The so-called singleton means that an instance of a class can only be created once from beginning to end.
Method 1
If you want to make a class have at most one instance from beginning to end, it is very simple to use the __new__ method. Classes in Python create instances through __new__:
class Singleton(object): def __new__(cls,*args,**kwargs): if not hasattr(cls,'_inst'): cls._inst=super(Singleton,cls).__new__(cls,*args,**kwargs) return cls._inst if __name__=='__main__': class A(Singleton): def __init__(self,s): self.s=s a=A('apple') b=A('banana') print id(a),a.s print id(b),b.s
Result:
29922256 banana
29922256 banana
Use the __new__ method to bind the instance of the class to when it is created Class attribute_inst on. If cls._inst is None, it means that the class has not been instantiated. Instantiate and bind the instance to cls._inst. The instance created by the first instantiation will be returned every time it is instantiated in the future. Note that when deriving a subclass from Singleton, do not overload __new__.
Method 2:
Sometimes we don’t care whether the generated instances have the same id, but only care about its status and behavior. We can allow many instances to be created, but all
class Borg(object): _shared_state={} def __new__(cls,*args,**kwargs): obj=super(Borg,cls).__new__(cls,*args,**kwargs) obj.__dict__=cls._shared_state return obj
will point the __dict__ of all instances to the same dictionary, so that the instances share the same methods and properties. The setting of the name attribute of any instance, whether modified in __init__ or directly, will affect all instances. However, the instance ids are different. To ensure that class instances can share attributes, but not with subclasses, be sure to use cls._shared_state instead of Borg._shared_state.
Because the instances have different ids, each instance can be used as a dictionary key:
if __name__=='__main__': class Example(Borg): pass a=Example() b=Example() c=Example() adict={} j=0 for i in a,b,c: adict[i]=j j+=1 for i in a,b,c: print adict[i]
Result:
0
1
2
If this behavior is not what you want, You can add __eq__ and __hash__ methods to the Borg class to make it closer to the behavior of the singleton pattern:
class Borg(object): _shared_state={} def __new__(cls,*args,**kwargs): obj=super(Borg,cls).__new__(cls,*args,**kwargs) obj.__dict__=cls._shared_state return obj def __hash__(self): return 1 def __eq__(self,other): try: return self.__dict__ is other.__dict__ except: return False if __name__=='__main__': class Example(Borg): pass a=Example() b=Example() c=Example() adict={} j=0 for i in a,b,c: adict[i]=j j+=1 for i in a,b,c: print adict[i]
Result:
2
2
2
All instances can When a key is used.
Method 3
When you write a class, some mechanism will use the class name, base class tuple, and class dictionary to create a class object. This mechanism in new classes defaults to type, and this mechanism is programmable and is called metaclass __metaclass__.
class Singleton(type): def __init__(self,name,bases,class_dict): super(Singleton,self).__init__(name,bases,class_dict) self._instance=None def __call__(self,*args,**kwargs): if self._instance is None: self._instance=super(Singleton,self).__call__(*args,**kwargs) return self._instance if __name__=='__main__': class A(object): __metaclass__=Singleton a=A() b=A() print id(a),id(b)
Result:
34248016 34248016
id is the same.
In the example, we construct a Singleton metaclass and use the __call__ method to enable it to simulate the behavior of the function. When constructing class A, set its metaclass to Singleton, then when creating class object A, the behavior occurs as follows:
A=Singleton(name, bases, class_dict), A is actually an instance of the Singleton class.
When creating an instance of A, A()=Singleton(name,bases,class_dict)()=Singleton(name,bases,class_dict).__call__(), thus pointing all instances of A to the attributes of A_ On the instance, this method is actually the same as method 1.
Method 4
The module module in python is only loaded once in the program and is a singleton itself. You can directly write a module and write the methods and attributes you need in the module as functions and global variables in the module scope. There is no need to write a class at all.
And there are some ways to combine the advantages of modules and classes:
class _singleton(object): class ConstError(TypeError): pass def __setattr__(self,name,value): if name in self.__dict__: raise self.ConstError self.__dict__[name]=value def __delattr__(self,name): if name in self.__dict__: raise self.ConstError raise NameError import sys sys.modules[__name__]=_singleton()
Python does not check sys.modules to ensure that they are module objects, we use this to bind the module to a class object, and will be bound to the same object in the future.
Store the code in single.py:
>>> import single >>> single.a=1 >>> single.a=2
ConstError
>>> del single.a
ConstError
Method 5
The simplest method:
class singleton(object): pass singleton=singleton()
Bind the name singleton to the instance, and the singleton is the only object of its own class.
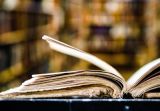
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
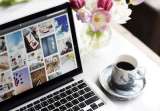
Dealing with noisy images is a common problem, especially with mobile phone or low-resolution camera photos. This tutorial explores image filtering techniques in Python using OpenCV to tackle this issue. Image Filtering: A Powerful Tool Image filter
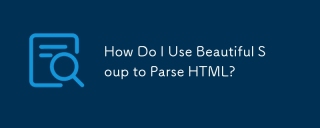
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
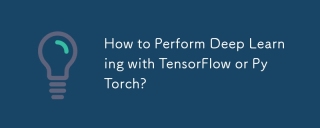
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
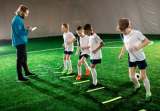
Python, a favorite for data science and processing, offers a rich ecosystem for high-performance computing. However, parallel programming in Python presents unique challenges. This tutorial explores these challenges, focusing on the Global Interprete
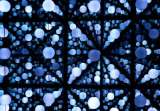
This tutorial demonstrates creating a custom pipeline data structure in Python 3, leveraging classes and operator overloading for enhanced functionality. The pipeline's flexibility lies in its ability to apply a series of functions to a data set, ge
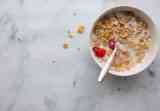
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
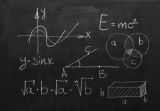
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
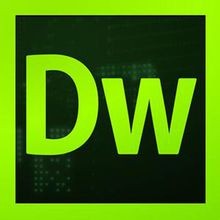
Dreamweaver CS6
Visual web development tools
