


Integrating JavaScript Frameworks (React, Vue, Angular) with a Laravel Backend
React, Vue, and Angular can be integrated with Laravel by following specific setup steps. 1) For React: Install React using Laravel UI, set up components in app.js. 2) For Vue: Use Laravel's built-in Vue support, configure in app.js. 3) For Angular: Set up Angular separately, serve through Laravel routes. Each integration requires attention to state management, performance, and API design for optimal results.
Integrating JavaScript Frameworks with a Laravel Backend: A Deep Dive into React, Vue, and Angular
So, you're looking to blend the power of modern JavaScript frameworks with Laravel's robust backend capabilities? Let's dive into how you can integrate React, Vue, and Angular with Laravel, and explore the nuances, best practices, and potential pitfalls of each approach.
When I first started working with these technologies, I was fascinated by how seamlessly they could work together, yet each integration posed its own unique challenges and learning curves. Let's unpack this journey together.
Why Choose Laravel for Your Backend?
Laravel stands out as an elegant and feature-rich PHP framework that's perfect for crafting modern, robust web applications. Its expressive syntax and comprehensive ecosystem make it an ideal choice for handling the backend logic of your application. But how do you marry this with the dynamic, interactive frontends provided by React, Vue, or Angular?
React and Laravel: A Symphony of Simplicity and Power
Integrating React with Laravel is like pairing a sleek sports car with a powerful engine. React's component-based architecture and virtual DOM make it incredibly efficient for building user interfaces, while Laravel provides a solid backend to handle data processing and API management.
Here's a simple way to set up React with Laravel:
// In your Laravel project, install React composer require laravel/ui php artisan ui react // Then, in your resources/js/app.js import React from 'react'; import ReactDOM from 'react-dom'; import Example from './components/Example'; if (document.getElementById('example')) { ReactDOM.render(<Example />, document.getElementById('example')); }
This setup allows you to use React components within your Laravel views. However, one potential pitfall is managing state across your application. While React's useState and useContext hooks are powerful, integrating them with Laravel's session or database can be tricky. My advice? Use a state management library like Redux or MobX to keep your frontend state in sync with your backend.
Vue.js and Laravel: A Match Made in Heaven
Vue.js is often praised for its ease of integration with Laravel, thanks to Laravel's built-in support for Vue. When I first integrated Vue with Laravel, I was amazed at how quickly I could prototype and build features.
Here's how you can get started:
// In your Laravel project, install Vue composer require laravel/ui php artisan ui vue // Then, in your resources/js/app.js import Vue from 'vue'; import Example from './components/Example.vue'; new Vue({ el: '#app', components: { Example } });
Vue's reactivity system works beautifully with Laravel's data-driven approach. But be cautious about performance. As your application grows, Vue's reactivity can sometimes lead to unnecessary re-renders. To mitigate this, use Vue's v-once
directive or optimize your component structure to minimize reactivity overhead.
Angular and Laravel: The Enterprise Powerhouse
Angular, with its TypeScript foundation and robust dependency injection system, is often the go-to choice for large-scale applications. Integrating Angular with Laravel requires a bit more setup, but the payoff is a highly maintainable and scalable application.
Here's how you can set up Angular with Laravel:
# In your Laravel project, set up Angular ng new frontend --directory=./public/angular cd frontend ng build --output-path=../public/angular/dist
Then, in your Laravel routes, you can serve the Angular app:
Route::get('/{any}', function () { return view('angular'); })->where('any', '.*');
Angular's strong typing and modular architecture make it easier to manage large codebases, but it can be overkill for smaller projects. Also, keep an eye on the initial load time; Angular's bundle size can be significant, so consider using lazy loading and tree shaking to optimize performance.
Common Challenges and Best Practices
Integrating any of these frameworks with Laravel comes with its own set of challenges. Here are some insights and best practices I've gathered over the years:
- API Design: Ensure your Laravel API is RESTful and well-documented. Use tools like Swagger or Postman to streamline API development and testing.
- Authentication: Implement a robust authentication system. Laravel's built-in authentication can be extended to work seamlessly with your frontend framework. Consider using JWT for stateless authentication.
- State Management: For React and Vue, consider using state management libraries to handle complex state logic. For Angular, leverage its built-in services and dependency injection.
- Performance Optimization: Use server-side rendering (SSR) or static site generation (SSG) to improve initial load times, especially for SEO purposes.
- Error Handling: Implement a unified error handling strategy across your frontend and backend. Use Laravel's exception handling to catch and log errors, and display them appropriately in your frontend.
Personal Experience and Tips
When I first integrated React with Laravel, I struggled with managing state across the application. I learned that using a state management library like Redux was crucial for maintaining a clean and scalable architecture. For Vue, I found that leveraging Laravel's built-in support made the integration process smoother, but I had to be mindful of performance as the application grew.
With Angular, the initial setup was more complex, but the benefits in terms of maintainability and scalability were undeniable. I've found that using Angular's CLI and leveraging its modular architecture can significantly streamline development.
In conclusion, integrating JavaScript frameworks with Laravel offers a powerful combination for building modern web applications. Each framework has its strengths and challenges, but with the right approach and best practices, you can create a seamless and efficient development experience. Whether you choose React, Vue, or Angular, the key is to understand the nuances of each integration and leverage their unique features to build something truly remarkable.
The above is the detailed content of Integrating JavaScript Frameworks (React, Vue, Angular) with a Laravel Backend. For more information, please follow other related articles on the PHP Chinese website!

React,Vue,andAngularcanbeintegratedwithLaravelbyfollowingspecificsetupsteps.1)ForReact:InstallReactusingLaravelUI,setupcomponentsinapp.js.2)ForVue:UseLaravel'sbuilt-inVuesupport,configureinapp.js.3)ForAngular:SetupAngularseparately,servethroughLarave

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.

ToscaleaLaravelapplicationeffectively,focusondatabasesharding,caching,loadbalancing,andmicroservices.1)Implementdatabaseshardingtodistributedataacrossmultipledatabasesforimprovedperformance.2)UseLaravel'scachingsystemwithRedisorMemcachedtoreducedatab

Toovercomecommunicationbarriersindistributedteams,use:1)videocallsforface-to-faceinteraction,2)setclearresponsetimeexpectations,3)chooseappropriatecommunicationtools,4)createateamcommunicationguide,and5)establishpersonalboundariestopreventburnout.The

LaravelBladeenhancesfrontendtemplatinginfull-stackprojectsbyofferingcleansyntaxandpowerfulfeatures.1)Itallowsforeasyvariabledisplayandcontrolstructures.2)Bladesupportscreatingandreusingcomponents,aidinginmanagingcomplexUIs.3)Itefficientlyhandleslayou

Laravelisidealforfull-stackapplicationsduetoitselegantsyntax,comprehensiveecosystem,andpowerfulfeatures.1)UseEloquentORMforintuitivebackenddatamanipulation,butavoidN 1queryissues.2)EmployBladetemplatingforcleanfrontendviews,beingcautiousofoverusing@i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
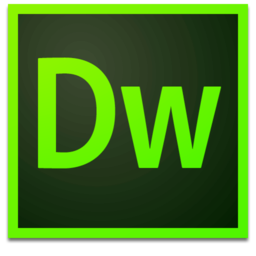
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
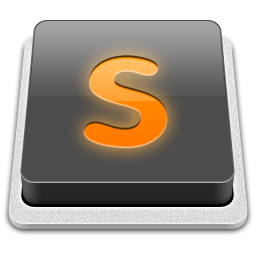
SublimeText3 Mac version
God-level code editing software (SublimeText3)
