


Does the greatest common divisor of any subset of the array belong to the given array?
Here we will see an interesting question. There is a set containing N elements. We must generate an array such that the GCD of any subset of the array lies within the given set of elements. Another limitation is that the resulting array should not exceed three times the length of the GCD collection. For example, if there are 4 numbers {2, 4, 6, 12}, then an array will be {2, 2, 4, 2, 6, 2, 12}
To solve this problem, we have to First the list is sorted, then if the GCD is the same as the smallest element of the given collection, the array is created by simply placing the GCD between each element. Otherwise the array cannot be formed.
Algorithm
generateArray(arr, n)
Begin answer := empty array gcd := gcd of array arr if gcd is same as the min element of arr, then for each element e in arr, do append gcd into answer append e into answer done display answer else array cannot be formed end if End
Example
#include<iostream> #include<vector> #include<set> using namespace std; int gcd(int a, int b) { if (a == 0) return b; return gcd(b % a, a); } int getGCDofArray(vector<int> arr) { int result = arr[0]; for (int i = 1; i < arr.size(); i++) result = gcd(arr[i], result); return result; } void generateArray(vector<int> arr) { vector<int> answer; int GCD_of_array = getGCDofArray(arr); if(GCD_of_array == arr[0]) { //if gcd is same as minimum element answer.push_back(arr[0]); for(int i = 1; i < arr.size(); i++) { //push min before each element answer.push_back(arr[0]); answer.push_back(arr[i]); } for (int i = 0; i < answer.size(); i++) cout << answer[i] << " "; } else cout << "No array can be build"; } int main() { int n = 4; int data[]= {2, 4, 6, 12}; set<int> GCD(data, data + n); vector<int> arr; set<int>::iterator it; for(it = GCD.begin(); it!= GCD.end(); ++it) arr.push_back(*it); generateArray(arr); }
Output
2 2 4 2 6 2 12
The above is the detailed content of Does the greatest common divisor of any subset of the array belong to the given array?. For more information, please follow other related articles on the PHP Chinese website!
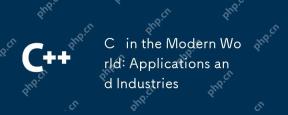
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
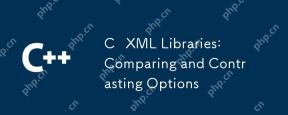
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
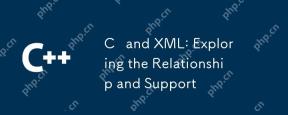
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
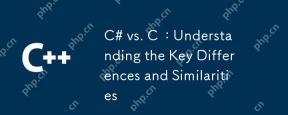
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
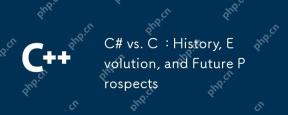
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
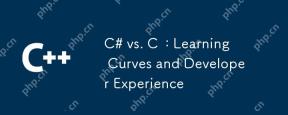
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
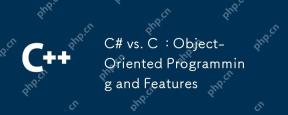
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
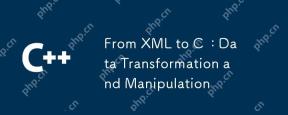
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
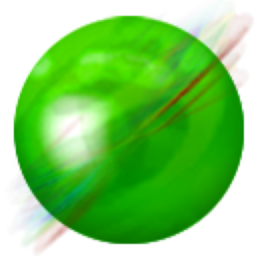
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
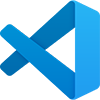
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version