It is a common thing to use Eloquent ORM to query the database in Laravel, but when we face some complex query requirements, we need to debug by viewing the executed SQL statements. So how to print the executed SQL statements?
Laravel provides two ways to print executed SQL statements, one is through log output, and the other is through event listener printing.
Through log output
SQL statements executed through log output in Laravel are the most convenient way, and there are corresponding log recording and viewing methods in each environment.
We can turn on the logging of SQL statements in the configuration file:
// 在config/database.php文件中,找到default下的connections数组,增加以下选项: 'log_queries' => true, // 开启SQL日志记录 'log_channel' => 'daily', // 日志存储方式,也可使用syslog、errorlog等方式 'log_level' => 'debug', // 日志级别
After adding the log_queries option, Laravel will automatically record the executed SQL statements, and the logs will be output to the storage/logs directory.
After executing the query statement in the code, we can output the SQL statement in the following way:
DB::enableQueryLog(); // 执行查询语句 $users = DB::table('users')->get(); // 获取执行的SQL语句 $sql = DB::getQueryLog()[0]['query'];
In the above code, first call the DB::enableQueryLog() method to enable recording of the SQL statement , after executing the query, you can obtain all executed SQL statements through the DB::getQueryLog() method. If you want to obtain the last SQL statement, you can also use the DB::getLastQuery() method.
However, it should be noted that using this method to record a large number of SQL statements in the production environment will cause the log volume to increase, which is not conducive to system maintenance and troubleshooting. Therefore, do not frequently record SQL statements in the production environment. Statement log.
Through the event listener
Laravel provides a way to listen to SQL execution events. Developers can output SQL statements by listening to SQL execution events. This method is safer and more reliable and will not be used in production. A large number of logs are generated in the environment.
By defining a listener, the corresponding SQL statement can be printed out when executing any query statement. The following is the implementation code:
// 在AppServiceProvider的boot方法中,添加以下代码 use Illuminate\Support\Facades\DB; use Illuminate\Database\Events\QueryExecuted; use Log; // 注册SQL执行监听器 DB::listen(function (QueryExecuted $queryExecuted) { $sql = str_replace("?", "'%s'", $queryExecuted->sql); $bindings = $queryExecuted->connection->prepareBindings($queryExecuted->bindings); $fullSql = vsprintf($sql, $bindings); Log::debug('SQL:'.$fullSql); });
In the above code, we use DB::listen() to register an event listener, which will be triggered when any query statement is executed.
In the listener, the SQL statement of the current query and related binding parameters and other information are obtained through the QueryExecuted event, and then output to the log through the Log::debug() method.
Through the above two methods, we have achieved the purpose of printing SQL statements in Laravel, which can make debugging and troubleshooting more convenient, and plays an irreplaceable role in development.
The above is the detailed content of How to print executed SQL statements in laravel (two methods). For more information, please follow other related articles on the PHP Chinese website!

React,Vue,andAngularcanbeintegratedwithLaravelbyfollowingspecificsetupsteps.1)ForReact:InstallReactusingLaravelUI,setupcomponentsinapp.js.2)ForVue:UseLaravel'sbuilt-inVuesupport,configureinapp.js.3)ForAngular:SetupAngularseparately,servethroughLarave

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.

ToscaleaLaravelapplicationeffectively,focusondatabasesharding,caching,loadbalancing,andmicroservices.1)Implementdatabaseshardingtodistributedataacrossmultipledatabasesforimprovedperformance.2)UseLaravel'scachingsystemwithRedisorMemcachedtoreducedatab

Toovercomecommunicationbarriersindistributedteams,use:1)videocallsforface-to-faceinteraction,2)setclearresponsetimeexpectations,3)chooseappropriatecommunicationtools,4)createateamcommunicationguide,and5)establishpersonalboundariestopreventburnout.The

LaravelBladeenhancesfrontendtemplatinginfull-stackprojectsbyofferingcleansyntaxandpowerfulfeatures.1)Itallowsforeasyvariabledisplayandcontrolstructures.2)Bladesupportscreatingandreusingcomponents,aidinginmanagingcomplexUIs.3)Itefficientlyhandleslayou

Laravelisidealforfull-stackapplicationsduetoitselegantsyntax,comprehensiveecosystem,andpowerfulfeatures.1)UseEloquentORMforintuitivebackenddatamanipulation,butavoidN 1queryissues.2)EmployBladetemplatingforcleanfrontendviews,beingcautiousofoverusing@i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
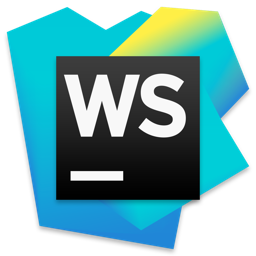
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
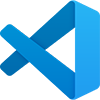
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
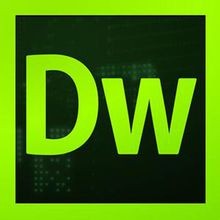
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
