


Detailed introduction to subprocess classes and constants in python
This article brings you a detailed introduction to the subprocess class and constants in python. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Constant
subprocess.DEVNULL: a special value that can be passed to the stdin, stdout, stderr parameters, which means that the special file os.devnull will be used to redirect input and output
subprocess .PIPE: A special value that can be passed to the stdin, stdout, stderr parameters, which means redirecting input and output using pipes
subprocess.STDOUT: A special value that can be passed to the stderr parameter, which means redirecting standard error to standard Output
Popen
Execute the subroutine in a new process.
Construction parameters
(args, bufsize=-1, executable=None, stdin=None, stdout=None, stderr=None, preexec_fn=None, close_fds=True, shell =False, cwd=None, env=None, universal_newlines=None, startupinfo=None, creationflags=0, restore_signals=True, start_new_session=False, pass_fds=(), *, encoding=None, errors=None, text=None)
args: String or sequence. If it is a sequence, the first element in args is the program to be executed; if it is a string, the interpretation is platform-dependent. On the POSIX system args will be interpreted as the name or path of the program to be executed (provided that it is not passed any parameters to the program).
shell: Specifies whether to use a shell as the program to be executed. If set to True, it is more recommended to use string type args parameters.
On POSIX systems, shell=True uses /bin/sh
as the shell by default. If args is a string, the string represents the command to be executed through the shell; if args is a sequence, the first element specifies the program to be executed, and the other elements are treated as parameters.
In Windows systems, shell=True defaults to the shell specified by the COMSPEC
environment variable, which is usually C:\WINDOWS\system32\cmd.exe
. The only scenario where you need to specify shell=True is when the instructions to be executed are built-in shells, such as dir
, copy
.
bufsize: When creating a stdin/stdout/stderr pipe file object, pass it as the corresponding parameter to the open()
function.
0: Do not use buffering
1: Use line buffering
Other positive integers: buffer size
Negative integers (default): Use system default values
executable: There are very few scenarios where shell=True is used. When shell=True, on POSIX systems, this parameter indicates specifying a new shell program to replace the default shell/bin/sh
.
stdin/stdout/stderr: Specify the standard input, standard output, and standard error of program execution respectively. Optional values include PIPE
, DEVNULL
, existing file descriptor (positive integer), existing file object, None. Child process file handles are inherited from the parent process. In addition, stderr can also be STDOUT
, indicating that standard error output is redirected to standard output.
preexec_fn: Limited to POSIX systems, sets a callable object to execute before the program in the child process.
close_fds: If False, the file descriptor follows the inheritable
identification described in Inheritance of File Descriptors.
If True, under POSIX systems, file descriptors other than 0, 1, and 2 are closed before the child process is executed.
pass_fds: POSIX only, optional sequence of file descriptors used to keep open between parent and child processes. Whenever this parameter is provided, close_fds is forced to True.
cwd: Change the working directory to cwd before the child process is executed, which can be a string or a path-like object.
restore_signals: Limited to POSIX, omitted
start_new_session: Limited to POSIX, omitted
env: A dict object that defines environment variables for the new process, replacing variables inherited from the parent process. Under Windows, to run side-by-side assembly
must include the available environment variable SystemRoot
. If env is specified, all environment variables that the program execution depends on must be provided
encoding/errors/text/universal_newlines: stdin/stdout/stderr defaults to binary mode Open. But if encoding/errors is specified or text is True, stdin/stdout/stderr will be opened in text mode using the specified encoding and errors. The universal_newlines parameter is equivalent to text for backward compatibility.
startupinfo: Windows only, omitted
creationflags: Windows only, omitted
Method
poll(): Check whether the child process has terminated. Return None to indicate not terminated, otherwise set the returncode attribute and return.
wait(timeout=None): If the child process does not terminate after timeout, a TimeoutExpired exception is thrown. Otherwise set the returncode attribute and return.
communicate(input=None, timeout=None): Process interaction: send data to stdin, read the data of stdout or stderr until the end character is read. Returns a tuple in the form of (stdout_data, stderr_data). The tuple type is string or byte.
Input is None or the data to be sent to the child process, which can be string or byte type depending on the stream opening mode.
If you want to interact with the stdin of the process, you need to specify stdin=PIPE when creating the Popen object. Similarly, if you want the returned tuple to be non-None, you need to set stdout=PIPE and/or stderr=PIPE.
If the child process does not terminate after timeout, a TimeoutExpired exception is thrown, but the child process is not killed. A good application should kill the child process and end the interaction:
proc = subprocess.Popen(...) try: outs, errs = proc.communicate(timeout=15) except TimeoutExpired: proc.kill() outs, errs = proc.communicate()
send_signal( signal): Send a signal to the child process
terminate(): Terminate the child process. On POSIX systems, send the SIGTERM signal to the child process. On Windows systems, TerminateProcess() will be called to terminate the process
kill(): Forcibly terminate the child process. On POSIX systems, send the SIGKILL signal to the child process. Kill() is an alias of terminate() on Windows systems
Attributes
args: Pass in the first parameter of the Popen
constructor, list or string type
stdin: If the stdin parameter passed to Popen is PIPE, this attribute represents a writable stream object of type string or byte. If the stdin parameter passed to Popen is not PIPE, the value of this attribute is None
stdout: Similar to Popen.stdin, but the stream object is readable
stderr: Similar to Popen.stdout
pid: The process ID of the child process. If shell=True is set, pid indicates the process number of the derived shell
#returncode: The child process return code, None indicates that the process has not terminated. A negative number -N means the process was terminated by signal N (POSIX only).
