


How to use caching to improve the performance of smart medical algorithms in Golang?
With the development of artificial intelligence technology, more and more intelligent algorithms are used in the medical field. However, in practical applications, we often find that performance has become a bottleneck restricting the application of intelligent medical algorithms. In this case, how to use caching to improve performance becomes particularly important.
Golang is an increasingly popular language, and its excellent concurrency performance makes it a good choice for implementing high-concurrency algorithms. In Golang, we can use caching to avoid repeated calculations and improve algorithm performance. Next, we will discuss how to use caching to improve the performance of smart medical algorithms based on specific application scenarios of smart medical algorithms.
1. Medical image processing
Medical image processing is an important application in the field of intelligent medical care and involves relatively complex image processing algorithms. For example, in the process of CT image processing, we often need to calculate the gray value of each pixel. However, in the same image processing, the same pixels are often repeatedly calculated multiple times, which will undoubtedly have a greater impact on the performance of the algorithm.
In order to avoid repeated calculations, we can use cache to store already calculated pixel information. In Golang, the Map data structure can be used to implement the cache pool:
type pixel struct { x int y int } type cache struct { m map[pixel]int sync.Mutex }
By defining two structures, pixel and cache, we can use pixel as the key to cache the calculated pixel information in the map. In addition, in order to ensure cache concurrency safety, we also need to use sync.Mutex.
Next, we can define a function to calculate the gray value of the pixel:
func computeGrayValue(x, y, width, height int, data []byte, c *cache) int { p := pixel{x, y} c.Mutex.Lock() if value, exists := c.m[p]; exists { c.Mutex.Unlock() return value } c.Mutex.Unlock() // 使用data对像素点进行计算 // ... // 将计算结果存入缓存中 c.Mutex.Lock() c.m[p] = value c.Mutex.Unlock() return value }
During the calculation process, we first try to obtain the pixel information from the cache. If the calculation result already exists, The result is returned directly. After the calculation is completed, we store the calculation results in the cache for subsequent use. In this way, we can avoid repeated calculations and improve the performance of the algorithm.
2. Disease diagnosis
Disease diagnosis is another important application in intelligent medical care, which often involves complex logical judgments and pattern matching algorithms. For example, for a patient's symptoms, we need to make judgments based on existing diagnostic rules and case databases to obtain possible disease diagnosis results.
In such a scenario, the use of cache is also necessary. We can cache already matched rules and case information to avoid repeated matching. In Golang, you can use map and struct respectively to implement the cache pool:
type record struct { rule string value string } type cache struct { rule map[string]bool rec map[string]record sync.Mutex }
By defining the record structure and cache structure, we can store the matched rules and case information in the cache. At the same time, in order to ensure cache concurrency safety, we also need to use sync.Mutex.
Next, we can define a function for matching rules:
func matchRule(rule string, c *cache) bool { c.Mutex.Lock() if exists := c.rule[rule]; exists { c.Mutex.Unlock() return true } c.Mutex.Unlock() // 匹配规则 // ... c.Mutex.Lock() c.rule[rule] = true c.Mutex.Unlock() return false }
During the matching process, we first try to obtain the matching results from the cache. If a matching result already exists, the result will be returned directly. After the matching is completed, we store the matching results in the cache for subsequent use.
In this way, we can effectively utilize the caching mechanism to avoid repeated calculations and matching, and improve the performance of intelligent medical algorithms.
Summary
In the practical application of intelligent medical algorithms, optimizing performance is a very important task. In Golang, by using the caching mechanism, we can avoid repeated calculations and matching and improve algorithm performance. In addition, Golang's excellent concurrency performance also provides good support for the implementation of high-concurrency intelligent medical algorithms.
The above is the detailed content of How to use caching to improve the performance of smart medical algorithms in Golang?. For more information, please follow other related articles on the PHP Chinese website!
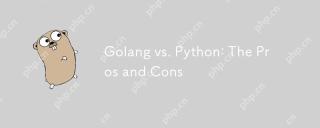
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
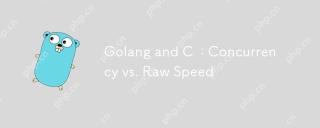
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
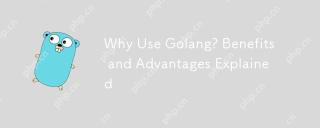
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
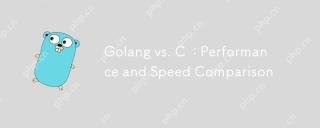
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
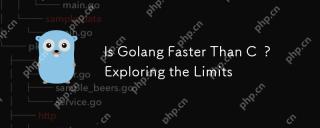
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
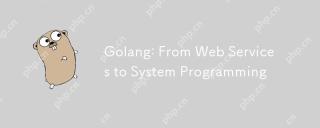
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
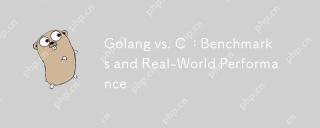
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
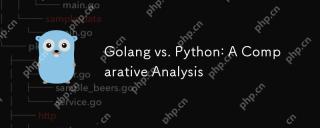
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
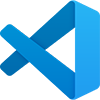
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
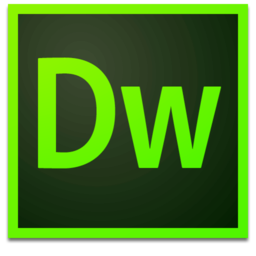
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software