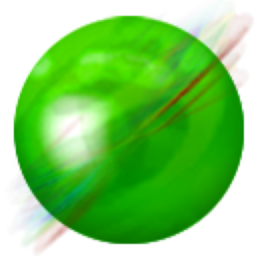
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
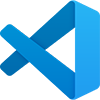
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version