CompletedProcess
run()
The return value of the function operation indicates that the process execution is completed.
Attributes
args: Pass in the first parameter of the run()
function, list or string type
returncode: Exit code of the child process. If it is a negative number, it means that the process exited due to a certain signal
stdout: The standard output of the captured child process, the default is byte type, if the run()
function is called When encoding or errors are specified, or text=True is set, it is of string type. If the standard output is not captured, None is returned.
stderr: The standard error of the captured child process, the default is byte type, if encoding is specified when the run()
function is called Or errors, or string type if text=True is set. If standard error is not caught, None is returned. #Exception
subprocess.SubprocessError
Exception base class of subprocess modulesubprocess.TimeoutExpiredThe subprocess execution timeout.
Properties
cmd:Command
timeout:Time in seconds
output: The output of the child process captured by therun() or
check_output()function, otherwise None
stdout: output attribute aliasstderr: The error output of the subprocess captured by the
run()
function, otherwise None
subprocess.CalledProcessError
check_call() or check_output() Thrown when the function returns a non-0 status code. Attributes
The child process exit code. If it is a negative number, it means that the process exited due to a signal cmd:
Same as TimeoutExpired
Same as TimeoutExpired
stdout:Same as TimeoutExpired
stderr:Same as TimeoutExpiredThis article has ended here. For more other exciting content, you can follow PHP The
python video tutorialcolumn of the Chinese website!
The above is the detailed content of Detailed introduction to subprocess classes and constants in python. For more information, please follow other related articles on the PHP Chinese website!
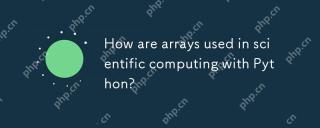
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
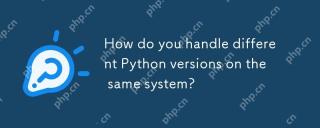
You can manage different Python versions by using pyenv, venv and Anaconda. 1) Use pyenv to manage multiple Python versions: install pyenv, set global and local versions. 2) Use venv to create a virtual environment to isolate project dependencies. 3) Use Anaconda to manage Python versions in your data science project. 4) Keep the system Python for system-level tasks. Through these tools and strategies, you can effectively manage different versions of Python to ensure the smooth running of the project.
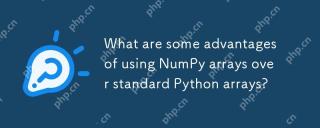
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making
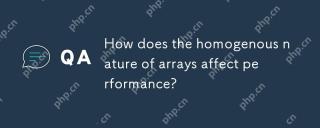
The impact of homogeneity of arrays on performance is dual: 1) Homogeneity allows the compiler to optimize memory access and improve performance; 2) but limits type diversity, which may lead to inefficiency. In short, choosing the right data structure is crucial.
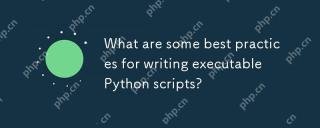
TocraftexecutablePythonscripts,followthesebestpractices:1)Addashebangline(#!/usr/bin/envpython3)tomakethescriptexecutable.2)Setpermissionswithchmod xyour_script.py.3)Organizewithacleardocstringanduseifname=="__main__":formainfunctionality.4
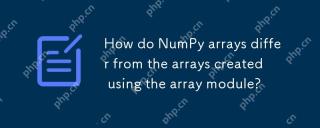
NumPyarraysarebetterfornumericaloperationsandmulti-dimensionaldata,whilethearraymoduleissuitableforbasic,memory-efficientarrays.1)NumPyexcelsinperformanceandfunctionalityforlargedatasetsandcomplexoperations.2)Thearraymoduleismorememory-efficientandfa
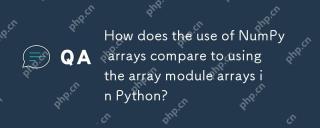
NumPyarraysarebetterforheavynumericalcomputing,whilethearraymoduleismoresuitableformemory-constrainedprojectswithsimpledatatypes.1)NumPyarraysofferversatilityandperformanceforlargedatasetsandcomplexoperations.2)Thearraymoduleislightweightandmemory-ef
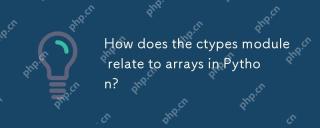
ctypesallowscreatingandmanipulatingC-stylearraysinPython.1)UsectypestointerfacewithClibrariesforperformance.2)CreateC-stylearraysfornumericalcomputations.3)PassarraystoCfunctionsforefficientoperations.However,becautiousofmemorymanagement,performanceo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
