Title: PHP and OAuth: Implementing Slack login integration
In current web application development, many applications need to integrate third-party login functionality. The OAuth protocol has become one of the most commonly used implementation methods. This article will introduce how to use PHP and OAuth to implement Slack login integration.
1. Preparation
Before we start, we need to do some preparations. First, we need a Slack developer account, which can be registered on the official Slack developer website. After registration is complete, we need to create a new application. On the application settings page, we can find two important credentials, Client ID and Client Secret.
2. Install the OAuth library
In PHP, there are many ready-made OAuth libraries that can be used. Here we use "thephpleague/oauth2-client" as an example to explain. You can use Composer to install:
composer require league/oauth2-client
3. Write OAuth login code
The following is a simple PHP code example that demonstrates how to use the OAuth library for Slack login integration.
<?php require_once 'vendor/autoload.php'; use LeagueOAuth2ClientProviderGenericProvider; $provider = new GenericProvider([ 'clientId' => '<your-client-id>', 'clientSecret' => '<your-client-secret>', 'redirectUri' => 'http://your-website.com/callback.php', 'urlAuthorize' => 'https://slack.com/oauth/v2/authorize', 'urlAccessToken' => 'https://slack.com/api/oauth.v2.access', 'urlResourceOwnerDetails' => '', ]); // Step 1: Redirect the user to the authorization URL $authUrl = $provider->getAuthorizationUrl(); $_SESSION['oauth2state'] = $provider->getState(); header('Location: ' . $authUrl); exit;
In the above code, we first introduced the OAuth library and created a GenericProvider instance. When creating an instance, we need to fill in information such as Client ID and Client Secret.
In line 20 of the code, we call the getAuthorizationUrl()
method to get the authorization URL and redirect the user to that URL. After the user logs in at this URL and agrees to the authorization, he will be redirected back to our preset callback URL.
4. Write the callback code
After the user is authorized, Slack will redirect the user to our pre-set callback URL and bring the authorization code (code). We need to write the code for the callback page to process this authorization code and obtain the access token.
<?php require_once 'vendor/autoload.php'; use LeagueOAuth2ClientProviderGenericProvider; $provider = new GenericProvider([ 'clientId' => '<your-client-id>', 'clientSecret' => '<your-client-secret>', 'redirectUri' => 'http://your-website.com/callback.php', 'urlAuthorize' => 'https://slack.com/oauth/v2/authorize', 'urlAccessToken' => 'https://slack.com/api/oauth.v2.access', 'urlResourceOwnerDetails' => '', ]); // Step 2: Get an access token if (!isset($_GET['code'])) { exit('Error: Missing authorization code'); } $accessToken = $provider->getAccessToken('authorization_code', [ 'code' => $_GET['code'] ]); // Step 3: Use the access token to access the API $response = $provider->getAuthenticatedRequest( 'GET', 'https://slack.com/api/users.identity', $accessToken ); $userInfo = $provider->getParsedResponse($response); // Here you can handle the user information returned by Slack var_dump($userInfo);
In the above code, we first introduced the OAuth library and created a GenericProvider instance. As before, we need to fill in information such as Client ID and Client Secret.
In line 14 of the code, we pass in the authorization code (code) to obtain the access token through the getAccessToken()
method.
In line 17 of the code, we use the obtained access token to call Slack’s API to obtain the user’s identity information.
Finally, you can implement your own business logic based on user information.
5. Summary
Through the above steps, we successfully implemented Slack login integration using PHP and OAuth. The OAuth protocol provides us with a safe and reliable method to integrate third-party login functions, making the user's login experience more convenient. Hope this article can be helpful to you.
The above is the detailed content of PHP and OAuth: Implementing Slack login integration. For more information, please follow other related articles on the PHP Chinese website!
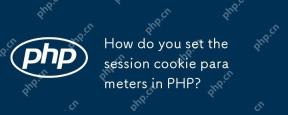
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
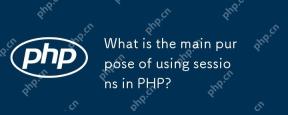
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
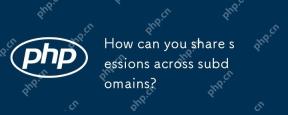
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
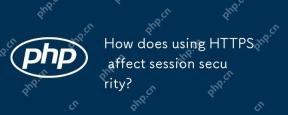
HTTPS significantly improves the security of sessions by encrypting data transmission, preventing man-in-the-middle attacks and providing authentication. 1) Encrypted data transmission: HTTPS uses SSL/TLS protocol to encrypt data to ensure that the data is not stolen or tampered during transmission. 2) Prevent man-in-the-middle attacks: Through the SSL/TLS handshake process, the client verifies the server certificate to ensure the connection legitimacy. 3) Provide authentication: HTTPS ensures that the connection is a legitimate server and protects data integrity and confidentiality.
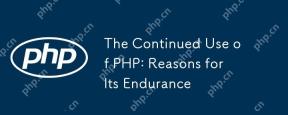
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
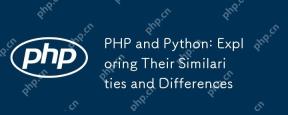
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
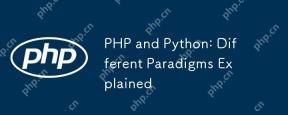
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
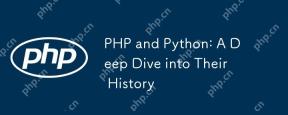
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
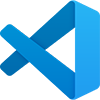
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor