In PHP programming, we often need to convert objects into json string arrays. This operation is very common in web development and can be used to pass data to the client or server, and also to share data between different applications. In this article we will explain how to convert an object into a json string array using php.
1. What is json?
JSON stands for JavaScript Object Notation, which is a lightweight data exchange format. JSON is based on JavaScript syntax, but does not depend on JavaScript, so it can be easily used in various programming languages. JSON uses key/value pairs to represent data, and its data structure is similar to a dictionary in Python. JSON represents data as an object (Object) or an array (Array). A JSON object is a collection of key/value pairs, where the key is a string and the value can be a string, number, Boolean value, array, object, etc. A JSON array is an ordered collection in which each element can be a value of any type (including objects and arrays).
Second, the json_encode function in php
The json_encode function in php is used to convert php variables into JSON format strings. Its syntax is as follows:
json_encode($value, $options = 0, $depth = 512)
$value: The variable to be converted to json format can be any data type, including Arrays, objects, Boolean types, integers, floating point numbers, null, etc.
$options: Optional parameters, used to determine the behavior of conversion. Common options include JSON_PRETTY_PRINT (formatted output), JSON_UNESCAPED_SLASHES (not escaping backslashes), etc. For a complete list, please refer to the official PHP documentation.
$depth: Optional parameter used to control the depth of conversion. Data beyond this depth will be converted to null. The default depth is 512.
The json_encode function converts the variable into a json format string that can be transmitted over the network or stored in a file.
Three, convert the object into a json string array
In PHP, converting the object into a json string array is very simple. We just need to convert the object into an associative array and then use the json_encode function to convert the array into a json string.
The following is a simple example that demonstrates how to convert an object in php to a json string array:
// Define a Person class
class Person
{
public $name; public $age; public $city; public function __construct($name, $age, $city) { $this->name = $name; $this->age = $age; $this->city = $city; }
}
// Instantiate the Person class
$person = new Person('Tom', 28, 'Shanghai');
// Convert object to associative array
$arr = [
'name' => $person->name, 'age' => $person->age, 'city' => $person->city,
];
// Convert array to json string
$json = json_encode($arr );
//Print json string
echo $json;
?>
The output result of the above code is:
{"name": "Tom","age":28,"city":"Shanghai"}
In the above example, we first define a Person class and instantiate an object. We then convert the object to an associative array and use the json_encode function to convert the array to a json string. Finally, we printed the json string on the screen.
Four, convert nested objects into json string arrays
If you want to convert nested objects into json string arrays, you need to recursively traverse the entire object tree and convert each sub-object into Convert to associative array. Here is an example that demonstrates how to convert a nested object into a json string array:
// Define a Person class
class Person
{
public $name; public $age; public $city; public function __construct($name, $age, $city) { $this->name = $name; $this->age = $age; $this->city = $city; }
}
// Define an Order class
class Order
{
public $id; public $customer; public function __construct($id, $customer) { $this->id = $id; $this->customer = $customer; }
}
// Instantiate the Person and Order classes
$person = new Person('Tom', 28, 'Shanghai');
$order = new Order(1001, $person);
// Convert nested objects to json string arrays
$arr = [
'id' => $order->id, 'customer' => [ 'name' => $order->customer->name, 'age' => $order->customer->age, 'city' => $order->customer->city, ],
];
// Convert array to json string
$json = json_encode($arr);
/ / Print json string
echo $json;
?>
The output result of the above code is:
{"id":1001,"customer":{"name ":"Tom","age":28,"city":"Shanghai"}}
In the above example, we defined two classes, Person and Order, and instantiated an Order object . We then convert the nested object into an associative array and use the json_encode function to convert the array into a json string. Finally, we printed the json string on the screen.
Five, summary
Converting objects in php to json string array is a very practical skill, it can effectively pass data to the client or server, and in different Share data between applications. In php, we can use the json_encode function to convert an object into a json string array. If you want to convert a nested object into a json string array, you need to recursively traverse the entire object tree and convert each sub-object into an associative array. Hope this article is helpful to everyone.
The above is the detailed content of How to convert object to json string array in php. For more information, please follow other related articles on the PHP Chinese website!
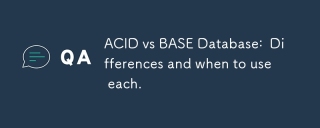
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
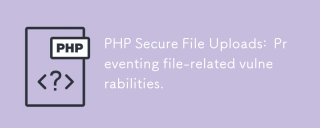
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
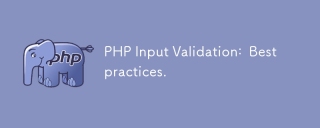
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
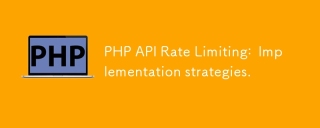
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
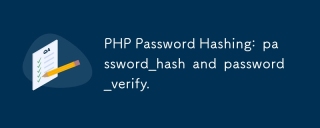
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
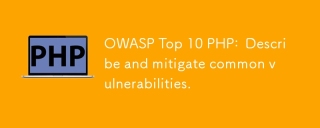
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
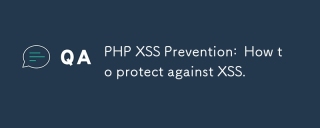
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
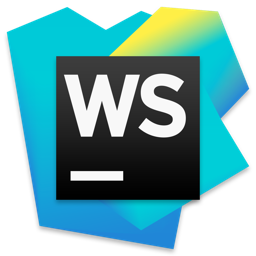
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
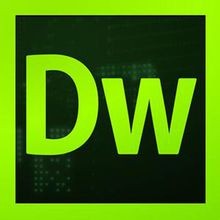
Dreamweaver CS6
Visual web development tools