Use python to simulate logging into Baidu
#!/usr/bin/python # -*- coding: utf-8 -*- import re; import cookielib; import urllib; import urllib2; import optparse; #------------------------------------------------------------------------------ # check all cookies in cookiesDict is exist in cookieJar or not def checkAllCookiesExist(cookieNameList, cookieJar) : cookiesDict = {}; for eachCookieName in cookieNameList : cookiesDict[eachCookieName] = False; allCookieFound = True; for cookie in cookieJar : if(cookie.name in cookiesDict) : cookiesDict[cookie.name] = True; for eachCookie in cookiesDict.keys() : if(not cookiesDict[eachCookie]) : allCookieFound = False; break; return allCookieFound; #------------------------------------------------------------------------------ # just for print delimiter def printDelimiter(): print '-'*80; #------------------------------------------------------------------------------ # main function to emulate login baidu def emulateLoginBaidu(): print "Function: Used to demostrate how to use Python code to emulate login baidu main page: http://www.baidu.com/"; print "Usage: emulate_login_baidu_python.py -u yourBaiduUsername -p yourBaiduPassword"; printDelimiter(); # parse input parameters parser = optparse.OptionParser(); parser.add_option("-u","--username",action="store",type="string",default='',dest="username",help="Your Baidu Username"); parser.add_option("-p","--password",action="store",type="string",default='',dest="password",help="Your Baidu password"); (options, args) = parser.parse_args(); # export all options variables, then later variables can be used for i in dir(options): exec(i + " = options." + i); printDelimiter(); print "[preparation] using cookieJar & HTTPCookieProcessor to automatically handle cookies"; cj = cookielib.CookieJar(); opener = urllib2.build_opener(urllib2.HTTPCookieProcessor(cj)); urllib2.install_opener(opener); printDelimiter(); print "[step1] to get cookie BAIDUID"; baiduMainUrl = "http://www.baidu.com/"; resp = urllib2.urlopen(baiduMainUrl); #respInfo = resp.info(); #print "respInfo=",respInfo; for index, cookie in enumerate(cj): print '[',index, ']',cookie; printDelimiter(); print "[step2] to get token value"; getapiUrl = "https://passport.baidu.com/v2/api/?getapi&class=login&tpl=mn&tangram=true"; getapiResp = urllib2.urlopen(getapiUrl); #print "getapiResp=",getapiResp; getapiRespHtml = getapiResp.read(); #print "getapiRespHtml=",getapiRespHtml; #bdPass.api.params.login_token='5ab690978812b0e7fbbe1bfc267b90b3'; foundTokenVal = re.search("bdPass\.api\.params\.login_token='(?P<tokenVal>\w+)';", getapiRespHtml); if(foundTokenVal): tokenVal = foundTokenVal.group("tokenVal"); print "tokenVal=",tokenVal; printDelimiter(); print "[step3] emulate login baidu"; staticpage = "http://www.baidu.com/cache/user/html/jump.html"; baiduMainLoginUrl = "https://passport.baidu.com/v2/api/?login"; postDict = { #'ppui_logintime': "", 'charset' : "utf-8", #'codestring' : "", 'token' : tokenVal, #de3dbf1e8596642fa2ddf2921cd6257f 'isPhone' : "false", 'index' : "0", #'u' : "", #'safeflg' : "0", 'staticpage' : staticpage, #http%3A%2F%2Fwww.baidu.com%2Fcache%2Fuser%2Fhtml%2Fjump.html 'loginType' : "1", 'tpl' : "mn", 'callback' : "parent.bdPass.api.login._postCallback", 'username' : username, 'password' : password, #'verifycode' : "", 'mem_pass' : "on", }; postData = urllib.urlencode(postDict); # here will automatically encode values of parameters # such as: # encode http://www.baidu.com/cache/user/html/jump.html into http%3A%2F%2Fwww.baidu.com%2Fcache%2Fuser%2Fhtml%2Fjump.html #print "postData=",postData; req = urllib2.Request(baiduMainLoginUrl, postData); # in most case, for do POST request, the content-type, is application/x-www-form-urlencoded req.add_header('Content-Type', "application/x-www-form-urlencoded"); resp = urllib2.urlopen(req); #for index, cookie in enumerate(cj): # print '[',index, ']',cookie; cookiesToCheck = ['BDUSS', 'PTOKEN', 'STOKEN', 'SAVEUSERID']; loginBaiduOK = checkAllCookiesExist(cookiesToCheck, cj); if(loginBaiduOK): print "+++ Emulate login baidu is OK, ^_^"; else: print "--- Failed to emulate login baidu !" else: print "Fail to extract token value from html=",getapiRespHtml; if __name__=="__main__": emulateLoginBaidu();
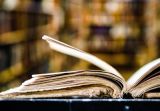
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
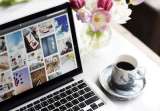
Dealing with noisy images is a common problem, especially with mobile phone or low-resolution camera photos. This tutorial explores image filtering techniques in Python using OpenCV to tackle this issue. Image Filtering: A Powerful Tool Image filter
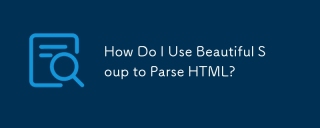
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
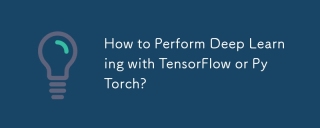
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
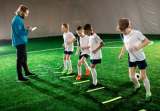
Python, a favorite for data science and processing, offers a rich ecosystem for high-performance computing. However, parallel programming in Python presents unique challenges. This tutorial explores these challenges, focusing on the Global Interprete
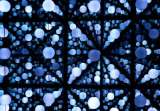
This tutorial demonstrates creating a custom pipeline data structure in Python 3, leveraging classes and operator overloading for enhanced functionality. The pipeline's flexibility lies in its ability to apply a series of functions to a data set, ge
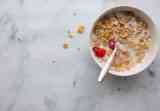
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
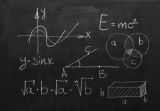
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
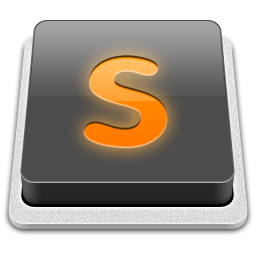
SublimeText3 Mac version
God-level code editing software (SublimeText3)
