Golang is a strong runtime programming language that can be used to develop high-availability applications. However, in actual development, we may encounter the problem of process shutdown. In this case, we need to use some tools and techniques in Golang to control the shutdown of the process.
This article will cover primitives, signals and other advanced techniques for process shutdown in Golang. We will explore these topics to help you better understand how to control the shutdown of Golang processes.
Primitives for process shutdown
In Golang, the most basic way to shut down a process is to use a two-way channel or cancel the channel. Bidirectional channel is the type of channel used in Golang for communication between different Goroutines. Cancellation channels are a Golang variant of bidirectional channels that can be used to communicate and cancel tasks between Goroutines.
Here, we will use the cancellation channel to demonstrate how to control the shutdown of the process. Here is an example of using a cancellation channel to control process shutdown:
func worker(cancel chan struct{}) { for { select { //检查是否收到取消指令 case <p> In this example, we create a <code>worker</code> function to simulate a worker process. The <code>worker</code> function uses an infinite loop to perform work, calling the <code>select</code> statement each time it loops to check whether a cancellation instruction has been received. If received, print a log and return, otherwise execute normal work logic. In the main function, we create a cancellation channel, start a worker subprocess, and wait five seconds to close the cancellation channel to stop the work. </p><p>After the cancellation channel is closed, the <code>worker</code> function will receive a <code>cancel</code> signal, and then it will exit the loop and return. </p><h3 id="Signals-for-process-shutdown">Signals for process shutdown</h3><p>In addition to closing the process by canceling the channel, we can also use process signals to control the shutdown of the Golang process. Signals are an operating system-level communication mechanism used to deliver messages and events within the system. In Linux systems, signals are very common. For example, when an application is executing, if it receives the <code>SIGKILL</code> signal, it will be forced to end. In Golang, we can use <code>os.Signal</code> to define signal types and <code>os.Notify</code> to subscribe to signal events. </p><p>The following is an example of using signals to control process shutdown: </p><pre class="brush:php;toolbar:false">func main() { //创建关闭信号 stop := make(chan os.Signal, 1) signal.Notify(stop, os.Interrupt) //启动工作子进程 go func() { for { log.Println("Worker: Doing some work") time.Sleep(time.Second * 1) } }() //等待收到关闭信号 <p>In this example, we create a channel called <code>stop</code> to respond to the shutdown signal, and Use <code>os.Notify</code> to subscribe to the <code>os.Interrupt</code> signal. The <code>os.Interrupt</code> signal is usually caused by the <code>Ctrl C</code> key. We also started a worker subprocess to simulate the job and output some messages on the console. </p><p>In the main function, we wait for the <code>stop</code> channel to receive data, that is, to receive the closing signal. When it happens, we print a log message and wait a second to allow the program to exit. </p><h3 id="Graceful-shutdown-of-the-process">Graceful shutdown of the process</h3><p>When we shut down the Golang process, there are some additional measures that can be taken to ensure that the process shuts down gracefully. These include closing other connections, waiting for running tasks to complete, saving data, etc. These measures help prevent data loss, file corruption, and other issues. </p><p>Here is an example that shows taking these extra steps when shutting down a process: </p><pre class="brush:php;toolbar:false">func main() { //创建关闭信号 stop := make(chan os.Signal, 1) signal.Notify(stop, os.Interrupt, os.Kill) //创建TCP服务器 listener, err := net.Listen("tcp", "localhost:8000") if err != nil { log.Fatal("Error:", err) } defer listener.Close() //启动RPC服务 srv := rpc.NewServer() srv.Register(&MathService{}) go srv.Accept(listener) //等待收到关闭信号 <p>In this example, we create a TCP server, RPC service, and shutdown signal. When we receive a shutdown signal, we shut down the server and wait for the running tasks to complete. Finally, we save the data to disk and wait for the program to exit. </p><h3 id="Summary">Summary</h3><p>In this article, we introduced how to use some tools and techniques in Golang to control the shutdown of the process. We learned how to use cancellation channels and signals to shut down processes. Additionally, we also discussed some techniques for gracefully shutting down processes, such as closing connections, waiting for tasks to complete, and saving data. </p><p>These tips can help us ensure the graceful shutdown of the process and avoid problems such as data loss and file corruption. Whether we are developing web servers, network applications, or other types of applications, process shutdown is very important. </p>
The above is the detailed content of How to shut down golang process. For more information, please follow other related articles on the PHP Chinese website!
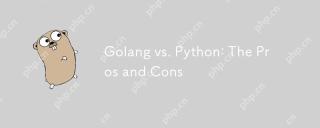
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
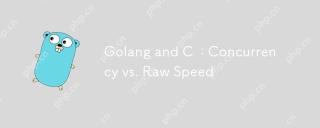
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
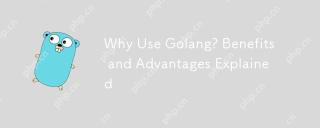
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
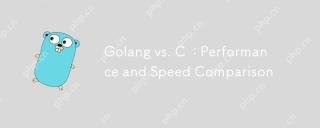
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
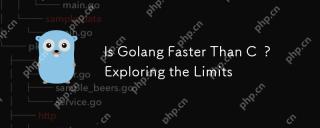
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
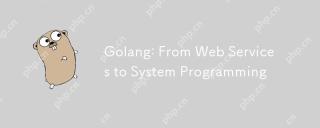
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
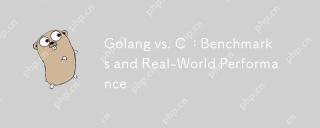
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
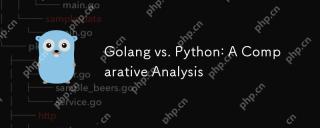
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!
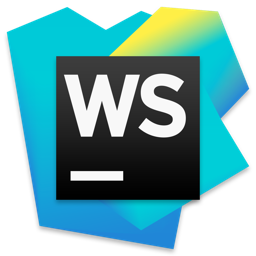
WebStorm Mac version
Useful JavaScript development tools
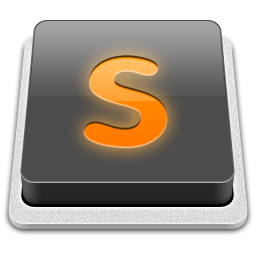
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version