How to use the caching function in the Yii framework
Caching is a common performance optimization method that can significantly improve the response speed of a website or application. The Yii framework provides powerful caching functions that can help us simplify the cache use and management process. This article will introduce how to use the caching function in the Yii framework and provide some code examples.
1. Caching Component in the Yii framework
The caching function in the Yii framework is implemented by a component called Caching Component. This component provides a series of methods for reading, setting, and deleting cached data. In the Yii framework, the caching component is enabled by default, but we can configure and extend it according to our needs.
2. Cache Backend in Yii framework
The cache component implements specific cache storage and reading operations through cache memory (Cache Backend). The Yii framework provides a variety of cache storage implementations, including memory, files, databases, etc. We can choose the appropriate memory according to our needs, or customize the memory.
3. Basic caching operations
The following are some basic operations for using the caching function in the Yii framework:
- Set the cache value
Yii::app() ->cache->set('key', 'value', $duration);
Where, 'key' is the unique identifier of the cache item, and 'value' is the one to be cached data, $duration is the duration of the cache in seconds. If the $duration parameter is set to 0, the cache is permanently valid.
- Get cache value
$value = Yii::app()->cache->get('key');
Through this operation , we can get the value corresponding to the cache item 'key'.
- Delete cache value
Yii::app()->cache->delete('key');
This operation can delete the specified Cache item.
4. Example of using cache
The following is an example of using the cache function. We will read the data in the database and cache it:
public function getBooks() { $key = 'books'; $books = Yii::app()->cache->get($key); if ($books === false) { // 如果缓存中不存在数据,则从数据库中获取数据 $books = Book::model()->findAll(); // 缓存数据,有效期为5分钟 Yii::app()->cache->set($key, $books, 300); } return $books; }
In the above example, we First try to get the book data from the cache. If the data does not exist in the cache (that is, the return value is false), the data is obtained from the database. Then, cache the obtained data and set the validity period to 5 minutes.
Through the above examples, we can see that using the cache function can significantly improve performance and reduce the number of accesses to the database.
5. Custom cache memory
The Yii framework also allows us to customize the cache memory to meet specific needs. The following is an example of a custom cache memory:
class MyCache extends CDbCache { public function init() { parent::init(); // 在这里可以进行自定义的初始化操作 } protected function getValue($key) { // 在这里可以实现自定义的读取操作 } protected function setValue($key, $value, $expire) { // 在这里可以实现自定义的存储操作 } protected function deleteValue($key) { // 在这里可以实现自定义的删除操作 } }
In the above example, we inherited the CDbCache class provided by the Yii framework and implemented the three methods getValue, setValue and deleteValue. By overriding these methods, we can implement custom read, store, and delete operations.
6. Summary
Caching is one of the important means to improve the performance of a website or application. In the Yii framework, using the caching function is very simple. By using the cache components and cache memory provided by the Yii framework, we can easily manage and operate caches. By using caching properly, we can significantly improve application performance and reduce the number of accesses to resources such as databases.
The above is an introduction and sample code about using the cache function in the Yii framework. Hope it helps.
The above is the detailed content of How to use caching function in Yii framework. For more information, please follow other related articles on the PHP Chinese website!
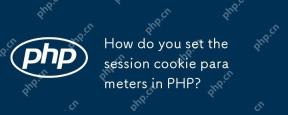
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
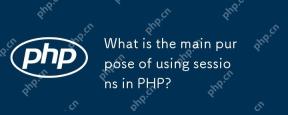
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
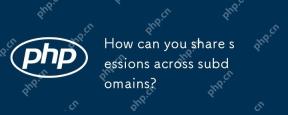
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
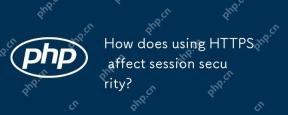
HTTPS significantly improves the security of sessions by encrypting data transmission, preventing man-in-the-middle attacks and providing authentication. 1) Encrypted data transmission: HTTPS uses SSL/TLS protocol to encrypt data to ensure that the data is not stolen or tampered during transmission. 2) Prevent man-in-the-middle attacks: Through the SSL/TLS handshake process, the client verifies the server certificate to ensure the connection legitimacy. 3) Provide authentication: HTTPS ensures that the connection is a legitimate server and protects data integrity and confidentiality.
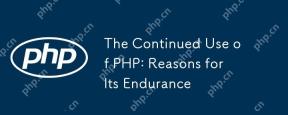
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
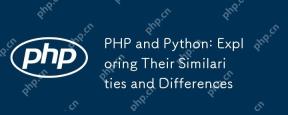
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
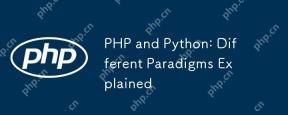
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
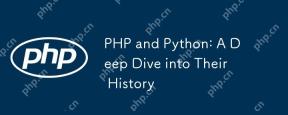
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.