


How to use the json module in Python 3.x to convert JSON strings into Python objects
JSON (JavaScript Object Notation) is a lightweight data exchange format commonly used for front-end and back-end data transmission and storage. In Python, you can use the json module to process JSON data. The json module provides a simple set of functions and methods for converting JSON strings into Python objects. This article will introduce how to use the json module to parse and convert JSON strings.
First, we need to import the json module.
import json
Next, we need a JSON string, which can be represented by single quotes or double quotes. Here is a sample JSON string:
json_str = '{"name": "John", "age": 30, "city": "New York"}'
With the JSON string, we can use the loads function in the json module to parse it into a Python object. The loads function parses the JSON string into a dictionary object.
data = json.loads(json_str)
Now, the data object is a Python dictionary, and we can access its values through keys.
print(data['name']) # 输出: John print(data['age']) # 输出: 30 print(data['city']) # 输出: New York
In addition to dictionary objects, JSON strings can also be parsed into other Python data types, such as lists. Here is a sample JSON string:
json_str = '["apple", "banana", "orange"]'
We can use the loads function to parse it into a Python list.
data = json.loads(json_str)
Now, the data object is a Python list, and we can use subscripts to access its elements.
print(data[0]) # 输出: apple print(data[1]) # 输出: banana print(data[2]) # 输出: orange
At the same time, the json module also provides the dumps function, which can convert Python objects into JSON strings. Here is an example:
data = { 'name': 'John', 'age': 30, 'city': 'New York' } json_str = json.dumps(data) print(json_str) # 输出: {"name": "John", "age": 30, "city": "New York"}
In addition to dictionaries and lists, the json module can also handle other data types, such as strings, integers, floating point numbers, and Boolean values.
