The content of this article is about flask application (form processing) in Python. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
1. Why use Flask-WTF?
The request object exposes all request information sent by the client. In particular, request.form has access to form data submitted by POST requests.
Although the support provided by Flask's request object is sufficient to handle web forms, there are still many tasks that can become monotonous and repetitive.
Form HTML code generation and validation of submitted form data are two good examples.
Advantages:
Flask-WTF extension makes processing web forms a more pleasant experience. This extension is a Flask integration that wraps the framework-independent WTForms package.
2. What is form processing?
In a web page, some forms always have to appear in order to interact with users.
flask designed the WTForm form library to make it easier for flask to manage and operate form data.
The most important concepts in WTForm are as follows:
1). Form class, developer-defined forms must inherit from the Form class or its subclasses.
The main function of the Form class is to provide quick access to data in the form through the Field class it contains.
2). Various Field classes, namely fields. Generally speaking, each Field class corresponds to an input HTML tag.
For example, some of the Field classes that come with WTForm, such as BooleanField, correspond to ,
SubmitField corresponds to , etc.
3). Validator class. This class is used to verify the validity of data entered by the user.
For example, the Length validator can be used to verify the length of input data,
FileAllowed verifies the type of uploaded files, etc.
In addition, in order to prevent csfr (cross-site request forgery) attacks, flask requires the app to set secret_key by default before using flask-wtf. The simplest way to configure it is through app.config['SECRET_KEY'] = 'xxxx'.
3. Common Field classes
PasswordField Password field, automatically converts input into small black dots
DateField Text field, the format requirement is datetime .date is the same
IntergerField Text field, the format requirement is an integer
DecimalField Text field, the format requirement is the same as decimal.Decimal
FloatField Text field, the value is a floating point number
BooleanField Check box, the value is True or False
RadioField A set of radio button boxes
SelectField Drop-down list, it should be noted that the choices parameter determines the drop-down option, but it is different from Like the
MultipleSelectField A drop-down list with multiple optional values
Validator verification function
Validator is a verification function that binds a field to a verification function Afterwards, flask will verify the data before receiving the data in the form. If the verification is successful, the data will be received. The verification function Validator is as follows. Specific validators may require different parameters. Here are only some commonly used ones. For more detailed usage, please refer to the source code of the wtforms/validators.py file and see what parameters each validator class requires:
*Basically every validator has a message parameter, which indicates what information is displayed when the input data does not meet the requirements of the validator.
Email Verify the validity of the email address, the required regular pattern is ^. @(12 )$
EqualTo Compare two fields The value is usually used in scenarios such as entering a password twice. You can write the parameter fieldname, but note that it is a string variable pointing to the field name of another field in the same form
IPAddress Verify IPv4 address, parameters Default ipv4=True, ipv6=False. If you want to verify ipv6, you can set these two parameters in reverse.
Length Verify the length of the input string. You can have two parameters min and max to indicate the lower limit and upper limit of the length to be set. Note that the parameter type is a string, not INT!!
NumberRange To verify whether the input number is within the range, you can have two parameters, min and max, to indicate the upper and lower limits of the number. Note that the parameter type is a string, not INT!! Then you can set %(min)s and %( in the message parameter of this validator max)s two formatting parts to tell the front end what this range is. Other validators also have similar tricks, you can refer to the source code.
Optional Skip other validation functions in the same field when there is no input value
Required Required field
Regexp Use regular expression to verify the value, parameter regex='regular pattern'
URL To verify the URL, the required regular pattern is ^[a-z] ://(?P
AnyOf Make sure the value is in the list of optional values. The parameters are values (a list of optional values). In particular, when used in conjunction with SelectField, I don't know why the value of the items in SelectField's choices cannot be a number. . Otherwise, even if there are relevant numbers in the values parameter of AnyOf, it cannot be recognized that the current option is a legal option. I suspect NoneOf may have the same trick.
