


PHP is a popular open source server-side scripting language used for web development and writing dynamic web applications. There are many situations where we need to do number operations in programming and sometimes we need to preserve the decimal point. However, in PHP, division can produce some confusing results, especially when it involves decimal points. In this article, we will explore division operations and decimal point issues in PHP.
1. Basic division operation
In PHP, we can use the division sign (/) to perform basic division operation. For example, if we want to calculate the result of dividing 2 by 3, we can write the following code:
$result = 2 / 3; echo $result;
This will output 0.66666666666667. Note that the result is rounded to 14 decimal points. This is because computers use binary to represent numbers internally, so some decimal fractions cannot be represented accurately as binary.
2. The problem: Decimal point precision
In PHP, we can use the ini_set function to set the decimal point precision. Suppose we want to keep the decimal point to 5 places, we can use the following code:
ini_set('precision', 5); $result = 2 / 3; echo $result;
This will output 0.66667, which is exactly the result we want. However, if we change the number of digits, say change the precision to 3, the result becomes 0.667. This is because PHP will use rounding to preserve decimal points, but it won't automatically adjust up or down. So when the 6th decimal place is 5, PHP will round it to the 5th place instead of adjusting it upwards.
3. Rounding problem
In PHP, we can also use the round function to manually round decimal points. For example, if we wanted to round 0.66666666666667 to 2 decimal places, we could use the following code:
$result = 2 / 3; $rounded = round($result, 2); echo $rounded;
This would output 0.67, correctly rounded to the 2nd decimal place.
If we want to round the decimal point up or down, we can use the ceil and floor functions. The ceil function rounds a number up to the nearest integer, while the floor function rounds a number down to the nearest integer.
4. Avoid floating-point number comparison problems
In PHP, due to the machine representation of floating-point numbers, comparison of floating-point numbers is not always accurate. For example, if we wanted to compare 0.1 0.2 and 0.3 for equality, we might write the following code:
$a = 0.1 + 0.2; $b = 0.3; if($a == $b) { echo "相等"; } else { echo "不相等"; }
This would output "not equal" because the result of 0.1 0.2 is not equal to 0.3, even though they appear to be equal . To solve this problem, we can use the mathematical functions provided by PHP to compare floating point numbers. For example, we can use the abs and round functions to compare whether their difference is within the error range:
$a = 0.1 + 0.2; $b = 0.3; if(abs(round($a - $b, 10)) <p>This will output "equal" because their difference is within the error range. </p><p>5. Summary</p><p> In PHP, division can produce some confusing results, especially when decimal points are involved. To avoid these problems, we can set the precision of the decimal point, use functions such as rounding, rounding up or down, and use mathematical functions to compare floating point numbers. By understanding these techniques, we can better handle decimal division in PHP and write more accurate code. </p>
The above is the detailed content of An in-depth discussion of division operations and decimal point issues in PHP. For more information, please follow other related articles on the PHP Chinese website!
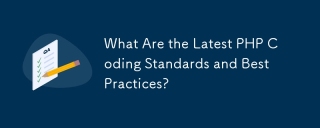
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
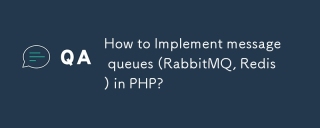
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
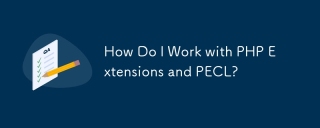
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
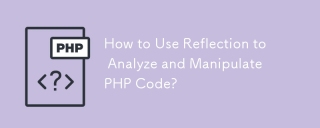
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
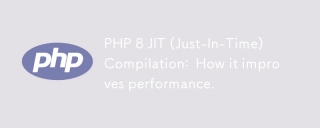
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
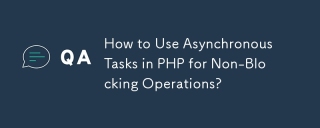
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
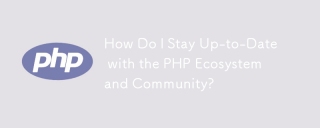
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
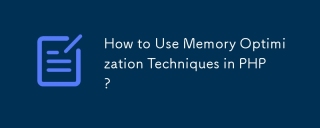
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
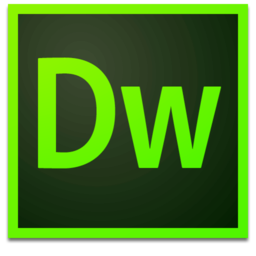
Dreamweaver Mac version
Visual web development tools
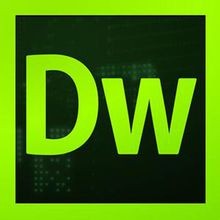
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
