


How to use Mozilla Firefox in Scrapy to solve the problem of scanning QR code to log in?
For crawlers to crawl websites that require login, verification code or scan code login is a very troublesome problem. Scrapy is a very easy-to-use crawler framework in Python, but when processing verification codes or scanning QR codes to log in, some special measures need to be taken. As a common browser, Mozilla Firefox provides a solution that can help us solve this problem.
The core module of Scrapy is twisted, which only supports asynchronous requests, but some websites need to use cookies and sessions to stay logged in, so we need to use Mozilla Firefox to handle these problems.
First, we need to install the Mozilla Firefox browser and the corresponding Firefox driver in order to use it in Python. The installation command is as follows:
pip install selenium
Then, we need to add some settings to the crawler's settings.py file in order to use the Firefox browser to scan the QR code to log in. The following is a sample setting:
DOWNLOADER_MIDDLEWARES = { 'scrapy.downloadermiddlewares.cookies.CookiesMiddleware':700, 'scrapy_selenium.SeleniumMiddleware':800, } SELENIUM_DRIVER_NAME = 'firefox' SELENIUM_DRIVER_EXECUTABLE_PATH = which('geckodriver') SELENIUM_BROWSER_EXECUTABLE_PATH = '/usr/bin/firefox'
We can set it according to our own operating system and Firefox installation path.
Next, we need to create a custom Scrapy Spider class to use the Firefox browser in it. In this class, we need to set some options for the Firefox browser, as shown below:
from selenium import webdriver from scrapy.selector import Selector from scrapy.spiders import CrawlSpider from scrapy.http import Request class MySpider(CrawlSpider): name = 'myspider' def __init__(self): self.driver = webdriver.Firefox(executable_path='geckodriver', firefox_binary='/usr/bin/firefox') self.driver.set_window_size(1400, 700) self.driver.set_page_load_timeout(30) self.driver.set_script_timeout(30) def parse(self, response): # 网站首页处理代码 pass
In this custom Spider class, we use the selenium.webdriver.Firefox class to create a Firefox browser control device object. The Firefox browser controller object is used to open the home page of the website and can also perform other operations as needed.
For websites that require scanning QR codes to log in, we can use the Firefox browser to identify the QR code on the page and wait for the scanning result of the QR code. We can use Selenium to simulate user behavior in Python to scan the QR code and log in to the website. The complete code scanning login code is as follows:
def parse(self, response): self.driver.get(response.url) # 等待页面加载完成 time.sleep(5) # 寻找二维码及其位置 frame = self.driver.find_element_by_xpath('//*[@class="login-qr-code iframe-wrap"]//iframe') self.driver.switch_to.frame(frame) qr_code = self.driver.find_element_by_xpath('//*[@id="login-qr-code"]/img') position = qr_code.location size = qr_code.size while True: # 判断是否已经扫描了二维码, # 如果扫描了,登录,并跳出循环 try: result = self.driver.find_element_by_xpath('//*[@class="login-qr-code-close"]') result.click() break except: pass # 如果没有扫描,等待并继续寻找 time.sleep(5) # 登录后处理的代码 pass
In the above code, we first use the self.driver.get() method to open the homepage of the website, and then use the find_element_by_xpath() method to find the QR code element. Get its position and size. Then use a while loop to wait for the QR code scanning result. If it has been scanned, click the close button on the QR code and jump out of the loop. If there is no scan, wait 5 seconds and continue searching.
When the QR code scanning results are available, we can execute our own login logic. The specific processing method depends on the actual situation of the website.
In short, when using Scrapy for crawler development, if we encounter a website that requires login, and the website uses a verification code or scan code to log in, we can use the above method to solve this problem. Using Selenium and Firefox browsers, we can simulate user operations, handle QR code login issues, and obtain the required data.
The above is the detailed content of How to use Mozilla Firefox in Scrapy to solve the problem of scanning QR code to log in?. For more information, please follow other related articles on the PHP Chinese website!
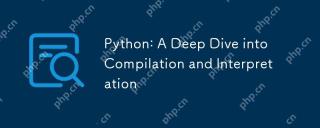
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
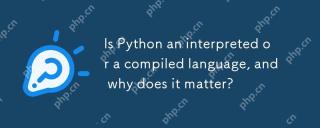
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
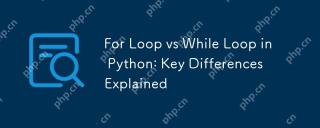
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
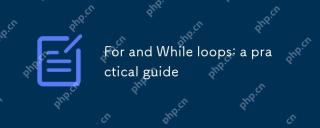
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond
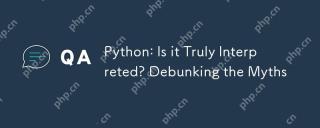
Pythonisnotpurelyinterpreted;itusesahybridapproachofbytecodecompilationandruntimeinterpretation.1)Pythoncompilessourcecodeintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).2)Thisprocessallowsforrapiddevelopmentbutcanimpactperformance,req
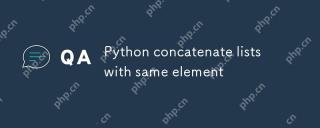
ToconcatenatelistsinPythonwiththesameelements,use:1)the operatortokeepduplicates,2)asettoremoveduplicates,or3)listcomprehensionforcontroloverduplicates,eachmethodhasdifferentperformanceandorderimplications.
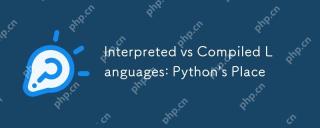
Pythonisaninterpretedlanguage,offeringeaseofuseandflexibilitybutfacingperformancelimitationsincriticalapplications.1)InterpretedlanguageslikePythonexecuteline-by-line,allowingimmediatefeedbackandrapidprototyping.2)CompiledlanguageslikeC/C transformt
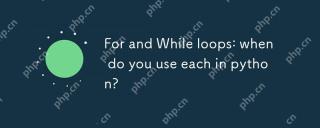
Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
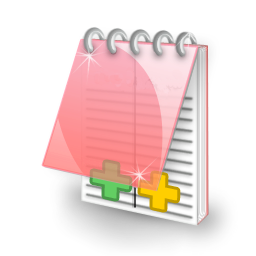
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
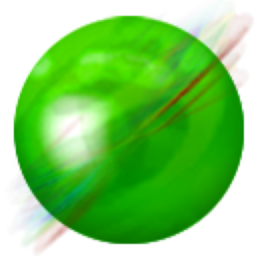
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
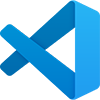
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
