JavaScript is a widely used scripting language used to achieve interactive and dynamic effects on web pages. In this language, we can easily draw various shapes and figures, including right triangles, with just a few lines of code.
The right triangle is a very basic geometric figure, consisting of a right angle and two acute angles. In this article, we will explore how to use JavaScript to write a program that can draw a right triangle.
First, let's learn how to use HTML and CSS to create a Canvas element, which is the container required for drawing.
HTML example:
<canvas id="myCanvas" width="500" height="500"></canvas>
CSS example:
canvas { border: 1px solid black; }
The above HTML code creates a Canvas element with a black border and sets its width and height to 500 pixels. Next, we need to prepare some JavaScript code to draw a right triangle in this Canvas element.
First, we need to get this Canvas element in JavaScript:
var canvas = document.getElementById("myCanvas");
Next, we need to get the context object of the Canvas element, which contains a series of graphics used to draw graphics. Method:
var ctx = canvas.getContext("2d");
Now, we can use the method on the ctx object to start drawing a right triangle.
ctx.beginPath(); // 开始路径 ctx.moveTo(100, 100); // 移动到起始位置 ctx.lineTo(100, 300); // 绘制垂直边 ctx.lineTo(300, 300); // 绘制水平边 ctx.closePath(); // 结束路径 ctx.stroke(); // 绘制轮廓线
In the above code, we start a new path by calling the ctx.beginPath()
method, and then use the ctx.moveTo()
method to move the path to the starting point Position (100,100), then use the ctx.lineTo()
method to draw the two right-angled sides of the right triangle respectively, and finally call the ctx.closePath()
method to end the path, and then use ctx.stroke()
The method draws the outline of the path.
With the above code, we successfully drew a right triangle in the Canvas element. However, such drawing code is inconvenient when multiple right triangles need to be drawn. Therefore, we can encapsulate them into a function to call directly when needed.
function drawRightTriangle(x, y, width, height) { ctx.beginPath(); ctx.moveTo(x, y); ctx.lineTo(x, y + height); ctx.lineTo(x + width, y + height); ctx.closePath(); ctx.stroke(); }
In the above code, we define a function named drawRightTriangle
, which accepts four parameters: x and y are the starting coordinates of the right triangle, width and height are the right angles The width and height of the triangle. This function is basically the same as the previous code, except that the code for drawing a right triangle is encapsulated into a function form. When calling this function, you only need to pass in the corresponding parameters to draw a right triangle with the corresponding position and size.
drawRightTriangle(50, 50, 100, 200);
Through the above method, we can easily write a program in JavaScript that can draw a right triangle, and realize the drawing of a right triangle with customizable position and size.
The above is the detailed content of How to write a right triangle in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

ChooseuseState()forsimple,independentstatevariables;useuseReducer()forcomplexstatelogicorwhenstatedependsonpreviousstate.1)useState()isidealforsimpleupdatesliketogglingabooleanorupdatingacounter.2)useReducer()isbetterformanagingmultiplesub-valuesorac

useState is superior to class components and other state management solutions because it simplifies state management, makes the code clearer, more readable, and is consistent with React's declarative nature. 1) useState allows the state variable to be declared directly in the function component, 2) it remembers the state during re-rendering through the hook mechanism, 3) use useState to utilize React optimizations such as memorization to improve performance, 4) But it should be noted that it can only be called on the top level of the component or in custom hooks, avoiding use in loops, conditions or nested functions.

UseuseState()forlocalcomponentstatemanagement;consideralternativesforglobalstate,complexlogic,orperformanceissues.1)useState()isidealforsimple,localstate.2)UseglobalstatesolutionslikeReduxorContextforsharedstate.3)OptforReduxToolkitorMobXforcomplexst

ReusablecomponentsinReactenhancecodemaintainabilityandefficiencybyallowingdeveloperstousethesamecomponentacrossdifferentpartsofanapplicationorprojects.1)Theyreduceredundancyandsimplifyupdates.2)Theyensureconsistencyinuserexperience.3)Theyrequireoptim

TheVirtualDOMisalightweightin-memorycopyoftherealDOMusedbyReacttooptimizeUIupdates.ItboostsperformancebyminimizingdirectDOMmanipulationthroughaprocessofupdatingtheVirtualDOMfirst,thenapplyingonlynecessarychangestotheactualDOM.

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
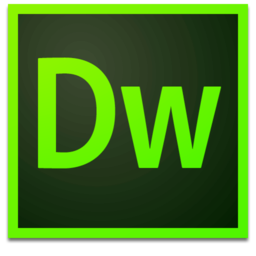
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
