When we write multi-threaded programs, we often encounter two types of variables.
One is a global variable, shared by multiple threads. In order to avoid changing the behavior, we have mentioned before that locking is required.
One is local variables. Only used by one thread, and threads do not affect each other.
For example, the count
variable defined in the task()
function in the following program is a local variable. Even if we create two threads, the increments of both count
will not affect each other, because count
is defined in task
.
import threading def task(): count = 0 for i in range(1000): count += 1 print count if __name__ == '__main__': t1 = threading.Thread(target=task) t1.start() t2 = threading.Thread(target=task) t2.start()
So, is it perfect to handle it this way? Not yet.
The above example is a very simple one, but when we encounter a more complex business logic, such as multiple local variables, multiple function calls, etc., defining local variables in this way will become unsuccinct. ,trouble.
Multiple calls of functions refer to, for example:
We have defined a function, methodA(), this method body calls methodB(), methodB() method body calls methodC()...
If we call methodA() in a thread and use a variable attr, then we need to pass attr to subsequent functions layer by layer.
Is there a way that allows us to define a variable in a thread, and then all functions in the thread can be called? This is simple and clear?
Python does it for us, that is ThreadLocal.
The usage of ThreadLocal only requires three steps:
Define an object threading.local
Bind parameters to the object within the thread. All bound parameters are thread-isolated.
Call within the thread.
The code is shown below:
# coding=utf-8 import threading local = threading.local() # 创建一个全局的对象 def task(): local.count = 0 # 初始化一个线程内变量,该变量线程间互不影响。 for i in range(1000): count_plus() def count_plus(): local.count += 1 print threading.current_thread().name, local.count if __name__ == '__main__': t1 = threading.Thread(target=task) t1.start() t2 = threading.Thread(target=task) t2.start()
For more python study notes - ThreadLocal related articles, please pay attention to the PHP Chinese website!
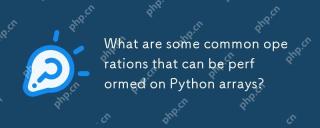
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
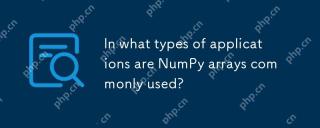
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
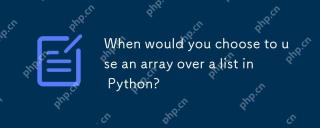
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf
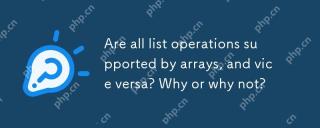
No,notalllistoperationsaresupportedbyarrays,andviceversa.1)Arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,whichimpactsperformance.2)Listsdonotguaranteeconstanttimecomplexityfordirectaccesslikearraysdo.
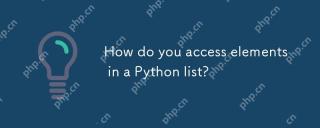
ToaccesselementsinaPythonlist,useindexing,negativeindexing,slicing,oriteration.1)Indexingstartsat0.2)Negativeindexingaccessesfromtheend.3)Slicingextractsportions.4)Iterationusesforloopsorenumerate.AlwayschecklistlengthtoavoidIndexError.
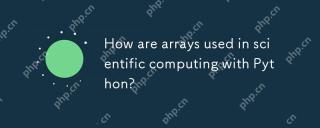
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
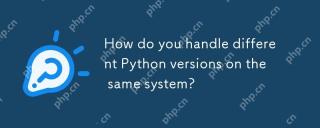
You can manage different Python versions by using pyenv, venv and Anaconda. 1) Use pyenv to manage multiple Python versions: install pyenv, set global and local versions. 2) Use venv to create a virtual environment to isolate project dependencies. 3) Use Anaconda to manage Python versions in your data science project. 4) Keep the system Python for system-level tasks. Through these tools and strategies, you can effectively manage different versions of Python to ensure the smooth running of the project.
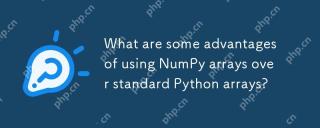
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
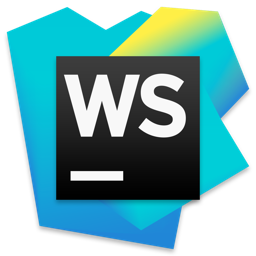
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
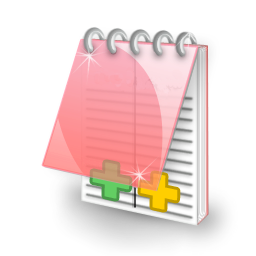
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
