How to use C++ to develop high-performance functions in embedded systems
How to use C to develop functions with high performance in embedded systems
Embedded systems are computer systems embedded in various devices and have specific functions . Since embedded systems often have limited resources and have high performance requirements, it is very important to choose a programming language suitable for developing embedded systems. C is a powerful and efficient programming language suitable for developing embedded system functions. In this article, we describe how to use C to develop high-performance functionality in embedded systems and provide some code examples.
- Use low-level operational control hardware
Embedded systems often require direct control of hardware, such as GPIO pins, analog input/output, and timers. In C, you can use low-level techniques such as bit operations, pointers, and memory mapping to achieve direct control of the hardware.
Sample Code 1: Control GPIO pins
// 假设引脚2用于控制LED灯 volatile unsigned int* gpio = (unsigned int*)0x12345678; // GPIO寄存器的基地址 // 设置引脚2为输出模式 *gpio &= ~(1 << 2); // 设置引脚2为高电平,LED灯亮 *gpio |= (1 << 2); // 设置引脚2为低电平,LED灯灭 *gpio &= ~(1 << 2);
- Use efficient algorithms and data structures
In embedded systems, resources are limited, so they need to be minimized Memory and processor usage. Using efficient algorithms and data structures improves performance and saves resources.
Sample code 2: use dynamic array to dynamically allocate memory
// 假设需要一个大小为50的整数数组 int* array = new int[50]; // 初始化数组 for (int i = 0; i < 50; ++i) { array[i] = i; } // 使用数组 for (int i = 0; i < 50; ++i) { // do something } // 释放内存 delete[] array;
- Avoid using dynamic memory allocation
In embedded systems, dynamic memory allocation (such as new and delete operator) can cause memory fragmentation and performance issues. To avoid these problems, dynamic memory allocation should be avoided whenever possible.
Sample Code 3: Use static arrays instead of dynamically allocated data structures
// 假设需要一个大小为50的整数数组 int array[50]; // 初始化数组 for (int i = 0; i < 50; ++i) { array[i] = i; } // 使用数组 for (int i = 0; i < 50; ++i) { // do something }
- Optimize for hardware characteristics
Embedded systems usually have specific hardware characteristics, Such as processor architecture and instruction set, etc. These hardware features can be optimized to improve performance.
Sample code 4: Vectorized calculations using SIMD instructions of the processor
#include <iostream> #include <immintrin.h> // SIMD指令集的头文件 void vector_add(const float* a, const float* b, float* result, int size) { __m128* va = (__m128*)a; __m128* vb = (__m128*)b; __m128* vr = (__m128*)result; int simd_size = size / 4; // 每次处理四个元素 for (int i = 0; i < simd_size; ++i) { vr[i] = _mm_add_ps(va[i], vb[i]); // 使用SIMD指令实现向量相加 } // 处理剩余的元素 for (int i = simd_size * 4; i < size; ++i) { result[i] = a[i] + b[i]; } } int main() { const int size = 16; float a[size] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16}; float b[size] = {1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1}; float result[size]; vector_add(a, b, result, size); for (int i = 0; i < size; ++i) { std::cout << result[i] << " "; } std::cout << std::endl; return 0; }
Summary:
When developing the functionality of an embedded system, it is very important to choose a suitable programming language. important. As an efficient and powerful programming language, C is well suited for developing embedded systems. High-performance embedded system functions can be achieved by using low-level operations to control the hardware, using efficient algorithms and data structures, avoiding the use of dynamic memory allocation, and optimizing for hardware characteristics.
The above is an introduction and code examples on how to use C to develop functions with high performance in embedded systems. Hope this helps!
The above is the detailed content of How to use C++ to develop high-performance functions in embedded systems. For more information, please follow other related articles on the PHP Chinese website!
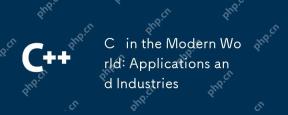
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
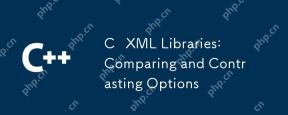
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
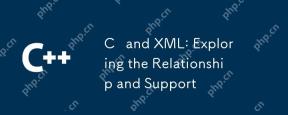
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
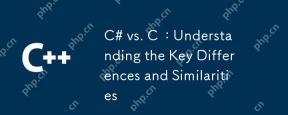
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
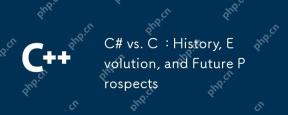
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
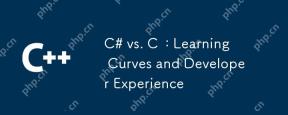
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
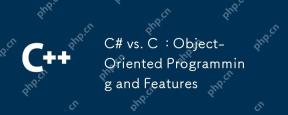
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
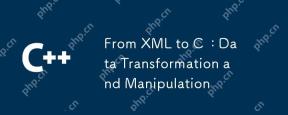
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
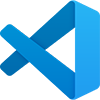
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!