Using Redis to achieve distributed cache consistency
In modern distributed systems, cache plays a very important role. It can greatly reduce the frequency of system access to the database and improve system performance and throughput. In a distributed system, in order to ensure cache consistency, we need to solve the problem of data synchronization between multiple nodes. In this article, we will introduce how to use Redis to achieve distributed cache consistency and give specific code examples.
Redis is a high-performance key-value database that supports persistence, replication, clustering and other functions. We can use the Pub/Sub function provided by Redis to achieve data consistency in distributed cache.
First, we need to create a central node to coordinate data synchronization between cache nodes. This central node can be an independent Redis instance, or one of the nodes specified in a configuration file.
On each cache node, we need to implement two key functions: subscribe() and publish(). Among them, the subscribe() function is used to listen to the subscription channel on the central node and trigger the corresponding callback function when a message is received; the publish() function is used to publish messages to the central node.
Next, we give a pseudo code to demonstrate how to use Redis to achieve distributed cache consistency in Python:
import redis # 初始化Redis连接 conn = redis.Redis() # 定义订阅频道名称 channel = 'cache_channel' # 订阅回调函数 def callback(message): # 处理接收到的消息 print('Received message:', message) # 订阅频道 def subscribe(): pubsub = conn.pubsub() pubsub.subscribe(**{channel: callback}) thread = pubsub.run_in_thread(sleep_time=0.001, daemon=True) # 发布消息 def publish(message): conn.publish(channel, message) # 示例使用 if __name__ == '__main__': # 在缓存节点上启动订阅 subscribe() # 在其他地方可以使用publish()函数发布消息 publish('Hello world!') # 阻塞主线程,保持订阅 while True: pass
In the above code, we use the redis-py library to work with Redis interacts. First, we create a Redis connection object conn. Then, a subscription channel name channel and a subscription callback function callback are defined. In the subscribe() function, we use Redis's pubsub() method to create a Pub/Sub object pubsub, and specify the subscription channel and callback function. Next, we use the run_in_thread() method to open a new thread for subscription, so that we can monitor the central node's messages in real time. In the publish() function, we use the publish() method of Redis to publish messages to the central node.
In actual applications, we can further encapsulate the subscribe() and publish() functions as needed, such as adding cached read and write operations, as well as exception handling, etc.
Through the above code examples, we successfully used Redis to achieve distributed cache consistency. The central node keeps the data status between cache nodes consistent by subscribing and publishing messages. This approach can effectively reduce database access and improve system performance and scalability.
Summary:
This article introduces the method of using Redis to achieve distributed cache consistency, and gives specific code examples. By using the Pub/Sub function of Redis, we can easily achieve data synchronization between cache nodes. This method can greatly improve the performance and scalability of the system and is an indispensable part of the distributed system. In order to adapt to different business needs, we can further optimize and customize the code.
The above is the detailed content of Using Redis to achieve distributed cache consistency. For more information, please follow other related articles on the PHP Chinese website!
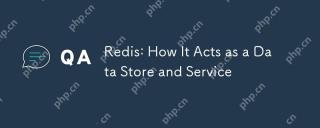
Redisactsasbothadatastoreandaservice.1)Asadatastore,itusesin-memorystorageforfastoperations,supportingvariousdatastructureslikekey-valuepairsandsortedsets.2)Asaservice,itprovidesfunctionalitieslikepub/submessagingandLuascriptingforcomplexoperationsan
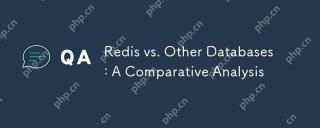
Compared with other databases, Redis has the following unique advantages: 1) extremely fast speed, and read and write operations are usually at the microsecond level; 2) supports rich data structures and operations; 3) flexible usage scenarios such as caches, counters and publish subscriptions. When choosing Redis or other databases, it depends on the specific needs and scenarios. Redis performs well in high-performance and low-latency applications.
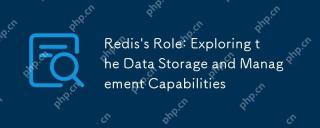
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
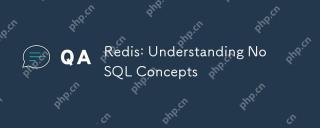
Redis is a NoSQL database suitable for efficient storage and access of large-scale data. 1.Redis is an open source memory data structure storage system that supports multiple data structures. 2. It provides extremely fast read and write speeds, suitable for caching, session management, etc. 3.Redis supports persistence and ensures data security through RDB and AOF. 4. Usage examples include basic key-value pair operations and advanced collection deduplication functions. 5. Common errors include connection problems, data type mismatch and memory overflow, so you need to pay attention to debugging. 6. Performance optimization suggestions include selecting the appropriate data structure and setting up memory elimination strategies.
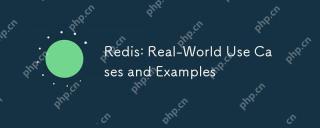
The applications of Redis in the real world include: 1. As a cache system, accelerate database query, 2. To store the session data of web applications, 3. To implement real-time rankings, 4. To simplify message delivery as a message queue. Redis's versatility and high performance make it shine in these scenarios.
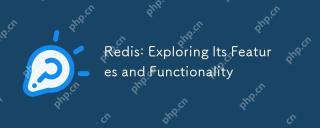
Redis stands out because of its high speed, versatility and rich data structure. 1) Redis supports data structures such as strings, lists, collections, hashs and ordered collections. 2) It stores data through memory and supports RDB and AOF persistence. 3) Starting from Redis 6.0, multi-threaded I/O operations have been introduced, which has improved performance in high concurrency scenarios.
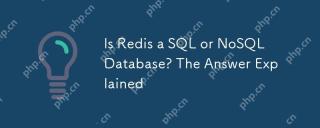
RedisisclassifiedasaNoSQLdatabasebecauseitusesakey-valuedatamodelinsteadofthetraditionalrelationaldatabasemodel.Itoffersspeedandflexibility,makingitidealforreal-timeapplicationsandcaching,butitmaynotbesuitableforscenariosrequiringstrictdataintegrityo
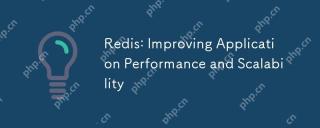
Redis improves application performance and scalability by caching data, implementing distributed locking and data persistence. 1) Cache data: Use Redis to cache frequently accessed data to improve data access speed. 2) Distributed lock: Use Redis to implement distributed locks to ensure the security of operation in a distributed environment. 3) Data persistence: Ensure data security through RDB and AOF mechanisms to prevent data loss.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
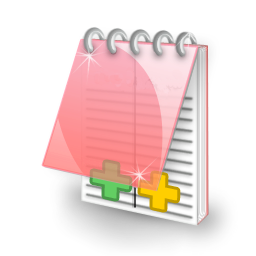
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use
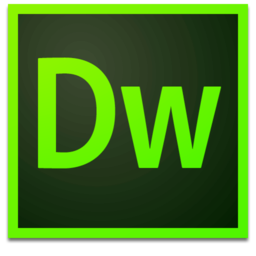
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