NoneOf Make sure the value is not in the list of optional values
#forms.py文件:用来设定规则 from flask_wtf import FlaskForm from flask_wtf.file import FileRequired, FileAllowed from wtforms import StringField, PasswordField, SubmitField, FileField from wtforms.validators import DataRequired, Length class LoginForm(FlaskForm): name = StringField( label="用户名/邮箱/手机号", validators=[ # DataRequired("请输入用户名"), Length(3, 20, message="长度不符"), ] ) passwd = PasswordField( label="密码", validators=[ # DataRequired("请输入密码"), Length(3, 20), ], ) file = FileField( label="简历", validators=[ FileRequired(), FileAllowed(['pdf', 'txt'], 'pdf 能被接收') ] )
#templates/demo/login.html nbsp;html> <meta> <title>Title</title>
#主程序 import random from flask import Flask, redirect, render_template from forms import LoginForm from flask_bootstrap import Bootstrap app = Flask(__name__) bootstrap = Bootstrap(app) app.config['SECRET_KEY'] = random._urandom(24) @app.route('/success/') def success(): return "success" @app.route('/login/', methods=('GET', 'POST')) def submit(): # 实例化表单对象; form = LoginForm() if form.validate_on_submit(): print(form.data) return redirect('/success/') return render_template('demo/login.html', form=form) app.run()
