


Are all list operations supported by arrays, and vice versa? Why or why not?
No, not all list operations are supported by arrays, and vice versa. 1) Arrays do not support dynamic operations like append or insert without resizing, which impacts performance. 2) Lists do not guarantee constant time complexity for direct access like arrays do.
When it comes to the question of whether all list operations are supported by arrays, and vice versa, the answer is a nuanced no. Let's dive into why this is the case and explore the fascinating world of data structures.
Arrays and lists are fundamental data structures in programming, but they have distinct characteristics that affect their operations. Arrays are fixed-size, homogeneous collections of elements stored in contiguous memory locations. Lists, on the other hand, are dynamic, heterogeneous collections that can grow or shrink as needed.
The reason not all list operations are supported by arrays is primarily due to their fixed size. For instance, operations like append
or insert
in a list, which dynamically add elements, are not directly supported by arrays without resizing, which can be costly in terms of performance. Conversely, arrays support direct access to elements by index with constant time complexity, which lists might not guarantee due to their dynamic nature.
Now, let's delve deeper into the world of arrays and lists, exploring their operations, sharing some personal experiences, and providing code examples that reflect my own style.
Arrays are like the sturdy, reliable workhorses of data structures. I remember working on a project where we needed to process large datasets quickly. Arrays were our go-to choice because of their predictable memory layout and fast access times. Here's a simple example in Java to illustrate how arrays work:
int[] numbers = new int[5]; numbers[0] = 10; numbers[1] = 20; numbers[2] = 30; numbers[3] = 40; numbers[4] = 50; for (int i = 0; i < numbers.length; i ) { System.out.println("Element at index " i ": " numbers[i]); }
This code showcases the simplicity and efficiency of arrays. However, if you try to add a sixth element, you'll run into an ArrayIndexOutOfBoundsException
. This limitation is where lists shine.
Lists, on the other hand, are like the Swiss Army knives of data structures. They offer flexibility and ease of use, which I found invaluable when working on a project that required frequent modifications to the data structure. Here's an example in Python to demonstrate the power of lists:
numbers = [10, 20, 30, 40, 50] numbers.append(60) # Adding a new element numbers.insert(0, 5) # Inserting at the beginning print("Updated list:", numbers)
This code shows how lists can grow dynamically, something arrays can't do without manual resizing. However, this flexibility comes at a cost. Operations like insert
at the beginning of a list can be O(n) in time complexity, whereas accessing an element in an array is always O(1).
When it comes to operations, arrays support direct access, modification, and iteration over elements. Lists, in addition to these, support operations like append
, insert
, remove
, and pop
, which are not directly supported by arrays without additional logic.
One of the pitfalls I've encountered with arrays is the need to manually manage their size. In a project where we were dealing with real-time data, we had to implement a custom resizing mechanism for our arrays, which added complexity to our code. Here's a snippet of how we handled it:
public class DynamicArray { private int[] array; private int size; private int capacity; public DynamicArray(int initialCapacity) { this.capacity = initialCapacity; this.array = new int[capacity]; this.size = 0; } public void add(int element) { if (size == capacity) { resize(); } array[size ] = element; } private void resize() { capacity *= 2; int[] newArray = new int[capacity]; System.arraycopy(array, 0, newArray, 0, size); array = newArray; } }
This code demonstrates the effort required to make arrays behave like lists, which can be error-prone and less efficient than using a built-in list structure.
On the flip side, lists can sometimes be less efficient for certain operations. For example, if you need to frequently access elements by index, a list might not be the best choice due to potential overhead in memory management. In a project where we needed to perform millions of index-based lookups, we switched from a list to an array and saw a significant performance boost.
In terms of best practices, when working with arrays, always ensure you're not exceeding the bounds, and consider using wrapper classes like ArrayList
in Java if you need dynamic resizing. For lists, be mindful of the operations you're performing and their time complexities. If you're frequently inserting or removing elements at the beginning of a list, consider using a data structure like a deque
instead.
To wrap up, while arrays and lists share some common operations, their fundamental differences mean that not all operations are supported by both. Arrays offer speed and efficiency for fixed-size collections, while lists provide flexibility and ease of use for dynamic collections. Understanding these trade-offs is crucial for choosing the right data structure for your specific needs.
So, the next time you're deciding between an array and a list, think about the operations you'll be performing most often and choose accordingly. Happy coding!
The above is the detailed content of Are all list operations supported by arrays, and vice versa? Why or why not?. For more information, please follow other related articles on the PHP Chinese website!
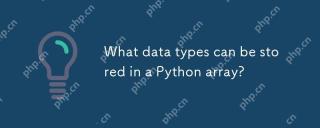
Pythonlistscanstoreanydatatype,arraymodulearraysstoreonetype,andNumPyarraysarefornumericalcomputations.1)Listsareversatilebutlessmemory-efficient.2)Arraymodulearraysarememory-efficientforhomogeneousdata.3)NumPyarraysareoptimizedforperformanceinscient
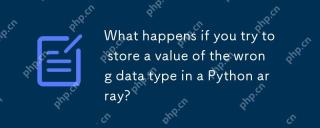
WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
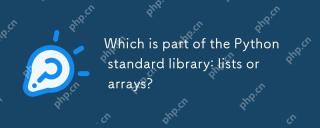
Pythonlistsarepartofthestandardlibrary,whilearraysarenot.Listsarebuilt-in,versatile,andusedforstoringcollections,whereasarraysareprovidedbythearraymoduleandlesscommonlyusedduetolimitedfunctionality.
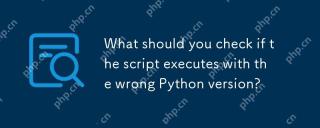
ThescriptisrunningwiththewrongPythonversionduetoincorrectdefaultinterpretersettings.Tofixthis:1)CheckthedefaultPythonversionusingpython--versionorpython3--version.2)Usevirtualenvironmentsbycreatingonewithpython3.9-mvenvmyenv,activatingit,andverifying
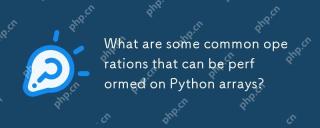
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
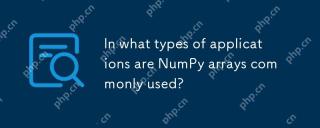
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
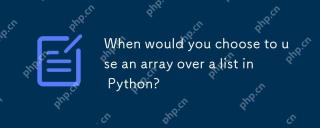
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf
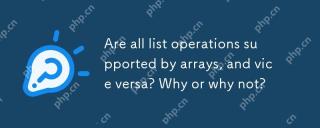
No,notalllistoperationsaresupportedbyarrays,andviceversa.1)Arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,whichimpactsperformance.2)Listsdonotguaranteeconstanttimecomplexityfordirectaccesslikearraysdo.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
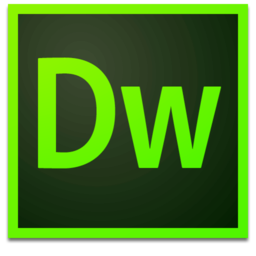
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
