Detailed explanation of socket module in python
The original English meaning of Socket is "hole" or "socket". As the process communication mechanism of BSD UNIX, it is also usually called "socket", which is used to describe the IP address and port. It is the handle of a communication chain and can be used to implement communication between different virtual machines or different computers.
Two programs on the network exchange data through a two-way communication connection. One end of this connection is called a socket.
At least a pair of port numbers (socket) is required to establish a network communication connection. Socket is essentially a programming interface (API), which encapsulates TCP/IP. TCP/IP also provides an interface for programmers to use for network development. This is the Socket programming interface; HTTP is a car that provides a way to encapsulate or display data. Specific form; Socket is the engine, providing the capability of network communication.
Let’s talk about python’s socket.
1.socket module
Use the socket.socket() function to create a socket. The syntax is as follows:
socket.socket(socket_family,socket_type,protocol=0)
socket_family can be the following parameters:
socket.AF_INET IPv4 (default)
socket.AF_INET6 IPv6
socket.AF_UNIX can only be used for inter-process communication in a single Unix system
socket_type can be the following parameters:
socket.SOCK_STREAM streaming socket, for TCP (default)
socket.SOCK_DGRAM Datagram socket, for UDP
socket.SOCK_RAW raw socket, ordinary socket cannot handle network packets such as ICMP and IGMP text, and SOCK_RAW can; secondly, SOCK_RAW can also handle special IPv4 messages; in addition, using raw sockets, the IP header can be constructed by the user through the IP_HDRINCL socket option.
socket.SOCK_RDM is a reliable form of UDP, which guarantees the delivery of datagrams but does not guarantee the order. SOCK_RAM is used to provide low-level access to the original protocol and is used when certain special operations need to be performed, such as sending ICMP messages. SOCK_RAM is usually restricted to programs run by power users or administrators.
socket.SOCK_SEQPACKET Reliable continuous packet service
protocol parameter:
0 (Default) The protocol related to the specific address family. If it is 0, the system will A suitable protocol will be automatically selected based on the address format and socket category
2. Socket object built-in methods
Server-side socket functions
s. bind() Bind the address (ip address, port) to the socket. The parameters must be in the format of tuples. For example: s.bind(('127.0.0.1',8009))
s.listen( 5) Start listening, 5 is the maximum number of pending connections
s.accept() Passively accept client connections, block, and wait for connections
Client socket function
s.connect() Connect to the server, the parameters must be in tuple format, for example: s.connect(('127,0.0.1',8009))
Public-purpose socket function
s.recv(1024) Receive TCP data, 1024 is the size of one data reception
s.send(bytes) Send TCP data, the format of python3 sending data must be bytes format
s.sendall() Send the data completely, and call send
s.close() in the internal loop to close the socket
Example 1. Simple implementation of socket program
server Terminal
#!/usr/bin/env python # _*_ coding:utf-8 _*_ import socket import time IP_PORT = ('127.0.0.1',8009) BUF_SIZE = 1024 tcp_server = socket.socket() tcp_server.bind(IP_PORT) tcp_server.listen(5) while True: print("waiting for connection...") conn,addr = tcp_server.accept() print("...connected from:",addr) while True: data = tcp_server.recv(BUF_SIZE) if not data:break tcp_server.send('[%s] %s'%(time.ctime(),data)) tcp_server.close()
Explanation of the above code:
1~4 lines
The first line is the Unix startup information line, and then the time module and socket module are imported
5~10 Lines
IP_PORT declares the IP address and port for the global variable, indicating that the bind() function is bound to this address, sets the buffer size to 1K, and the listen() function indicates that the maximum How many connections are allowed to come in at the same time, subsequent ones will be rejected
11~Go to the last line
After entering the server's loop, passively wait for the arrival of the connection. When there is a connection, enter the conversation loop and wait for the client to send data. If the message is empty, it means that the client has exited, and it will break out of the loop and wait for the next connection to arrive. After getting the client message, add a timestamp in front of the message and return. The last line will not be executed because the loop will not exit so the server will not execute close(). Just a reminder not to forget to call the close() function.
client side
#!/usr/bin/env python # _*_ coding:utf-8 _*_ import socket HOST = '127.0.0.1' PORT = 8009 BUF_SIZE = 1024 ADDR = (HOST,PORT) client = socket.socket() client.connect(ADDR) while True: data = input(">>> ") if not data:break client.send(bytes(data,encoding='utf-8')) recv_data = client.recv(BUF_SIZE) if not recv_data:break print(recv_data.decode()) client.close()
Lines 5~11
The HOST and PORT variables represent the IP address and port number of the server. Since the demonstration is on the same server, the IP addresses are all 127.0.0.1. If it is run on other servers, corresponding modifications must be made. The port number must be exactly the same as that of the server, otherwise communication will not be possible. The buffer size is still 1K.
