In this article, we will learn how to get word frequency as a percentage in Python.
Suppose we have obtained a string input list. Now, we will find the percentage of each word in the given list of input strings.
formula
(Occurrence of X word / Total words) * 100
usage instructions
Use sum(), Counter(), join() and split() functions
Use join(), split() and count() functions
Use the countOf() function of the operator module.
Method 1: Use sum(), Counter(), join() and split() functions
join() is a string function in Python that is used to join sequence elements separated by string delimiters to form a string.
Counter() The function is a subclass that counts the number of hashable objects. It implicitly creates a hash table of iterable objects when called/invoked.
Algorithm (steps)
The following are the algorithms/steps to perform the required task:
Use the import keyword to import the Counter function from the collection module.
Create a variable to store the input list string and print the list.
Use the join() function to join all string elements of the input list.
Use the split() function (split the string into a list. The delimiter can be defined; the default delimiter is any whitespace character) to split the concatenated string into a list of words, and use Counter() Function gets word frequency as key-value pair
Use values() function to get all values (frequency/count) from Counter and use sum() function to get their sum (returns the sum of all values) in the iterable project).
Use the items() function to get the percentage of each word in the above counter words (returns a view object, i.e. it contains the key-value pairs of the dictionary, as tuples in a list).
Print the percentage of each word in the input list.
Example
is:Example
The following program uses the sum(), Counter(), join() and split() functions to return the percentage of each word in a given list of input strings –
# importing a Counter function from the collections module from collections import Counter # input list of strings inputList = ["hello tutorialspoint", "python codes", "tutorialspoint for python", "see python codes tutorialspoint"] print("Input list:\n", inputList) # Joining all the string elements of the list using the join() function join_string = " ".join(i for i in inputList) # splitting the joined string into a list of words and getting the # frequency of words as key-value pairs using Counter() function counter_words = Counter(join_string.split()) # getting all the values(frequencies/counts) from counter and # finding the total sum of them total_sum = sum(counter_words.values()) # getting the percentage of each word from the above counter words res_percentage = {key: value / total_sum for key, value in counter_words.items()} # printing the percentage of each word from the input list print("Percentage of each word from the input list:\n", res_percentage)
Output
When executed, the above program will generate the following output -
Input list: ['hello tutorialspoint', 'python codes', 'tutorialspoint for python', 'see python codes tutorialspoint'] Percentage of each word from the input list: {'hello': 0.09090909090909091, 'tutorialspoint': 0.2727272727272727, 'python': 0.2727272727272727, 'codes': 0.18181818181818182, 'for': 0.09090909090909091, 'see': 0.09090909090909091}
Method 2: Use join(), split() and count() functions
Algorithm (steps)
The following are the algorithms/steps to perform the required task:
Create an empty dictionary to store the result percentage/term frequency.
Use for loop to traverse the word list.
Use the if conditional statement to check whether the current element is not in the key of the dictionary, use the keys() function.
If the above condition is true, use the count() function to get the count of the key (word).
Divide it by the number of words to get the current word frequency and store it as a key in the new dictionary created above.
Print the percentage of each word in the input list.
