With the rapid development of Golang, more and more people are trying to use it to implement various applications. However, Golang does not currently support generics, which makes it more difficult to write certain types of applications. This article will take a deep dive into how to implement generics using Golang to make it easier for developers to write more flexible and scalable applications using Golang.
What are generics?
In programming, generics are when you are not sure which data type to use when writing code. Simply put, generics can be applied to any type of data, rather than targeting a specific data type. This allows us to write more versatile code without having to copy and paste the same code to accommodate different data types.
In other languages, such as Java or C, generics have been widely adopted. They enable programmers to write efficient, clear and reusable code. However, implementing generics in Golang is a more challenging task because the Golang compiler does not have the same type inference and function template capabilities as other programming languages such as Java or C.
Methods to implement generics
Since the Golang compiler does not support generics, we need to use some techniques to achieve the effect of generics. Here are some methods:
- Empty interface
Golang’s empty interface can represent any type of data, which allows us to write universal code. Here is a simple example implemented using an empty interface, demonstrating how to create a generic PrintValue function:
func PrintValue(value interface{}) { fmt.Println(value) }
This function can accept any type of data and print it out. The empty interface provides us with a way to implement generics, but its drawback is that we need to manually convert types inside the function. This can become very tedious and expensive when dealing with large amounts of data.
- Reflection
Golang’s reflection makes it possible to query and modify the type and value of variables at runtime. By using reflection, we can write generic code that can handle any type of data and determine its type at runtime. Here is an example implemented using reflection, demonstrating how to create a generic PrintValue function:
func PrintValue(value interface{}) { v := reflect.ValueOf(value) fmt.Println(v) }
In this example, we use the ValueOf function of the reflect package to get the reflected value of the passed variable. This function returns a variable of type Value that represents the reflected value. We can further manipulate the reflected value by using some utility functions of the Value type, such as TypeOf and Kind.
Reflection allows the type and value of variables to be determined at runtime, which allows us to create generic code. However, the use of reflection increases execution time and memory footprint, which may affect program performance.
- Code Generator
A code generator is a program that reads the source code of a program and generates specific code based on a template. In Golang, we can use code generators to generate code for specific types, thereby avoiding type checking and conversion at runtime.
The code generator works as follows: First, use a method similar to text replacement to replace the type parameters in the source code with the actual type. Then, use the go generate command to generate new concrete code. Finally, the generated code is compiled and linked into the program.
Using a code generator can speed up program execution by avoiding type checking and conversion at runtime. However, doing so requires us to write additional source code to generate the specific code and is difficult to maintain.
Conclusion
Golang's generics support has received a lot of attention, but it does not yet provide the same type inference and function template capabilities as other programming languages. Nonetheless, we can use techniques such as empty interfaces, reflection, and code generators to achieve the effect of generics.
When using these technologies, we need to choose the best method based on the specific situation and needs. No matter which method we choose to use, we need to carefully consider its efficiency and maintainability to ensure that the code we write is efficient, clear, and reusable.
The above is the detailed content of How to implement generics in golang. For more information, please follow other related articles on the PHP Chinese website!
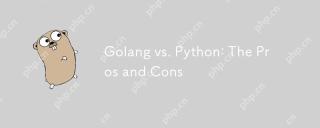
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
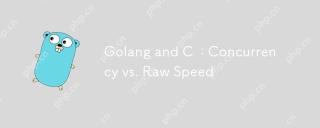
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
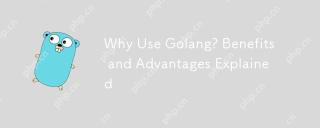
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
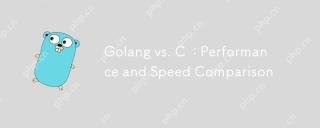
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
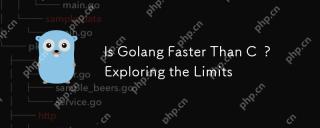
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
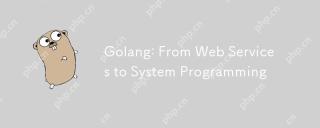
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
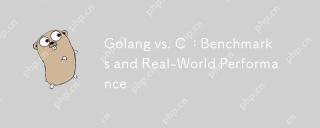
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
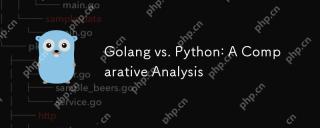
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
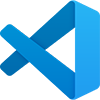
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
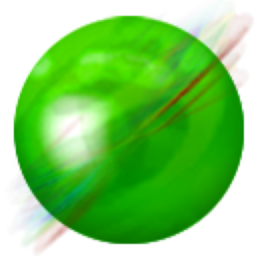
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
