


Python script to monitor network connections and save to log file
Monitoring network connections is critical to ensuring the stability and security of your computer system. Whether you are a network administrator or an individual user, having a way to track network connections and log related information can be invaluable. In this blog post, we will explore how to create a Python script to monitor a network connection and save the data to a log file.
By leveraging the power of Python and its rich libraries, we can develop a script to periodically check the network status, capture relevant details (such as IP address, timestamp, and connection status), and store them in a log file for future reference. This script not only provides real-time insights into network connections, but also provides historical records that aid in troubleshooting and analysis.
Set up environment
Before we start writing a Python script to monitor network connections, we need to make sure that our environment is set up correctly. Here are the steps to follow−
Install Python − If Python is not installed on your system, please visit the official Python website (https://www.python.org) and Download the latest version for your operating system. Follow the installation instructions provided to complete the setup.
Install the required libraries − We will use the socket library in Python to establish network connections and retrieve information. Fortunately, this library is part of the standard Python library, so no additional installation is required.
Create Project Directory− It is a good practice to create a dedicated directory for our project. Open a terminal or command prompt and navigate to the desired location on your system. Create a new directory using the following command:
mkdir network-monitoring
Set up a virtual environment (optional) − Although not mandatory, it is recommended to create a virtual environment for our project. This allows us to isolate project dependencies and avoid conflicts with other Python packages on the system. To set up a virtual environment, run the following command:
cd network-monitoring python -m venv venv
Activate the virtual environment− Activate the virtual environment by running the command appropriate for your operating system:
For Windows −
venv\Scripts\activate
For macOS/Linux −
source venv/bin/activate
After the environment is set up, we can start writing Python scripts to monitor network connections. In the next section, we'll delve deeper into the code implementation and explore the necessary steps to achieve our goals.
Monitoring network connections
To monitor the network connection and save the information to a log file, we will follow the following steps -
Import the required libraries− First import the necessary libraries in the Python script−
import socket import datetime
设置日志文件 −我们将创建一个日志文件来存储网络连接信息。添加以下代码以创建带有时间戳的日志文件−
log_filename = "network_log.txt" # Generate timestamp for the log file timestamp = datetime.datetime.now().strftime("%Y-%m-%d_%H-%M-%S") log_filename = f"{timestamp}_{log_filename}" # Create or open the log file in append mode log_file = open(log_filename, "a")
监控网络连接− 使用循环持续监控网络连接。在每次迭代中,检索当前连接并将其写入日志文件。下面是实现此目的的示例代码片段−
while True: # Get the list of network connections connections = socket.net_connections() # Write the connections to the log file log_file.write(f"Timestamp: {datetime.datetime.now()}\n") for connection in connections: log_file.write(f"{connection}\n") log_file.write("\n") # Wait for a specified interval (e.g., 5 seconds) before checking again time.sleep(5)
关闭日志文件− 监控网络连接后,关闭日志文件以确保正确保存数据非常重要。添加以下代码以关闭文件−
log_file.close()
异常处理−最好处理脚本执行期间可能发生的任何异常。将代码包含在 try- except 块内以捕获并处理任何潜在错误−
try: # Code for monitoring network connections except Exception as e: print(f"An error occurred: {e}") log_file.close()
现在我们有了 Python 脚本来监视网络连接并将信息保存到日志文件中,让我们运行该脚本并观察结果。
(注意− 提供的代码是演示该概念的基本实现。您可以根据您的具体要求进一步增强它。)
执行脚本并解释日志文件
要执行Python脚本来监视网络连接并将信息保存到日志文件中,请按照以下步骤操作 -
保存脚本 − 使用 .py 扩展名保存脚本,例如 network_monitor.py。
运行脚本− 打开终端或命令提示符并导航到保存脚本的目录。使用以下命令运行脚本:
python network_monitor.py
监控网络连接− 脚本开始运行后,它将按照指定的时间间隔(例如每 5 秒)持续监控网络连接。连接信息将实时写入日志文件。
停止脚本− 要停止脚本,请在终端或命令提示符中按 Ctrl+C。
解释日志文件 − 停止脚本后,您可以打开日志文件来检查记录的网络连接信息。日志文件中的每个条目代表特定时间戳的网络连接快照。
The timestamp indicates the time when the network connection was recorded.
Each connection entry provides details such as local address, remote address, and connection status.
Analyzing log files can help identify patterns, troubleshoot network problems, or track the history of network connections.
Custom script (optional)− The provided script is a basic implementation. You can customize it to suit your specific requirements. For example, you can modify the time interval between network connection checks, filter connections based on specific criteria, or extend the script's functionality to include additional network monitoring capabilities.
in conclusion
By using a Python script to monitor network connections and save the information to a log file, you can gain valuable insights into your system's network activity. Whether it's troubleshooting, security analysis, or performance optimization, this script provides a useful tool for network monitoring and analysis.
The above is the detailed content of Python script to monitor network connections and save to log file. For more information, please follow other related articles on the PHP Chinese website!
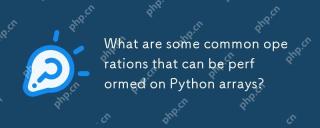
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
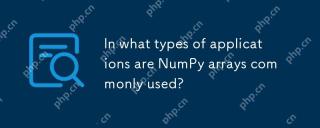
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
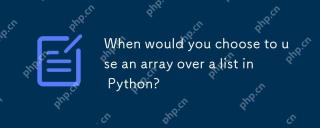
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf
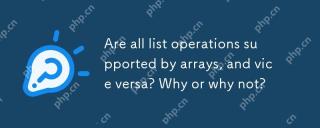
No,notalllistoperationsaresupportedbyarrays,andviceversa.1)Arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,whichimpactsperformance.2)Listsdonotguaranteeconstanttimecomplexityfordirectaccesslikearraysdo.
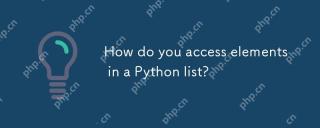
ToaccesselementsinaPythonlist,useindexing,negativeindexing,slicing,oriteration.1)Indexingstartsat0.2)Negativeindexingaccessesfromtheend.3)Slicingextractsportions.4)Iterationusesforloopsorenumerate.AlwayschecklistlengthtoavoidIndexError.
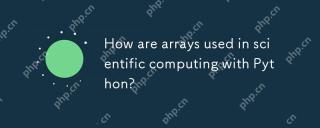
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
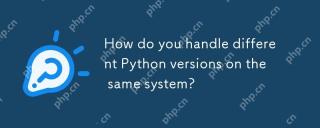
You can manage different Python versions by using pyenv, venv and Anaconda. 1) Use pyenv to manage multiple Python versions: install pyenv, set global and local versions. 2) Use venv to create a virtual environment to isolate project dependencies. 3) Use Anaconda to manage Python versions in your data science project. 4) Keep the system Python for system-level tasks. Through these tools and strategies, you can effectively manage different versions of Python to ensure the smooth running of the project.
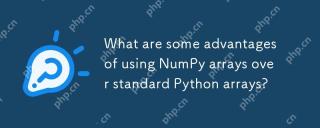
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
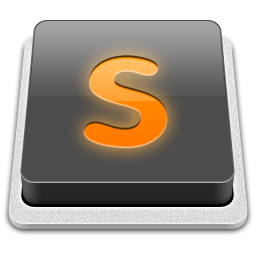
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