Example
is:Example
The following program uses the join(), split() and count() functions to return the percentage of each word in a given list of input strings –
# input list of strings inputList = ["hello tutorialspoint", "python codes", "tutorialspoint for python", "see python codes tutorialspoint"] # joining all the elements of the list using join() join_string = " ".join(i for i in inputList) # splitting the joined string into a list of words listOfWords = join_string.split() # Creating an empty dictionary for storing the resultant percentages resDict = dict() # traversing through the list of words for item in listOfWords: # checking whether the current element is not in the keys of a dictionary if item not in resDict.keys(): # getting the percentage of a current word if the condition is true resDict[item] = listOfWords.count(item)/len(listOfWords) # printing the percentage of each word from the input list print("Percentage of each word from the input list:\n", resDict)
Output
When executed, the above program will generate the following output -
Percentage of each word from the input list: {'hello': 0.09090909090909091, 'tutorialspoint': 0.2727272727272727, 'python': 0.2727272727272727, 'codes': 0.18181818181818182, 'for': 0.09090909090909091, 'see': 0.09090909090909091}
Method 3: Use the countOf() function of the operator module
The Chinese translation ofExample
is:Example
The following program uses the countOf() function to return the percentage of each word in a given list of input strings -
import operator as op # input list of strings inputList = ["hello tutorialspoint", "python codes", "tutorialspoint for python", "see python codes tutorialspoint"] # joining all the elements of list using join() join_string = " ".join(i for i in inputList) # splitting the joined string into list of words listOfWords = join_string.split() resDict = dict() for item in listOfWords: # checking whether the current element is not in the keys of dictionary if item not in resDict.keys(): resDict[item] = op.countOf(listOfWords, item)/len(listOfWords) print("Percentage of each word from the input list:\n", resDict)
Output
When executed, the above program will generate the following output -
Percentage of each word from the input list: {'hello': 0.09090909090909091, 'tutorialspoint': 0.2727272727272727, 'python': 0.2727272727272727, 'codes': 0.18181818181818182, 'for': 0.09090909090909091, 'see': 0.09090909090909091}
in conclusion
In this article, we learned three different Python methods to calculate percent word frequency. We also learned how to use the operator module's new function countOf() to get the frequency of a list element.
The above is the detailed content of Python program to get percentage of word frequency. For more information, please follow other related articles on the PHP Chinese website!
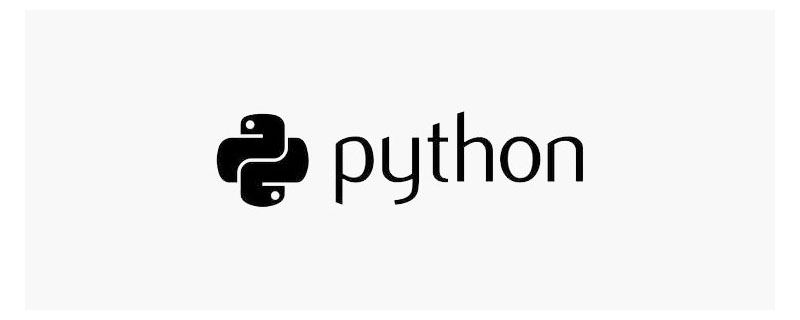
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
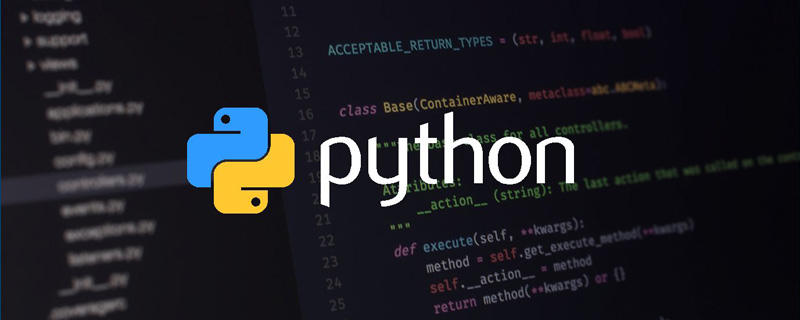
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
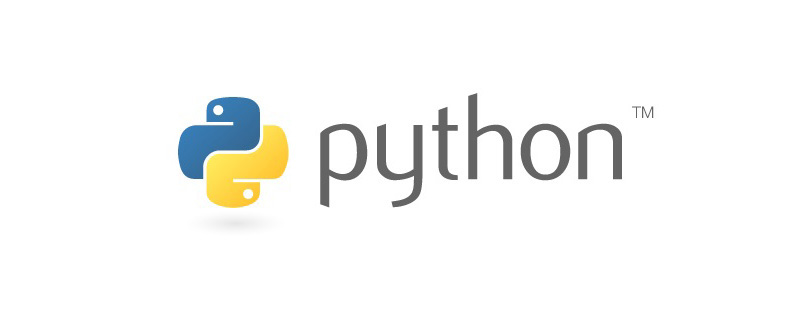
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
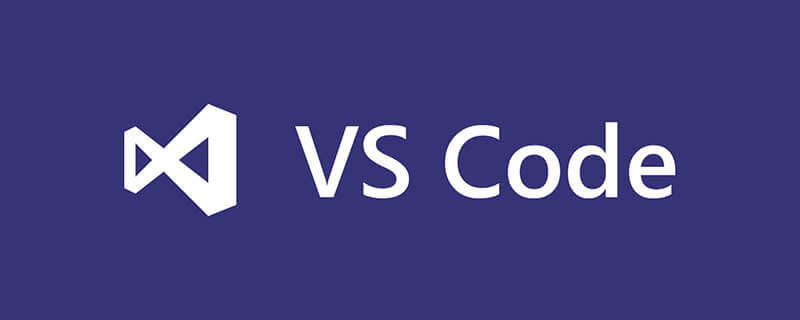
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
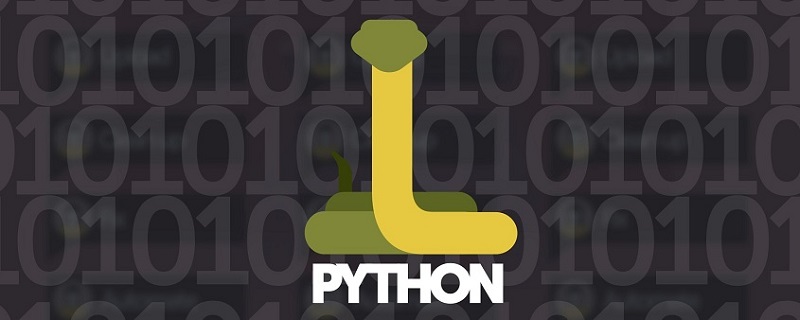
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
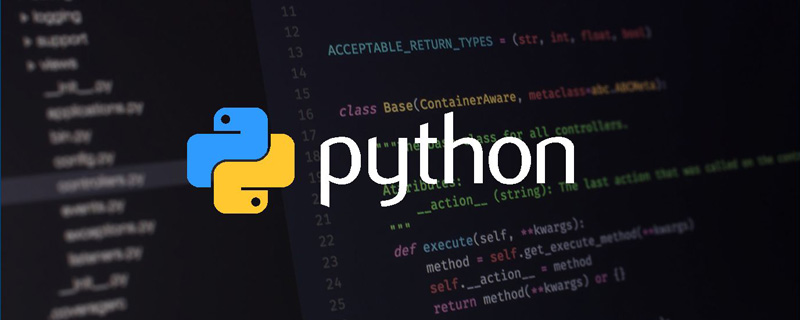
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
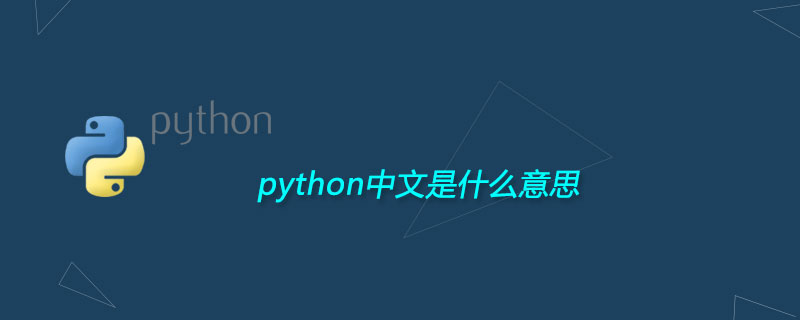
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
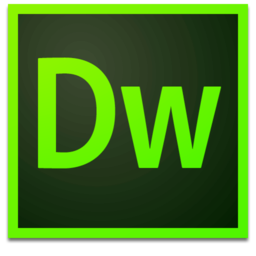
Dreamweaver Mac version
Visual web development tools
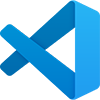
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
