


React's Reusable Components: Enhancing Code Maintainability and Efficiency
Reusable components in React enhance code maintainability and efficiency by allowing developers to use the same component across different parts of an application or projects. 1) They reduce redundancy and simplify updates. 2) They ensure consistency in user experience. 3) They require optimization to prevent unnecessary re-renders, using tools like memo and useCallback. 4) Best practices include keeping components focused and using type safety tools like prop-types or TypeScript.
When it comes to React, the concept of reusable components is not just a feature—it's a philosophy that drives the framework's design. Reusable components in React are all about enhancing code maintainability and efficiency. But why is this so important, and how can we truly leverage this concept to its fullest potential?
Let's dive into the world of React's reusable components and explore how they can transform the way we build applications.
Reusable components in React are essentially building blocks that can be used across different parts of an application or even across different projects. This approach not only reduces redundancy but also makes it easier to manage and update code. Imagine having a single component for a button that you can use throughout your app, and if you need to change its style or functionality, you only need to update it in one place.
Here's a simple example of a reusable button component:
import React from 'react'; const Button = ({ onClick, children }) => { return ( <button onClick={onClick} style={{ padding: '10px 20px', fontSize: '16px', cursor: 'pointer' }}> {children} </button> ); }; export default Button;
This component can be used like this:
import React from 'react'; import Button from './Button'; const App = () => { return ( <div> <Button onClick={() => alert('Clicked!')}>Click me</Button> <Button onClick={() => alert('Submit!')}>Submit</Button> </div> ); }; export default App;
The beauty of this approach lies in its simplicity and power. By creating components that are modular and reusable, we can build complex UIs with ease. But there's more to it than just writing less code.
One of the key advantages of reusable components is the ability to maintain consistency across an application. When you use the same component for similar functionalities, you ensure that the user experience remains uniform. This is particularly important for larger applications where maintaining a consistent look and feel can be challenging.
However, creating reusable components isn't without its challenges. One common pitfall is overgeneralization. It's tempting to create a component that tries to do too much, which can lead to bloated, hard-to-maintain code. The key is to strike a balance between reusability and specificity. A good rule of thumb is to start with a specific use case and then gradually abstract it into a more general component if needed.
Another aspect to consider is the performance impact of reusable components. While they can reduce the overall size of your codebase, they can also introduce unnecessary re-renders if not optimized properly. React's memo
and useCallback
hooks can be invaluable here. Here's an example of how to optimize a component:
import React, { memo, useCallback } from 'react'; const OptimizedButton = memo(({ onClick, children }) => { const handleClick = useCallback(() => { onClick(); }, [onClick]); return ( <button onClick={handleClick} style={{ padding: '10px 20px', fontSize: '16px', cursor: 'pointer' }}> {children} </button> ); }); export default OptimizedButton;
This optimized version of the button component uses memo
to prevent unnecessary re-renders and useCallback
to memoize the onClick
handler, which can be particularly useful if the parent component re-renders frequently.
In terms of best practices, it's crucial to keep your components focused and single-purpose. A component should ideally do one thing well. This not only makes your components more reusable but also easier to test and maintain. Additionally, consider using prop-types or TypeScript to enforce type safety, which can catch errors early in the development process.
From my experience, one of the most rewarding aspects of working with reusable components is the sense of accomplishment when you see your components being used across different parts of an application or even in different projects. It's like building a library of your own, where each component is a testament to your understanding of React and its ecosystem.
In conclusion, reusable components in React are a powerful tool for enhancing code maintainability and efficiency. By understanding their benefits and challenges, and by following best practices, you can leverage them to build more robust, scalable, and maintainable applications. Remember, the key is to keep your components focused, optimized, and well-documented, and you'll be well on your way to mastering the art of reusable components in React.
The above is the detailed content of React's Reusable Components: Enhancing Code Maintainability and Efficiency. For more information, please follow other related articles on the PHP Chinese website!

ChooseuseState()forsimple,independentstatevariables;useuseReducer()forcomplexstatelogicorwhenstatedependsonpreviousstate.1)useState()isidealforsimpleupdatesliketogglingabooleanorupdatingacounter.2)useReducer()isbetterformanagingmultiplesub-valuesorac

useState is superior to class components and other state management solutions because it simplifies state management, makes the code clearer, more readable, and is consistent with React's declarative nature. 1) useState allows the state variable to be declared directly in the function component, 2) it remembers the state during re-rendering through the hook mechanism, 3) use useState to utilize React optimizations such as memorization to improve performance, 4) But it should be noted that it can only be called on the top level of the component or in custom hooks, avoiding use in loops, conditions or nested functions.

UseuseState()forlocalcomponentstatemanagement;consideralternativesforglobalstate,complexlogic,orperformanceissues.1)useState()isidealforsimple,localstate.2)UseglobalstatesolutionslikeReduxorContextforsharedstate.3)OptforReduxToolkitorMobXforcomplexst

ReusablecomponentsinReactenhancecodemaintainabilityandefficiencybyallowingdeveloperstousethesamecomponentacrossdifferentpartsofanapplicationorprojects.1)Theyreduceredundancyandsimplifyupdates.2)Theyensureconsistencyinuserexperience.3)Theyrequireoptim

TheVirtualDOMisalightweightin-memorycopyoftherealDOMusedbyReacttooptimizeUIupdates.ItboostsperformancebyminimizingdirectDOMmanipulationthroughaprocessofupdatingtheVirtualDOMfirst,thenapplyingonlynecessarychangestotheactualDOM.

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
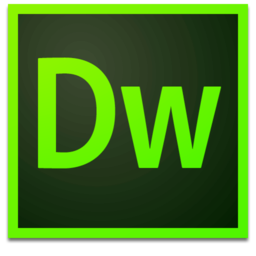
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