The above is the detailed content of Flask application (form processing) in python. For more information, please follow other related articles on the PHP Chinese website!
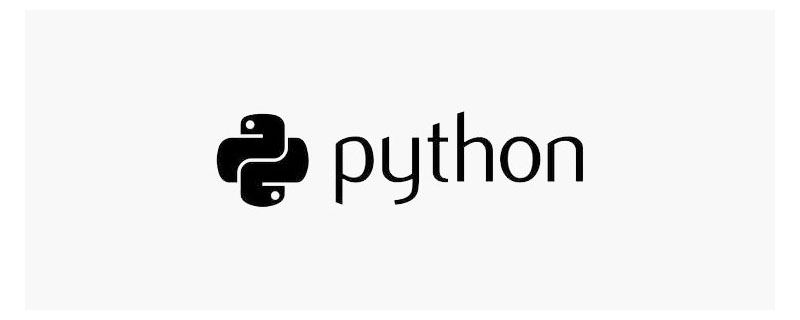
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
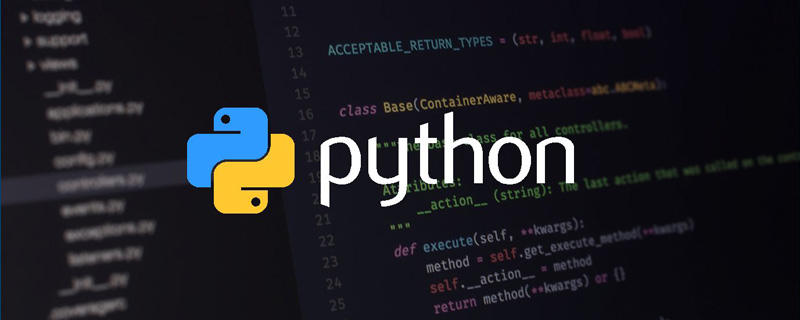
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
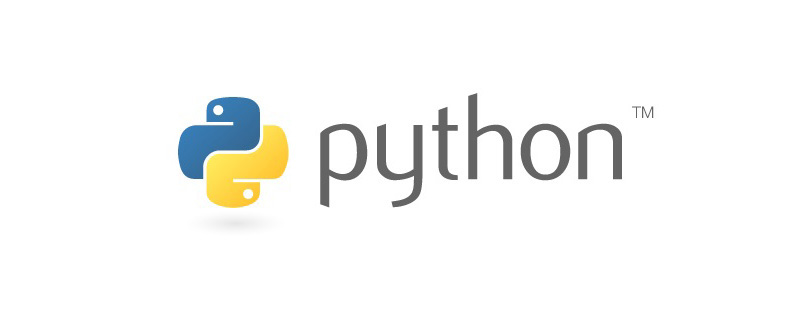
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
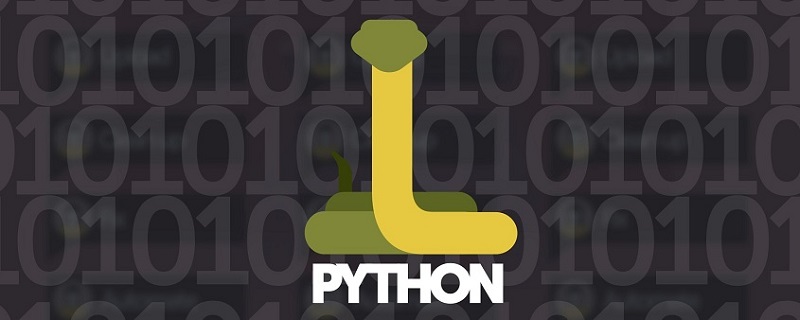
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
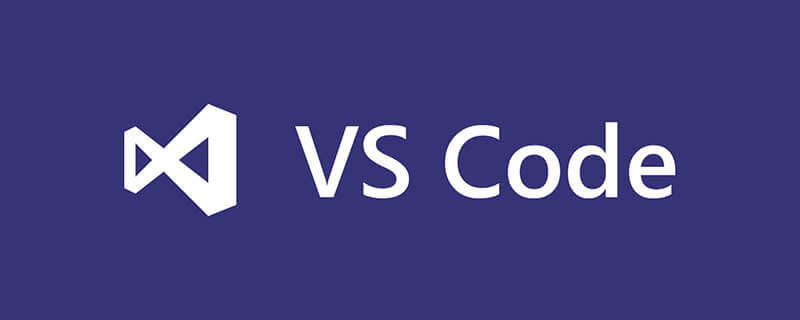
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
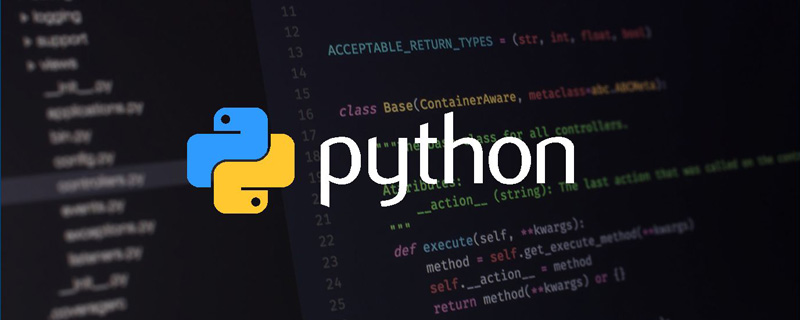
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
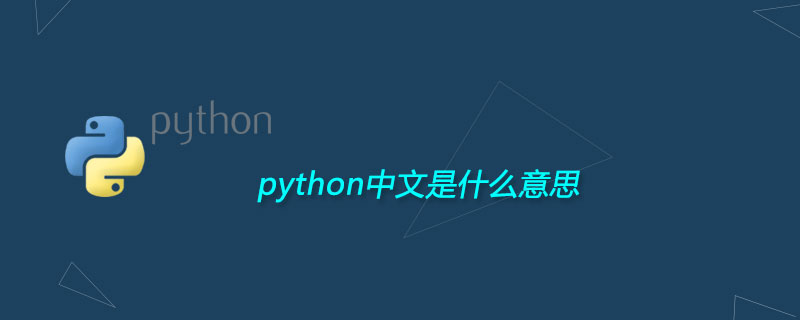
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
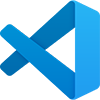
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
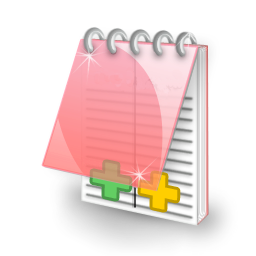
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
