How to develop a cache warm-up function using Redis and Objective-C
How to use Redis and Objective-C to develop cache preheating function
When developing Internet applications, in order to improve performance and response speed, we usually use cache to store Frequently accessed data. Cache warm-up is a common optimization strategy. By loading popular data into the cache in advance, you can avoid the waiting time when users access it for the first time. This article will introduce how to use Redis and Objective-C to develop cache preheating function, and provide specific code examples.
1. Introduction to Redis
Redis is an in-memory database that supports various data structures, such as strings, hash tables, lists, sets, ordered sets, etc. The advantage of Redis lies in its efficient reading and writing speed and rich functions. It is suitable for various scenarios such as caching, queues, and message publishing and subscription.
2. Objective-C and Redis connection
To use Objective-C to communicate with Redis, we can use a third-party library to achieve it. Here, we use the "redis-objc" library to connect to the Redis server.
The steps are as follows:
- Download the "redis-objc" library. You can search "redis-objc" on GitHub and download the source code, or install the library through CocoaPods.
- Configure connection information. Introduce the "RedisClient.h" header file into the project and set the address, port number and password of the Redis server (if any).
#import "RedisClient.h" NSString *const kRedisHost = @"127.0.0.1"; NSInteger const kRedisPort = 6379; NSString *const kRedisPassword = @"your_password";
- Connect to the Redis server. Where cache preheating is required, use the following code to connect to the Redis server:
RedisClient *client = [[RedisClient alloc] init]; [client connectToHost:kRedisHost port:kRedisPort password:kRedisPassword];
- Send instructions to the Redis server. We can use different instructions to operate the Redis server, such as SET, GET, etc. The following is an example of storing data into Redis:
NSString *key = @"myKey"; NSString *value = @"myValue"; [client set:key value:value];
- Disconnect from Redis. After completing the operation, use the following code to disconnect from the Redis server:
[client disconnect];
3. Implementation of cache preheating
Cache preheating refers to when the application starts or in a scheduled task. Load popular data into cache. In this way, when users access the data, they can avoid reading data from the database or other data sources and improve read performance.
The following is a sample code for cache preheating using Redis and Objective-C:
- (void)preloadCache { // 连接Redis服务器 RedisClient *client = [[RedisClient alloc] init]; [client connectToHost:kRedisHost port:kRedisPort password:kRedisPassword]; // 获取待预热的数据,这里以数据库中的数据为例 NSArray *hotDataArray = [self fetchHotDataFromDatabase]; // 将热门数据存入Redis for (NSDictionary *data in hotDataArray) { NSString *key = data[@"key"]; NSString *value = data[@"value"]; [client set:key value:value]; } // 断开与Redis的连接 [client disconnect]; } - (NSArray *)fetchHotDataFromDatabase { // 从数据库中获取热门数据的逻辑 // ... return hotDataArray; }
In the above code, we first connect to the Redis server and then get the hot data from the database. Next, store the data into Redis through a loop. Finally, disconnect from Redis.
Through the above code examples, we can see how to use Redis and Objective-C to implement the cache preheating function. By pre-loading popular data into the cache, you can significantly improve your app's performance and responsiveness. However, it should be noted that in actual applications, we also need to consider issues such as data update and invalidation to ensure the consistency and validity of cached data.
The above is the detailed content of How to develop a cache warm-up function using Redis and Objective-C. For more information, please follow other related articles on the PHP Chinese website!
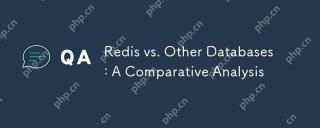
Compared with other databases, Redis has the following unique advantages: 1) extremely fast speed, and read and write operations are usually at the microsecond level; 2) supports rich data structures and operations; 3) flexible usage scenarios such as caches, counters and publish subscriptions. When choosing Redis or other databases, it depends on the specific needs and scenarios. Redis performs well in high-performance and low-latency applications.
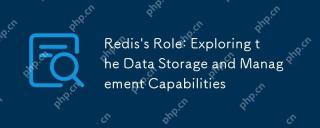
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
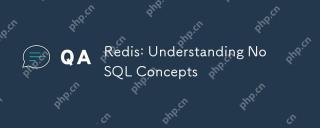
Redis is a NoSQL database suitable for efficient storage and access of large-scale data. 1.Redis is an open source memory data structure storage system that supports multiple data structures. 2. It provides extremely fast read and write speeds, suitable for caching, session management, etc. 3.Redis supports persistence and ensures data security through RDB and AOF. 4. Usage examples include basic key-value pair operations and advanced collection deduplication functions. 5. Common errors include connection problems, data type mismatch and memory overflow, so you need to pay attention to debugging. 6. Performance optimization suggestions include selecting the appropriate data structure and setting up memory elimination strategies.
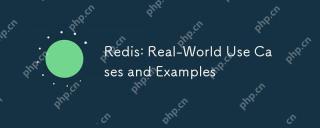
The applications of Redis in the real world include: 1. As a cache system, accelerate database query, 2. To store the session data of web applications, 3. To implement real-time rankings, 4. To simplify message delivery as a message queue. Redis's versatility and high performance make it shine in these scenarios.
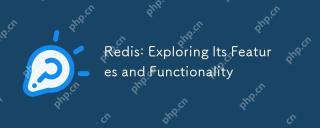
Redis stands out because of its high speed, versatility and rich data structure. 1) Redis supports data structures such as strings, lists, collections, hashs and ordered collections. 2) It stores data through memory and supports RDB and AOF persistence. 3) Starting from Redis 6.0, multi-threaded I/O operations have been introduced, which has improved performance in high concurrency scenarios.
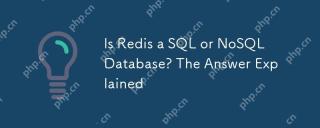
RedisisclassifiedasaNoSQLdatabasebecauseitusesakey-valuedatamodelinsteadofthetraditionalrelationaldatabasemodel.Itoffersspeedandflexibility,makingitidealforreal-timeapplicationsandcaching,butitmaynotbesuitableforscenariosrequiringstrictdataintegrityo
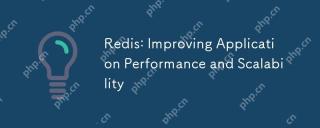
Redis improves application performance and scalability by caching data, implementing distributed locking and data persistence. 1) Cache data: Use Redis to cache frequently accessed data to improve data access speed. 2) Distributed lock: Use Redis to implement distributed locks to ensure the security of operation in a distributed environment. 3) Data persistence: Ensure data security through RDB and AOF mechanisms to prevent data loss.
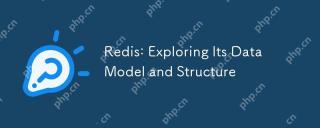
Redis's data model and structure include five main types: 1. String: used to store text or binary data, and supports atomic operations. 2. List: Ordered elements collection, suitable for queues and stacks. 3. Set: Unordered unique elements set, supporting set operation. 4. Ordered Set (SortedSet): A unique set of elements with scores, suitable for rankings. 5. Hash table (Hash): a collection of key-value pairs, suitable for storing objects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
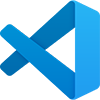
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!