Redis: Improving Application Performance and Scalability
Redis improves application performance and scalability by caching data, implementing distributed locking and data persistence. 1) Cache data: Use Redis to cache frequently accessed data to improve data access speed. 2) Distributed lock: Use Redis to implement distributed locks to ensure the security of operation in a distributed environment. 3) Data persistence: Ensure data security through RDB and AOF mechanisms to prevent data loss.
introduction
In today's Internet age, application performance and scalability have become one of the most concerned topics for developers. Redis, as an open source memory data structure storage system, has become a powerful tool to improve application performance and scalability with its fast speed, rich data structure and diverse application scenarios. This article will explore in-depth how Redis helps us achieve these goals and share some of my experiences and experiences using Redis in actual projects.
By reading this article, you will learn about the basic concepts of Redis, how it works, and how to use Redis to optimize your application. You will learn how to use Redis to cache data, implement distributed locks, and how to use Redis's persistence mechanism to ensure data security. I hope these contents can provide you with some practical guidance and inspiration.
Review of basic knowledge
Redis is a memory-based key-value storage system that supports multiple data types, such as strings, lists, collections, hash tables, etc. It's very fast, mainly due to all its data being stored in memory, rather than traditional disk storage. Redis can not only serve as a cache, but also as a database, message broker and other roles.
Before using Redis, it is necessary to understand some basic concepts, such as key-value pairs, expiration time, transactions, etc. These concepts will help you better understand how Redis works and application scenarios.
Core concept or function analysis
The role and advantages of Redis
The main function of Redis is to accelerate data access and improve application scalability. Its advantages include:
- Fast speed : Redis reads and writes very fast, usually at the microsecond level because data is stored in memory.
- Rich data structures : Redis supports a variety of data structures, such as strings, lists, collections, hash tables, etc., which makes it very flexible in various application scenarios.
- Persistence : Redis provides two persistence mechanisms: RDB and AOF, ensuring that data will not be lost after restarting.
- High Availability : Redis can achieve high availability and failover through master-slave replication and sentinel mechanisms.
A simple example of using Redis:
import redis # Connect to Redis server r = redis.Redis(host='localhost', port=6379, db=0) # Set a key-value pair r.set('my_key', 'Hello, Redis!') # Get key value = r.get('my_key') print(value) # Output: b'Hello, Redis!'
How Redis works
The working principle of Redis can be understood from the following aspects:
- Memory storage : Redis stores all data in memory, which makes data access very fast.
- Single-threaded model : Redis uses a single-threaded model to handle client requests, which avoids the complexity and performance problems caused by multithreading.
- Event-driven : Redis uses event-driven mechanism to handle client requests, which improves the system's response speed.
- Persistence mechanism : Redis realizes data persistence through RDB and AOF mechanisms to ensure data security.
When using Redis, you need to pay attention to its memory usage, because all data is stored in memory, which may cause memory overflow. Memory can be managed by setting maximum memory limits and phasing policies.
Example of usage
Basic usage
The basic usage of Redis includes setting and obtaining key-value pairs, list operations, collection operations, etc. Here is an example of caching using Redis:
import redis r = redis.Redis(host='localhost', port=6379, db=0) # Cache a user_info = {'name': 'John', 'age': 30} r.hmset('user:1', user_info) # Get user information user_info = r.hgetall('user:1') print(user_info) # Output: {b'name': b'John', b'age': b'30'}
This example shows how to use Redis to cache user information and improve data access speed.
Advanced Usage
Advanced usage of Redis includes distributed locks, publish subscriptions, geolocation and other features. Here is an example of using Redis to implement distributed locks:
import redis import time r = redis.Redis(host='localhost', port=6379, db=0) def acquire_lock(lock_name, acquire_time=10): identifier = str(time.time()) lock_name = 'lock:' lock_name if r.setnx(lock_name, identifier): r.expire(lock_name, acquire_time) return identifier return False def release_lock(lock_name, identifier): lock_name = 'lock:' lock_name pipe = r.pipeline(True) While True: try: pipe.watch(lock_name) if pipe.get(lock_name) == identifier: pipe.multi() pipe.delete(lock_name) pipe.execute() return True pipe.unwatch() break except redis.exceptions.WatchError: pass return False # Use distributed lock lock_id = acquire_lock('my_lock') if lock_id: print('Lock acquired') # Perform some operations time.sleep(5) release_lock('my_lock', lock_id) print('Lock released') else: print('Failed to acquire lock')
This example shows how to use Redis to implement distributed locking to ensure that multiple processes in a distributed environment do not perform certain operations at the same time.
Common Errors and Debugging Tips
Common errors when using Redis include connection problems, memory overflow, data consistency problems, etc. Here are some debugging tips:
- Connection problem : Make sure the Redis server is running and the network connection is normal. The connection can be tested through the
ping
command. - Memory overflow : Monitor Redis's memory usage, set maximum memory limits and elimination policies.