The client socket is created in line 10 and then connects to the server
13~21 lines
The client also loops infinitely, and the client's loop is in the following two Exit after any one of the conditions occurs: 1. The user input is empty or the server-side response message is empty. Otherwise, the client will send the string entered by the user to the server for processing, and then receive the string with timestamp returned by the display server.
Run the client program and server program
The following is the input and output of the client
[root@pythontab]# python client.py >>> hello python [Thu Sep 15 22:29:12 2016] b'hello python'
The following is the output of the server
[root@pythontab]# python server.py waiting for connection... ...connected from: ('127.0.0.1', 55378)
3.socketserver module
socketserver is a high-level module in the standard library. Used to simplify the large amount of boilerplate code required to implement network clients and servers. Some classes that can be used have been implemented in the module.
Example 1: Use socketserver to achieve the same function as the above socket() example
服务端程序代码
#!/usr/bin/env python # _*_ coding:utf-8 _*_ import socketserver import time HOST = '127.0.0.1' PORT = 8009 ADDR = (HOST,PORT) BUF_SIZE = 1024 class Myserver(socketserver.BaseRequestHandler): def handle(self): while True: print("...connected from:",self.client_address) data = self.request.recv(BUF_SIZE) if not data:break self.request.send(bytes("%s %s"%(time.ctime(),data))) server = socketserver.ThreadingTCPServer(ADDR,Myserver) print("waiting for connection...") server.serve_forever()
11~17行
主要的工作在这里。从socketserver的BaseRequestHandler类中派生出一个子类,并重写handle()函数。
在有客户端发进来的消息的时候,handle()函数就会被调用。
19~21行
代码的最后一部分用给定的IP地址和端口加上自定义处理请求的类(Myserver)。然后进入等待客户端请求与处理客户端请求的无限循环中。
客户端程序代码
import socket HOST = '127.0.0.1' PORT = 8009 ADDR = (HOST,PORT) BUF_SIZE = 1024 client = socket.socket() client.connect(ADDR) while True: data = input(">>> ") if not data:continue client.send(bytes(data,encoding='utf-8')) recv_data = client.recv(BUF_SIZE) if not recv_data:break print(recv_data.decode()) client.close()
执行服务端和客户端代码
下面是客户端输出
[root@pythontab]# python socketclient.py >>> hello python Thu Sep 15 23:53:31 2016 b'hello python' >>> hello pythontab Thu Sep 15 23:53:49 2016 b'hello pythontab'
下面是服务端输出
[root@pythontab]# python socketserver.py waiting for connection... ...connected from: ('127.0.0.1', 55385) ...connected from: ('127.0.0.1', 55385) ...connected from: ('127.0.0.1', 55385) ...connected from: ('127.0.0.1', 55385)
The above is the detailed content of Detailed explanation of socket module in python. For more information, please follow other related articles on the PHP Chinese website!
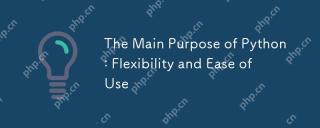
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
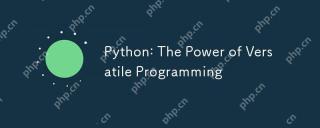
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
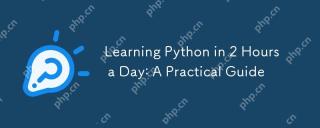
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.
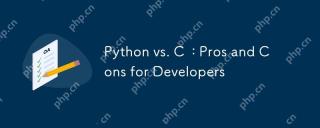
Python is suitable for rapid development and data processing, while C is suitable for high performance and underlying control. 1) Python is easy to use, with concise syntax, and is suitable for data science and web development. 2) C has high performance and accurate control, and is often used in gaming and system programming.
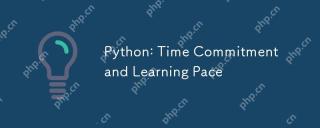
The time required to learn Python varies from person to person, mainly influenced by previous programming experience, learning motivation, learning resources and methods, and learning rhythm. Set realistic learning goals and learn best through practical projects.
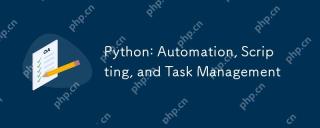
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
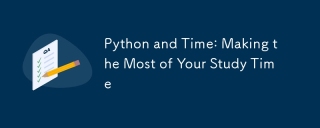
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
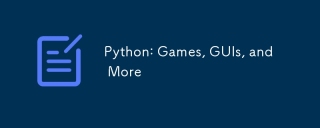
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
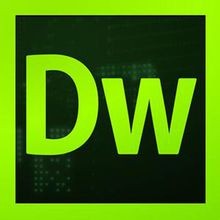
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
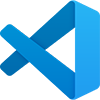
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft