Python is a beginner-friendly language. However, it also has many advanced features that are difficult to master, such as decorators. Many beginners have never understood decorators and how they work. In this article, we will introduce the ins and outs of decorators.
In Python, a function is a very flexible structure. We can assign it to a variable, pass it as a parameter to another function, or use it as the output of a function. A decorator is essentially a function that allows other functions to add some functionality without modification.
This is the meaning of "decoration". This "decoration" itself represents a function. If it is used to modify different functions, it will add this function to these functions.
Generally speaking, we can use the @ syntactic sugar (Syntactic Sugar) provided by the decorator to decorate other functions or objects. As shown below, we use the @dec decorator to decorate the function func ():
@dec def func(): pass
The best way to understand the decorator is to understand what problem the decorator solves. This article will introduce the decorator step by step starting from the specific problem, and Showcase its elegance and power.
Setting issues
To understand the purpose of the decorator, let's look at a simple example. Suppose you have a simple addition function dec.py with a default value of 10 for the second parameter:
# dec.py def add(x, y=10): return x + y
Let’s take a closer look at this addition function:
>>> add(10, 20) 30 >>> add <function add at 0x7fce0da2fe18> >>> add.__name__ 'add' >>> add.__module__ '__main__' >>> add.__defaults__ # default value of the `add` function (10,) >>> add.__code__.co_varnames # the variable names of the `add` function ('x', 'y')
We don’t need to understand What these are, just remember that every function in Python is an object and they have various properties and methods. You can also view the source code of the add() function through the inspect module:
>>> from inspect import getsource >>> print(getsource(add)) def add(x, y=10): return x + y
Now you use the addition function in some way, for example you use some operations to test the function:
# dec.py from time import time def add(x, y=10): return x + y print('add(10)', add(10)) print('add(20, 30)', add(20, 30)) print('add("a", "b")', add("a", "b")) Output: i add(10) 20 add(20, 30) 50 add("a", "b") ab
If you want to know the time of each operation, you can call the time module:
# dec.py from time import time def add(x, y=10): return x + y before = time() print('add(10)', add(10)) after = time() print('time taken: ', after - before) before = time() print('add(20, 30)', add(20, 30)) after = time() print('time taken: ', after - before) before = time() print('add("a", "b")', add("a", "b")) after = time() print('time taken: ', after - before) Output: add(10) 20 time taken:6.699562072753906e-05 add(20, 30) 50 time taken:6.9141387939453125e-06 add("a", "b") ab time taken:6.9141387939453125e-06
Now, as a programmer, are you a little itchy? After all, we don’t like to copy and paste the same code all the time. The current code is not very readable. If you want to change something, you have to modify everything where it appears. There must be a better way in Python.
We can capture the running time directly in the add function as follows:
# dec.py from time import time def add(x, y=10): before = time() rv = x + y after = time() print('time taken: ', after - before) return rv print('add(10)', add(10)) print('add(20, 30)', add(20, 30)) print('add("a", "b")', add("a", "b"))
This method is definitely better than the previous one. But if you have another function, then this seems inconvenient. When we have multiple functions:
# dec.py from time import time def add(x, y=10): before = time() rv = x + y after = time() print('time taken: ', after - before) return rv def sub(x, y=10): return x - y print('add(10)', add(10)) print('add(20, 30)', add(20, 30)) print('add("a", "b")', add("a", "b")) print('sub(10)', sub(10)) print('sub(20, 30)', sub(20, 30))
Because add and sub are both functions, we can take advantage of this to write a timer function. We want timer to calculate the operation time of a function:
def timer(func, x, y=10): before = time() rv = func(x, y) after = time() print('time taken: ', after - before) return rv
This is nice, but we must use the timer function to wrap different functions, as follows:
print('add(10)', timer(add,10)))
Now the default value Is it still 10? not necessarily. So how to do it better?
Here's an idea: create a new timer function, wrap other functions, and return the wrapped function:
def timer(func): def f(x, y=10): before = time() rv = func(x, y) after = time() print('time taken: ', after - before) return rv return f
Now, you just wrap add with timer And sub function:
add = timer(add)
That’s it! The following is the complete code:
# dec.py from time import time def timer(func): def f(x, y=10): before = time() rv = func(x, y) after = time() print('time taken: ', after - before) return rv return f def add(x, y=10): return x + y add = timer(add) def sub(x, y=10): return x - y sub = timer(sub) print('add(10)', add(10)) print('add(20, 30)', add(20, 30)) print('add("a", "b")', add("a", "b")) print('sub(10)', sub(10)) print('sub(20, 30)', sub(20, 30)) Output: time taken:0.0 add(10) 20 time taken:9.5367431640625e-07 add(20, 30) 50 time taken:0.0 add("a", "b") ab time taken:9.5367431640625e-07 sub(10) 0 time taken:9.5367431640625e-07 sub(20, 30) -10
Let's summarize the process: we have a function (such as the add function), and then wrap the function with an action (such as timing). The result of packaging is a new function that can implement certain new functions.
Of course, there is still something wrong with the default values, we will fix it later.
Decorator
Now, the above solution is very close to the idea of a decorator. It uses common behaviors to wrap a specific function. This pattern is decoration. What the device is doing. The code after using the decorator is:
def add(x, y=10): return x + y add = timer(add) You write: @timer def add(x, y=10): return x + y
Their functions are the same, this is the function of Python decorators. The function it implements is similar to add = timer(add), except that the decorator puts the syntax above the function, and the syntax is simpler: @timer.
# dec.py from time import time def timer(func): def f(x, y=10): before = time() rv = func(x, y) after = time() print('time taken: ', after - before) return rv return f @timer def add(x, y=10): return x + y @timer def sub(x, y=10): return x - y print('add(10)', add(10)) print('add(20, 30)', add(20, 30)) print('add("a", "b")', add("a", "b")) print('sub(10)', sub(10)) print('sub(20, 30)', sub(20, 30))
Parameters and keyword parameters
Now, there is still a small problem that has not been solved. In the timer function, we hard-code the parameters x and y, that is, specify the default value of y as 10. There is a way to pass arguments and keyword arguments to the function, namely *args and **kwargs. Parameters are the standard parameters of the function (in this case, x is the parameter), and keyword parameters are parameters that already have a default value (in this case, y=10). The code is as follows:
# dec.py from time import time def timer(func): def f(*args, **kwargs): before = time() rv = func(*args, **kwargs) after = time() print('time taken: ', after - before) return rv return f @timer def add(x, y=10): return x + y @timer def sub(x, y=10): return x - y print('add(10)', add(10)) print('add(20, 30)', add(20, 30)) print('add("a", "b")', add("a", "b")) print('sub(10)', sub(10)) print('sub(20, 30)', sub(20, 30))
Now, the timer function can handle any function, any parameters, and any default value settings, because it only passes these parameters into the function.
Higher Order Decorators
You may be wondering: if we can wrap a function with another function to add useful behavior, can we go one step further? Do we wrap a function with another function and be wrapped by another function?
Can! In fact, the function can be as deep as you want. For example, you want to write a decorator that executes a function n times. As shown below:
def ntimes(n): def inner(f): def wrapper(*args, **kwargs): for _ in range(n): rv = f(*args, **kwargs) return rv return wrapper return inner
Then you can use the above function to wrap another function, such as the add function in the previous article:
@ntimes(3) def add(x, y): print(x + y) return x + y
The output statement shows that the code is indeed executed 3 times.
The above is the detailed content of Understand Python decorators in one article. For more information, please follow other related articles on the PHP Chinese website!
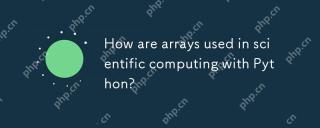
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
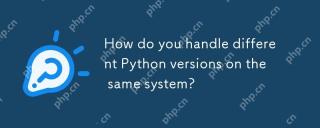
You can manage different Python versions by using pyenv, venv and Anaconda. 1) Use pyenv to manage multiple Python versions: install pyenv, set global and local versions. 2) Use venv to create a virtual environment to isolate project dependencies. 3) Use Anaconda to manage Python versions in your data science project. 4) Keep the system Python for system-level tasks. Through these tools and strategies, you can effectively manage different versions of Python to ensure the smooth running of the project.
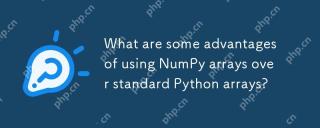
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making
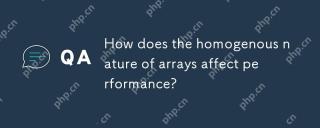
The impact of homogeneity of arrays on performance is dual: 1) Homogeneity allows the compiler to optimize memory access and improve performance; 2) but limits type diversity, which may lead to inefficiency. In short, choosing the right data structure is crucial.
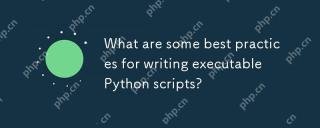
TocraftexecutablePythonscripts,followthesebestpractices:1)Addashebangline(#!/usr/bin/envpython3)tomakethescriptexecutable.2)Setpermissionswithchmod xyour_script.py.3)Organizewithacleardocstringanduseifname=="__main__":formainfunctionality.4
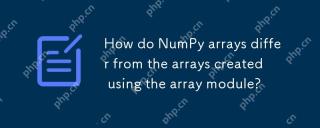
NumPyarraysarebetterfornumericaloperationsandmulti-dimensionaldata,whilethearraymoduleissuitableforbasic,memory-efficientarrays.1)NumPyexcelsinperformanceandfunctionalityforlargedatasetsandcomplexoperations.2)Thearraymoduleismorememory-efficientandfa
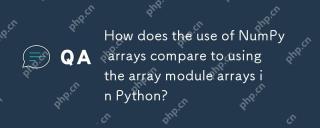
NumPyarraysarebetterforheavynumericalcomputing,whilethearraymoduleismoresuitableformemory-constrainedprojectswithsimpledatatypes.1)NumPyarraysofferversatilityandperformanceforlargedatasetsandcomplexoperations.2)Thearraymoduleislightweightandmemory-ef
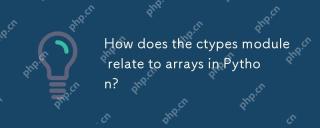
ctypesallowscreatingandmanipulatingC-stylearraysinPython.1)UsectypestointerfacewithClibrariesforperformance.2)CreateC-stylearraysfornumericalcomputations.3)PassarraystoCfunctionsforefficientoperations.However,becautiousofmemorymanagement,performanceo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
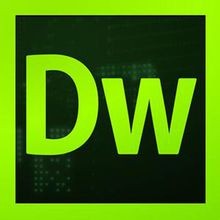
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
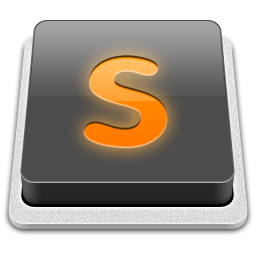
SublimeText3 Mac version
God-level code editing software (SublimeText3)