- Data consistency : Use Redis's transaction function to ensure data consistency and avoid data inconsistency caused by concurrent operations.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance and best practices of Redis. Here are some suggestions:
- Using cache : Redis is the most common use as a cache, using Redis to cache frequently accessed data can significantly improve the performance of your application.
- Data sharding : Through data sharding, data can be distributed among multiple Redis instances to improve the scalability of the system.
- Persistence optimization : Choose the appropriate persistence mechanism according to the needs of the application. RDB is suitable for scenarios with large data volumes, and AOF is suitable for scenarios that require high reliability.
- Monitoring and Tuning : Use Redis's monitoring tools to monitor its performance and tune it according to monitoring results, such as adjusting memory usage, adjusting network configuration, etc.
In my project experience, using Redis for caching and distributed locking greatly improves the performance and scalability of the application. Especially in the e-commerce system, Redis helps us achieve efficient product search and recommendation functions, greatly improving the user experience.
In short, Redis is a powerful and flexible tool that can help us improve the performance and scalability of our applications. Hope this article provides you with some useful guidance and inspiration to help you better use Redis in your project.
The above is the detailed content of Redis: Improving Application Performance and Scalability. For more information, please follow other related articles on the PHP Chinese website!
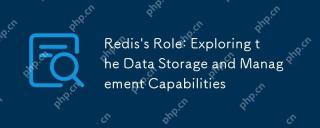
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
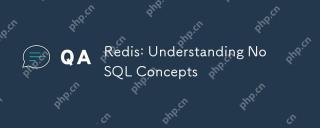
Redis is a NoSQL database suitable for efficient storage and access of large-scale data. 1.Redis is an open source memory data structure storage system that supports multiple data structures. 2. It provides extremely fast read and write speeds, suitable for caching, session management, etc. 3.Redis supports persistence and ensures data security through RDB and AOF. 4. Usage examples include basic key-value pair operations and advanced collection deduplication functions. 5. Common errors include connection problems, data type mismatch and memory overflow, so you need to pay attention to debugging. 6. Performance optimization suggestions include selecting the appropriate data structure and setting up memory elimination strategies.
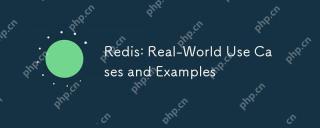
The applications of Redis in the real world include: 1. As a cache system, accelerate database query, 2. To store the session data of web applications, 3. To implement real-time rankings, 4. To simplify message delivery as a message queue. Redis's versatility and high performance make it shine in these scenarios.
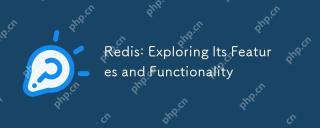
Redis stands out because of its high speed, versatility and rich data structure. 1) Redis supports data structures such as strings, lists, collections, hashs and ordered collections. 2) It stores data through memory and supports RDB and AOF persistence. 3) Starting from Redis 6.0, multi-threaded I/O operations have been introduced, which has improved performance in high concurrency scenarios.
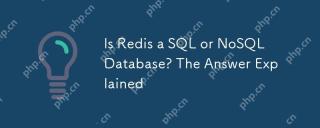
RedisisclassifiedasaNoSQLdatabasebecauseitusesakey-valuedatamodelinsteadofthetraditionalrelationaldatabasemodel.Itoffersspeedandflexibility,makingitidealforreal-timeapplicationsandcaching,butitmaynotbesuitableforscenariosrequiringstrictdataintegrityo
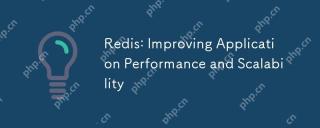
Redis improves application performance and scalability by caching data, implementing distributed locking and data persistence. 1) Cache data: Use Redis to cache frequently accessed data to improve data access speed. 2) Distributed lock: Use Redis to implement distributed locks to ensure the security of operation in a distributed environment. 3) Data persistence: Ensure data security through RDB and AOF mechanisms to prevent data loss.
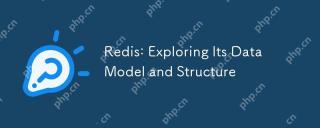
Redis's data model and structure include five main types: 1. String: used to store text or binary data, and supports atomic operations. 2. List: Ordered elements collection, suitable for queues and stacks. 3. Set: Unordered unique elements set, supporting set operation. 4. Ordered Set (SortedSet): A unique set of elements with scores, suitable for rankings. 5. Hash table (Hash): a collection of key-value pairs, suitable for storing objects.
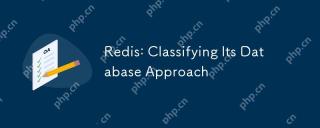
Redis's database methods include in-memory databases and key-value storage. 1) Redis stores data in memory, and reads and writes fast. 2) It uses key-value pairs to store data, supports complex data structures such as lists, collections, hash tables and ordered collections, suitable for caches and NoSQL databases.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.