json_str = 'true' data = json.loads(json_str) print(data) # 输出: True json_str = '42' data = json.loads(json_str) print(data) # 输出: 42 json_str = '3.14' data = json.loads(json_str) print(data) # 输出: 3.14 json_str = '"Hello, World!"' data = json.loads(json_str) print(data) # 输出: Hello, World!
It should be noted that the JSON string must conform to the JSON format specification, otherwise an error will occur in parsing. For example, key names and string values in JSON strings must use double quotes, and single quotes cannot be used.
When processing JSON data, we can also use some parameters to perform customized operations. These parameters include: indent, sort_keys, ensure_ascii, etc. For specific usage, please refer to the documentation of the json module.
To summarize, using the json module can easily convert JSON strings into Python objects for operation and processing. In Python 3.x, the loads function provided by the json module can parse JSON strings into Python objects, and the dumps function can convert Python objects into JSON strings. This allows us to easily process JSON data in Python.
I hope that through the introduction of this article, readers will have an understanding of how to use the json module to convert JSON strings, so that they can better process and utilize JSON data in actual development.
The above is the detailed content of How to use the json module to convert JSON strings into Python objects in Python 3.x. For more information, please follow other related articles on the PHP Chinese website!
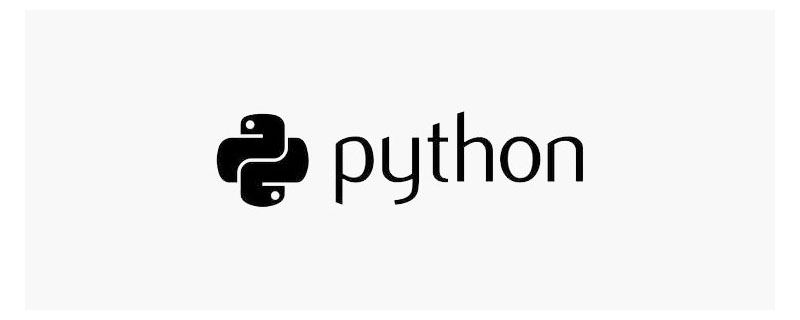
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
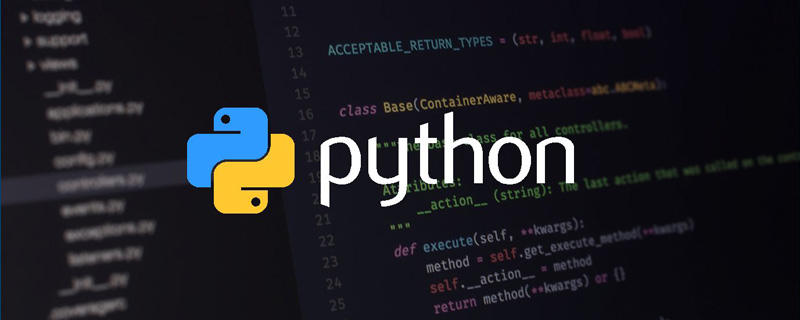
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
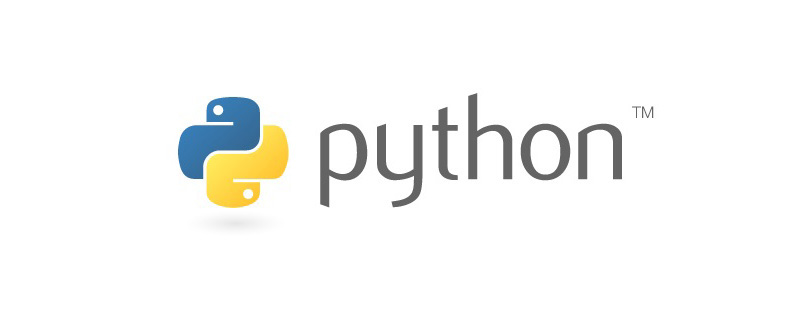
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
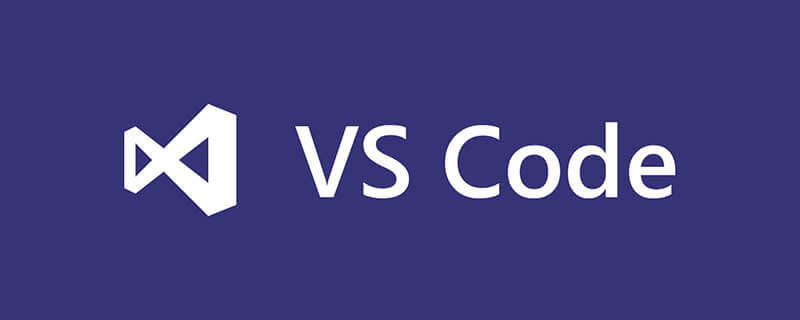
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
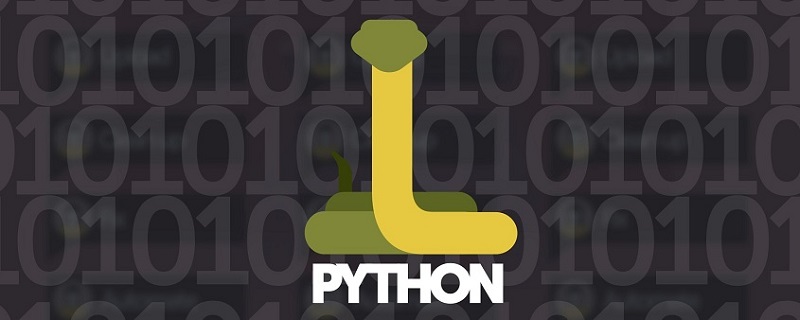
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
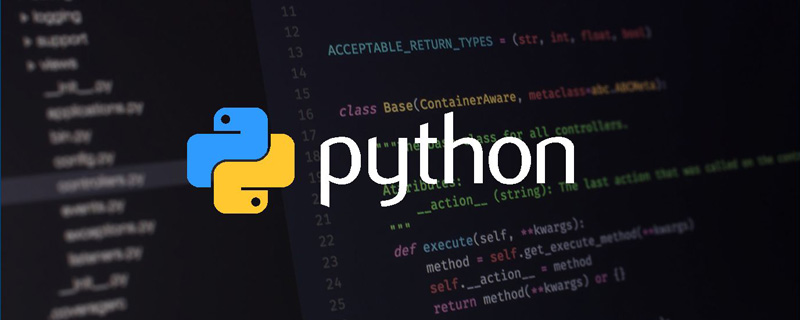
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
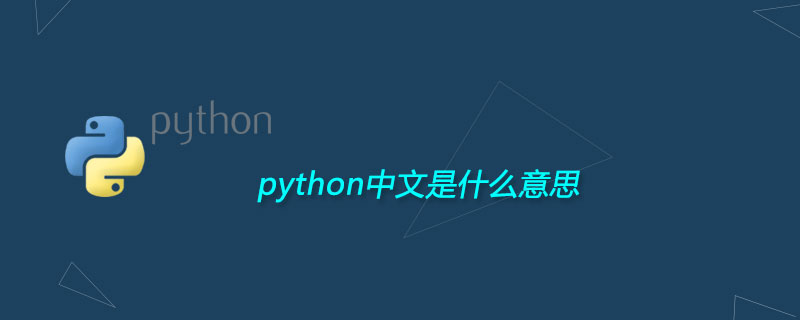
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
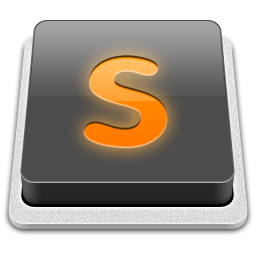
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
