When writing PHP programs, we often need to read files and convert them into arrays to facilitate data manipulation. Converting php files to arrays is a common task and can be accomplished through the following methods.
1. Use the file_get_contents function
The file_get_contents function can read the file as a string. We can read the file into a string and then use the explode function to split the string into an array based on the delimiter we require. For example, we have a file data.txt which contains the following content:
Tom:24 Jerry:25 Kate:28
You can use the following code to convert it to an array:
$data = file_get_contents('data.txt'); $data_array = explode("\n", $data); foreach ($data_array as &$value) { $value = explode(':', trim($value)); } unset($value); print_r($data_array);
The output is as follows:
Array ( [0] => Array ( [0] => Tom [1] => 24 ) [1] => Array ( [0] => Jerry [1] => 25 ) [2] => Array ( [0] => Kate [1] => 28 ) )
2. Use the file function
The file function reads the entire file as an array, and each array element is a line in the file. Therefore, we can directly use the file function to read the file as an array. For example, we have a file data.txt which contains the following content:
Tom:24 Jerry:25 Kate:28
You can use the following code to convert it to an array:
$data_array = file('data.txt', FILE_IGNORE_NEW_LINES | FILE_SKIP_EMPTY_LINES); foreach ($data_array as &$value) { $value = explode(':', trim($value)); } unset($value); print_r($data_array);
The output is as follows:
Array ( [0] => Array ( [0] => Tom [1] => 24 ) [1] => Array ( [0] => Jerry [1] => 25 ) [2] => Array ( [0] => Kate [1] => 28 ) )
3. Use the fgets function
The fgets function can read the file content line by line. We can use the fgets function in a loop to read the file as an array. For example, we have a file data.txt which contains the following content:
Tom:24 Jerry:25 Kate:28
You can use the following code to convert it to an array:
$handle = fopen('data.txt', 'r'); $data_array = array(); while (!feof($handle)) { $line = fgets($handle); if ($line !== false) { $line = explode(':', trim($line)); $data_array[] = $line; } } fclose($handle); print_r($data_array);
The output is as follows:
Array ( [0] => Array ( [0] => Tom [1] => 24 ) [1] => Array ( [0] => Jerry [1] => 25 ) [2] => Array ( [0] => Kate [1] => 28 ) )
The above three methods can all convert files into arrays. Which method to choose depends on the actual application scenario. If the file content is small, it is recommended to use the file_get_contents or file function to read the file into a string or array, and then convert it to an array operation; if the file content is large, it is recommended to use the fgets function to read the file content line by line.
The above is the detailed content of How to convert php files to array. For more information, please follow other related articles on the PHP Chinese website!
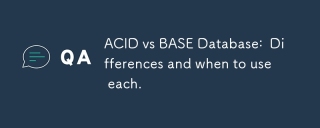
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
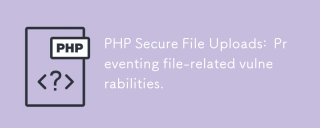
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
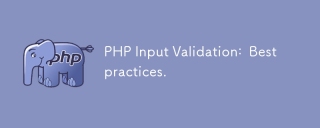
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
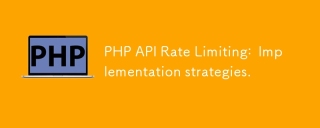
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
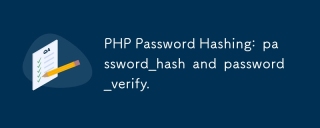
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
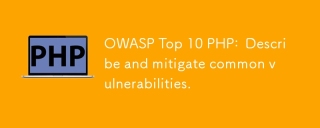
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
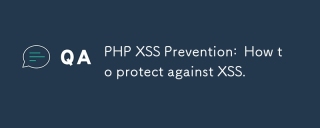
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
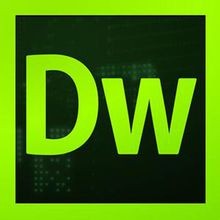
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
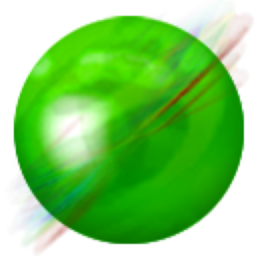
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment