Golang is an efficient, reliable and easy-to-use programming language that is often used in network servers, distributed systems, cloud computing and other fields. When writing Golang applications, you often need to operate variables: create, modify, use, etc. Among them, deleting variables is also a very common operation. This article will explain in detail how to delete variables in Golang.
1. What is a variable?
Variables are the basic units for storing, representing and processing data in computer programs. In Golang, variables consist of three parts: variable name, variable type and value. For example, the following code defines an integer variable named "num" and assigns it a value of 10:
var num int = 10
Variables can be used to save the state of the program, transfer data, perform calculations, etc. When using variables, you often need to operate on them. Among them, deleting variables is also a relatively common operation.
2. How to delete variables in Golang
The method of deleting variables in Golang is very simple, just use the delete
statement. The syntax of the delete
statement is as follows:
delete(map, key)
Among them, map
is the mapping table that needs to be deleted, and key
is the key that needs to be deleted. The following code is an example to demonstrate the deletion of key-value pairs in the map:
func main() { m := make(map[string]int) m["a"] = 1 m["b"] = 2 fmt.Println(m) //输出:map[a:1 b:2] delete(m, "a") fmt.Println(m) //输出:map[b:2] }
The above code first defines a variable m
of type map
, and adds it to it There are two key-value pairs. Then, use the delete
statement to delete the key-value pair with key "a". Finally, output the value of the map
type variable. You can see that the key-value pair with the key "a" has been deleted.
In addition to deleting map
type variables, Golang can also delete slice
, array
and struct
types. elements in the variable. The following is a sample code to delete elements in slice
and array
type variables:
func main() { a := []int{1, 2, 3} fmt.Println(a) //输出:[1 2 3] a = append(a[:1], a[2:]...) fmt.Println(a) //输出:[1 3] b := [3]int{1, 2, 3} fmt.Println(b) //输出:[1 2 3] c := append(b[:1], b[2:]...) fmt.Println(c) //输出:[1 3] }
The above code first defines a slice
type variablea
and a array
type variableb
, and some elements are passed to it. Then, the append
function of slice
and the slicing operation are used to delete the elements in the slice
and array
type variables. Finally, output the value of the variable and you can see that the element has been successfully deleted.
3. Notes
When performing variable deletion operations, you need to pay attention to the following points:
- Only
map
type variables can be useddelete
statement,slice
,array
andstruct
type variables need to use other methods to delete elements. - Deleting keys that do not exist in
map
type variables will not cause any errors or exceptions. - When deleting elements in
slice
,array
andstruct
type variables, you need to take into account the change in the order of the elements to avoid unexpected results.
4. Summary
This article introduces the method of deleting variables in Golang. We can easily delete elements in variables through delete
statements and slicing operations. When performing deletion operations, paying attention to some details can effectively avoid abnormal situations in the program. Hope this article can help you solve problems in Golang programming.
The above is the detailed content of golang delete variable. For more information, please follow other related articles on the PHP Chinese website!
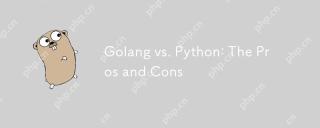
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
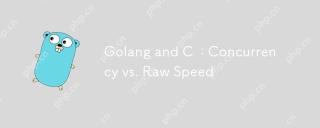
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
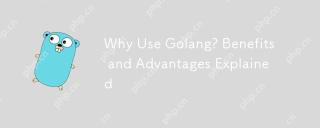
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
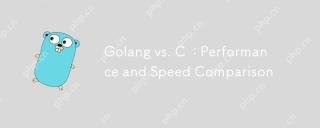
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
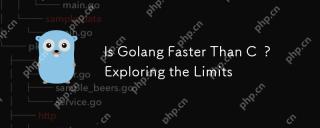
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
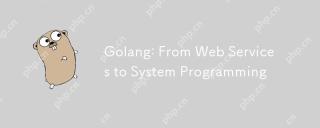
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
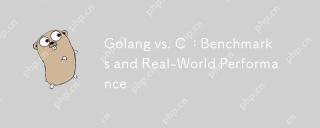
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
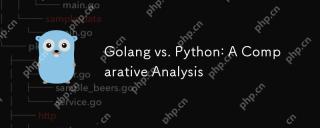
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
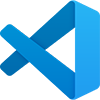
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
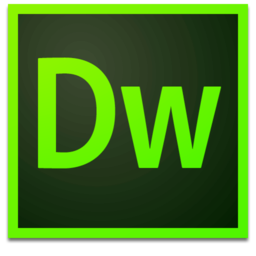
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software