JavaScript is a widely used scripting language that is important when creating interactive web sites and web applications. In JavaScript, we often need to deal with dates and times. JavaScript provides some built-in functions and objects to handle dates and times. This article will explain how to convert JavaScript to a date object.
1. Date object in JavaScript
The date object in JavaScript allows us to represent dates in a standard format (year/month/day). We can create dates using the built-in Date object. Here is a simple example:
var today = new Date(); console.log(today);
Executing this code will output the current date and time, for example:
Tue Sep 21 2021 09:18:53 GMT+0800 (中国标准时间)
Using the date object, we can get and set the date, time and UTC time.
2. Use strings to create date objects
We can also use strings to create dates. We can create a string containing a date and time and convert it to a date object using a Date object. Here is an example:
var dateStr = "2021-07-15T03:30:00.000Z"; var date = new Date(dateStr); console.log(date);
Executing this code will output the date and time represented by a string, for example:
Thu Jul 15 2021 11:30:00 GMT+0800 (中国标准时间)
In this example, "Z" represents Greenwich Mean Time. It indicates that the time passed is based on UTC (Coordinated Universal Time). If we want to use a different time zone, we need to convert it to UTC time.
3. Convert JavaScript string to date object
If we use JavaScript string to represent the date, we can use the built-in "Date.parse()" method to convert it into a date object. Here is an example:
var datestr = "2021-07-20"; var date = new Date(Date.parse(datestr)); console.log(date);
Executing this code will output the date represented by a string, for example:
Tue Jul 20 2021 08:00:00 GMT+0800 (中国标准时间)
Here, the "Date.parse()" method converts the string to UTC time. We can also pass the date and time together:
var datestr = "2021-07-20T13:30:00.000Z"; var date = new Date(Date.parse(datestr)); console.log(date);
Executing this code will output the date and time represented by a string, for example:
Tue Jul 20 2021 21:30:00 GMT+0800 (中国标准时间)
4. Using the methods and properties of the date object
Using date objects, we can get and set various parts of the date. For example, we can get the year using the following method:
var date = new Date(); var year = date.getFullYear(); console.log(year);
Executing this code will output the current year (e.g. 2021).
Similarly, we can get the month using the following method:
var date = new Date(); var month = date.getMonth(); console.log(month);
Executing this code will output the month of the current month (for example, 9 means September).
We can also get the date using the following method:
var date = new Date(); var day = date.getDate(); console.log(day);
Executing this code will output the current date (e.g. 21).
We can get the hour using:
var date = new Date(); var hour = date.getHours(); console.log(hour);
Executing this code will output the current hour (e.g. 9 means 9 am).
Similarly, we can get the minutes using:
var date = new Date(); var minute = date.getMinutes(); console.log(minute);
Executing this code will output the current minute (e.g. 18).
We can also get the seconds using:
var date = new Date(); var second = date.getSeconds(); console.log(second);
Executing this code will output the current number of seconds (e.g. 43).
5. Change the date object
We can also change the value of the date object. For example, we can use the following method to change the year:
var date = new Date(); console.log(date); date.setFullYear(2022); console.log(date);
Executing this code will first output the current date, and then output the set date (i.e. the current year is 2021, and the changed year is 2022).
Similarly, we can use the following method to change the month:
var date = new Date(); console.log(date); date.setMonth(9); console.log(date);
Executing this code will first output the current date, and then output the set date (i.e. the current month is 9, the changed month is 10).
We can also use the following method to change the date:
var date = new Date(); console.log(date); date.setDate(1); console.log(date);
Executing this code will first output the current date, and then output the set date (i.e. the current date is 21, and the changed date is 1 ).
6. Conclusion
Date and time are very important in JavaScript. We can use the built-in Date objects and methods to handle dates and times. We can create date objects, use methods and properties of date objects, and change the values of date objects. When using Date objects, please be aware of time zone and date formatting issues. Through studying this article, you have learned the basic knowledge of converting JavaScript to date objects. I hope it will be helpful to you.
The above is the detailed content of JavaScript to date. For more information, please follow other related articles on the PHP Chinese website!
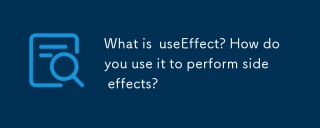
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
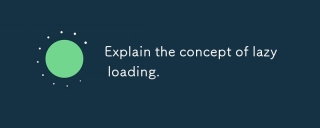
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
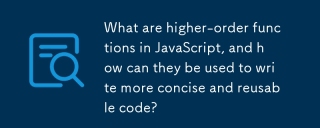
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
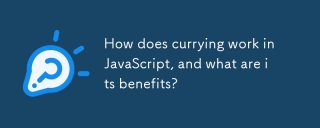
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
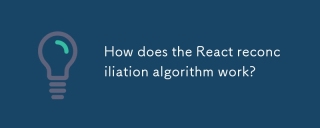
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
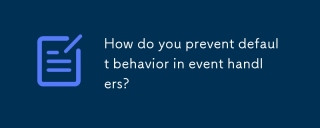
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
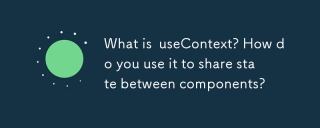
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
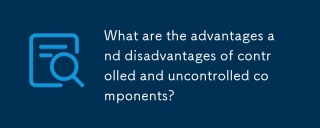
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
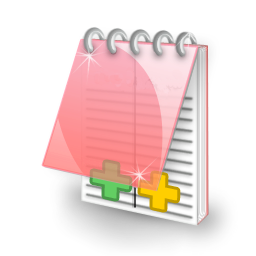
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use
