Laravel is a popular PHP framework that provides a lot of features and tools to quickly build web applications. In actual development, we often need to process strings, such as intercepting characters. This article will introduce how to intercept characters in Laravel.
1. Use PHP built-in functions to intercept characters
In Laravel, we can use PHP built-in functions to intercept characters. PHP provides three functions to intercept strings: substr(), mb_substr() and mb_strcut().
- substr function
The substr function is one of PHP's built-in functions, used to intercept part of a string. This function takes three parameters: string, starting position, and length.
Sample code:
$str = "Hello world"; $substr = substr($str, 0, 5); echo $substr; //输出 Hello
In the sample code, $str is the string to be intercepted, 0 is the starting position, and 5 is the length to be intercepted. The substr function returns the intercepted string.
- mb_substr function
The mb_substr function is a string interception function for multi-byte character sets. Its parameters and usage are the same as the substr function, but it can handle multi-byte characters such as Chinese. It should be noted that using the mb_substr function requires enabling the mbstring extension in php.ini.
Sample code:
$str = "你好,世界"; $substr = mb_substr($str, 0, 2, 'utf-8'); echo $substr; //输出 你好
In the sample code, $str is the string to be intercepted, 0 is the starting position, 2 is the length to be intercepted, 'utf-8' represents the character string encoding. The mb_substr function returns the intercepted string.
- mb_strcut function
The mb_strcut function is also a string interception function for multi-byte character sets. Its parameters and usage are the same as the mb_substr function, but it can ensure that the intercepted result does not contain half a character.
Sample code:
$str = "你好,世界"; $substr = mb_strcut($str, 0, 2, 'utf-8'); echo $substr; //输出 你好
2. Use Laravel’s string operation functions to intercept characters
Laravel provides many string operation functions that can simplify our operation of intercepting strings .
- Str::substr function
Str::substr function is Laravel's string interception function. The parameters of this function are the same as those of the substr function.
Sample code:
use Illuminate\Support\Str; $str = "Hello world"; $substr = Str::substr($str, 0, 5); echo $substr; //输出 Hello
In the sample code, we need to introduce the Str class first, and then we can use the Str::substr function to intercept the string.
- Str::limit function
Str::limit function is Laravel's function to limit the string length. This function takes three parameters: string, length, and trailing character.
Sample code:
use Illuminate\Support\Str; $str = "Hello world"; $limitStr = Str::limit($str, 5, '...'); echo $limitStr; //输出 Hello...
In the sample code, $str is the string to be intercepted, 5 is the length to be intercepted, and '...' is the end character. The Str::limit function returns the intercepted string.
- Str::words function
Str::words function is Laravel’s function that limits the number of words. This function takes three parameters: string, number of words, and ending character.
Sample code:
use Illuminate\Support\Str; $str = "Hello world, how are you doing today?"; $wordsStr = Str::words($str, 3, '...'); echo $wordsStr; //输出 Hello world, how...
In the sample code, $str is the string to be intercepted, 3 is the number of words to be intercepted, and '...' is the ending character. The Str::words function returns the intercepted string.
Summary
This article introduces two methods of intercepting characters in Laravel: using PHP built-in functions and using Laravel's string manipulation functions. Multi-byte characters can be processed using PHP's built-in functions, but the mbstring extension needs to be enabled in php.ini; using Laravel's string manipulation functions can simplify development and limit the string length or number of words. Just choose different methods according to actual needs.
The above is the detailed content of How to intercept characters in laravel. For more information, please follow other related articles on the PHP Chinese website!

React,Vue,andAngularcanbeintegratedwithLaravelbyfollowingspecificsetupsteps.1)ForReact:InstallReactusingLaravelUI,setupcomponentsinapp.js.2)ForVue:UseLaravel'sbuilt-inVuesupport,configureinapp.js.3)ForAngular:SetupAngularseparately,servethroughLarave

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.

ToscaleaLaravelapplicationeffectively,focusondatabasesharding,caching,loadbalancing,andmicroservices.1)Implementdatabaseshardingtodistributedataacrossmultipledatabasesforimprovedperformance.2)UseLaravel'scachingsystemwithRedisorMemcachedtoreducedatab

Toovercomecommunicationbarriersindistributedteams,use:1)videocallsforface-to-faceinteraction,2)setclearresponsetimeexpectations,3)chooseappropriatecommunicationtools,4)createateamcommunicationguide,and5)establishpersonalboundariestopreventburnout.The

LaravelBladeenhancesfrontendtemplatinginfull-stackprojectsbyofferingcleansyntaxandpowerfulfeatures.1)Itallowsforeasyvariabledisplayandcontrolstructures.2)Bladesupportscreatingandreusingcomponents,aidinginmanagingcomplexUIs.3)Itefficientlyhandleslayou

Laravelisidealforfull-stackapplicationsduetoitselegantsyntax,comprehensiveecosystem,andpowerfulfeatures.1)UseEloquentORMforintuitivebackenddatamanipulation,butavoidN 1queryissues.2)EmployBladetemplatingforcleanfrontendviews,beingcautiousofoverusing@i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
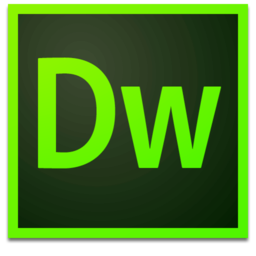
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor
