The function of "react-dom" is to render the virtual DOM into the document and turn it into the actual DOM; "react-dom" is a toolkit that needs to be used when developing react projects. It provides DOM-specific methods, which can Used at the top level of the application, it can also be used as an interface for special DOM manipulation outside of the React model.
The operating environment of this tutorial: Windows 10 system, react17.0.1 version, Dell G3 computer.
What is the role of react-dom
When using react to develop a web page, two packages will be downloaded, one is react and the other is react-dom, where the react package is the core code of react. React-dom is the part related to DOM operations stripped out of React.
The core idea of react is virtual DOM. React includes the function react.createElement that generates virtual DOM, and the Component class. When we encapsulate components ourselves, we need to inherit the Component class to use life cycle functions, etc. The core function of the react-dom package is to render these virtual DOMs into documents and turn them into actual DOMs.
react-dom is a toolkit that needs to be used when developing react projects. It is a platform implementation for dom, mainly used for rendering on the web side. The react-dom package provides DOM-specific methods that can be used at the top level of the application or as an interface for special operations on the DOM outside of the React model.
react-dom mainly contains three APIs: findDOMNode, unmountComponentAtNode and render. The following is introduced in order of triggering.
1. render
render is used to render the virtual DOM rendered by React to the browser DOM, and is generally used in top-level components. This method mounts the element into the container and returns the instance of element (that is, the refs reference). If it is a stateless component, render will return null. When the component is loaded, the callback will be called. The syntax is:
render(ReactElement element,DOMElement container,[function callback])
For example:
import React from 'react' import ReactDOM from 'react-dom' import Router from './router' import { Provider } from 'react-redux' import store from './store' // Provider react-redux的内容 ReactDOM.render( <Provider store={store}> <Router/> </Provider>, document.getElementById('root'))
2, findDOMNode
findDOMNode is used to obtain the real DOM element in order to operate on the DOM node .
Before this, you must first know: In React, the virtual DOM is actually added to HTML and converted into a real DOM after the component is mounted (render()), so we can use componentDidMount and componentDidUpdate. Obtained in two ways. An example is as follows:
import { findDOMNode } from 'react-dom'; <Example ref={ node=>{ this.node = node} }> // 利用ref获取Example组件的实例 const dom = findDOMNode(this.node); // 通过findDOMNode获取实例对应的真实DOM
Note: When it comes to complex operations, there are many element DOM APIs available. However, DOM operations will have a great impact on performance, so DOM operations should be minimized.
3. unmountComponentAtNode
unmountComponentAtNode is used to perform unmount operations and is executed before componentWillUnmount.
Recommended learning: "react video tutorial"
The above is the detailed content of What does react-dom do?. For more information, please follow other related articles on the PHP Chinese website!

useState()isaReacthookusedtomanagestateinfunctionalcomponents.1)Itinitializesandupdatesstate,2)shouldbecalledatthetoplevelofcomponents,3)canleadto'stalestate'ifnotusedcorrectly,and4)performancecanbeoptimizedusinguseCallbackandproperstateupdates.

Reactispopularduetoitscomponent-basedarchitecture,VirtualDOM,richecosystem,anddeclarativenature.1)Component-basedarchitectureallowsforreusableUIpieces,improvingmodularityandmaintainability.2)TheVirtualDOMenhancesperformancebyefficientlyupdatingtheUI.

TodebugReactapplicationseffectively,usethesestrategies:1)AddresspropdrillingwithContextAPIorRedux.2)HandleasynchronousoperationswithuseStateanduseEffect,usingAbortControllertopreventraceconditions.3)OptimizeperformancewithuseMemoanduseCallbacktoavoid

useState()inReactallowsstatemanagementinfunctionalcomponents.1)Itsimplifiesstatemanagement,makingcodemoreconcise.2)UsetheprevCountfunctiontoupdatestatebasedonitspreviousvalue,avoidingstalestateissues.3)UseuseMemooruseCallbackforperformanceoptimizatio

ChooseuseState()forsimple,independentstatevariables;useuseReducer()forcomplexstatelogicorwhenstatedependsonpreviousstate.1)useState()isidealforsimpleupdatesliketogglingabooleanorupdatingacounter.2)useReducer()isbetterformanagingmultiplesub-valuesorac

useState is superior to class components and other state management solutions because it simplifies state management, makes the code clearer, more readable, and is consistent with React's declarative nature. 1) useState allows the state variable to be declared directly in the function component, 2) it remembers the state during re-rendering through the hook mechanism, 3) use useState to utilize React optimizations such as memorization to improve performance, 4) But it should be noted that it can only be called on the top level of the component or in custom hooks, avoiding use in loops, conditions or nested functions.

UseuseState()forlocalcomponentstatemanagement;consideralternativesforglobalstate,complexlogic,orperformanceissues.1)useState()isidealforsimple,localstate.2)UseglobalstatesolutionslikeReduxorContextforsharedstate.3)OptforReduxToolkitorMobXforcomplexst

ReusablecomponentsinReactenhancecodemaintainabilityandefficiencybyallowingdeveloperstousethesamecomponentacrossdifferentpartsofanapplicationorprojects.1)Theyreduceredundancyandsimplifyupdates.2)Theyensureconsistencyinuserexperience.3)Theyrequireoptim


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
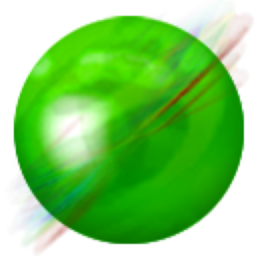
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
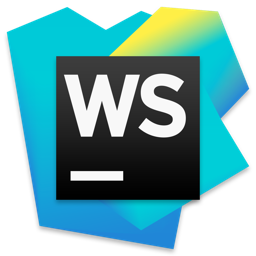
WebStorm Mac version
Useful JavaScript development tools
