You should be familiar with HTML forms. The following code is a very simple HTML form:
Last name:
Name:
When you enter data and press the submit button, this form will send the data to submitform.php3. This PHP script then processes the received data. Here is the code of submitform.php3:
mysql_connect (localhost, username, password);
mysql_select_db (dbname) ;
mysql_query ("INSERT INTO tablename (first_name, last_name)
VALUES ('$first_name', '$last_name')
");
print ($first_name);
print (" ");
print ($last_name) ;
print ("");
print ("Thanks for filling out the registration form");
?>
In the third line of code, "username " and "password" respectively represent your account number and password to log in to the MySQL database. "dbname" in the fifth line represents the name of the MySQL database. "tablename" in line 13 is the name of a table in the database.
When you press submit, you can see the name you entered displayed on a new page. Take another look at the URL bar of the browser. Its content should be like this:
…/submitform.php3?first_name=Fred&last_name=Flintstone
Because we are using the form GET method, the data is transmitted to the URL through the URL. submitform.php3. Obviously, the GET method has limitations. When there is a lot of content to be transferred, GET cannot be used and only the POST method can be used. But no matter what method is used, when the data transfer is completed, PHP automatically creates a variable for each field in the form that is the same as their name (the name attribute of the form).
PHP variables all start with a dollar sign. In this way, during the processing of the submitform.php3 script, there will be two variables $first_name and $last_name. The content of the variables is what you input.
Let’s check whether the name you entered has actually been entered into the database. Start MySQL and enter at the mysql> prompt:
mysql> select * from tablename;
You should be able to get a table with the content you just entered:
+------------+ ------------+
| first_name | last_name |
+------------+------------+
| Liu | Rufeng
+----------------+------------+
1 rows in set (0.00 sec)
Let’s analyze how submitform.php3 works Working:
The first two lines of the script are:
mysql_connect (localhost, username, password);
mysql_select_db (dbname);
These two function calls are used to open the MySQL database. The meaning of the specific parameters has just been mentioned.
The following line executes a SQL statement:
mysql_query ("INSERT INTO tablename (first_name, last_name)
VALUES ('$first_name', '$last_name')
");
The mysql_query function is used to query the selected database Execute a SQL query. You can execute any SQL statement in the mysql_query function. The SQL statement to be executed must be enclosed in double quotes as a string, and variables within it must be enclosed in single quotes.
There is one thing to note: MySQL statements must end with a semicolon (;), and the same is true for a line of PHP code, but MySQL statements in PHP scripts cannot have semicolons. That is, when you enter a MySQL command at the mysql> prompt, you should add a semicolon:
INSERT INTO tablename (first_name, last_name)
VALUES ('$first_name', '$last_name');
But if When this command appears in a PHP script, the semicolon must be removed. The reason for this is that some statements, such as SELECT and INSERT, work with or without semicolons. But there are some statements, such as UPDATE, which won't work if you add a semicolon. To avoid trouble, just remember this rule.
How to extract data from MySQL with PHP
Now we create another HTML form to perform this task:
Please enter your query Content:
Last name:
First name:
Similarly, there will be a php script to process this form, and we will create a searchform .php3 file:
'%';}
if ($last_name == "")
{$last_name = '%';}
$result = mysql_query ("SELECT * FROM tablename
WHERE first_name LIKE '$first_name%'
AND last_name LIKE ' $last_name%'
");
if ($row = mysql_fetch_array($result)) {
do {
print $row["first_name"];
print (" ");
print $row["last_name"] ;
print ("");
} while($row = mysql_fetch_array($result));
} else {print "Sorry, no matching record was found in our database. ";}
?>
When you enter the content you want to retrieve in the form and press the SUBMIT button, you will enter a new page, which lists All matching search results are found. Let’s take a look at how this script completes the search task. The previous statements are the same as mentioned above. First, the database connection is established, and then the database and data table are selected. These are. Necessary for every database application. Then there are several statements like this:
if ($first_name == "")
{$first_name = '%';}
if ($last_name == "")
{$last_name = '%';}
These lines are used to check whether each field of the form is empty. Pay attention to the two equal signs, because most of PHP's syntax is derived from the C language, and the usage of the equal signs here is also the same as that of C. : One equal sign is an assignment sign, and two equal signs represent logical equality. It should also be noted that when the condition after IF is true, the statements to be executed later are placed in "{" and "}", and Each statement must be followed by a semicolon to indicate the end of the statement. The percent sign % is a wildcard character in the SQL language. After understanding one point, you should know the meaning of these two lines: If the "FIRST_NAME" field is empty. , then all FIRST_NAME will be listed. The following two sentences have the same meaning.
$result = mysql_query ("SELECT * FROM tablename
WHERE first_name LIKE '$first_name%'
AND last_name LIKE '$last_name%'"
" );
This line does most of the work of searching. When the mysql_query function completes a query, it returns an integer flag.
The query selects from all records those records whose first_name column is the same as the $first_name variable, and whose last_name column and $last_name variable values are also the same, put them into the temporary record set, and use the returned integer as the mark of this record set.
if ($row = mysql_fetch_array($result)) {
do {
print $row["first_name"];
print (" ");
print $row["last_name"];
print ("} while($row = mysql_fetch_array($result));
} else {print "Sorry, no matching record was found in our database. ";}
This is the last step, which is to display. Partially. The mysql_fetch_array function first extracts the content of the first row of the query result, and then displays it using the PRINT statement. The parameter of this function is the integer flag returned by the mysql_query function. After mysql_fetch_array is executed successfully, the record set pointer will automatically move down, so that when mysql_fetch_array is executed again, the content of the next row of records will be obtained.
The array variable $row is created by the mysql_fetch_array function and filled with the query result fields. Each component of the array corresponds to each field of the query result.
If a matching record is found, the variable $row will not be empty, and the statement in the curly braces will be executed:
do {
print $row["first_name"];
print (" ");
print $ row["last_name"];
print ("");
} while($row = mysql_fetch_array($result));
This is a do ... while loop. The difference from the while loop is that it first executes the loop body and then checks whether the loop condition is met. Since we already know that when the record set is not empty, the loop body must be executed at least once, so we should use do...while instead of while loop. What is in the curly braces is the loop body to be executed:
print $row["first_name"];
print (" ");
print $row["last_name"];
print ("");
The next step is to check whether the while condition is met. The Mysql_fetch_array function is called again to get the contents of the current record. This process keeps looping. When no next record exists, mysql_fetch_array returns false, the loop ends, and the record set is completely traversed.
The array returned by mysql_fetch_array($result) can not only be called by field name, but also can be referenced by subscripts like a normal array. In this way, the above code can also be written like this:
print $row[0];
print (" ");
print $row[1];
print ("");
We can also use echo function to write these four statements more compactly:
echo $row[0], " ", $row[1], "";
When no matching record is found, in $row There will be no content, and the else clause of the if statement will be called:
else {print "Sorry, no matching record was found in our database. ";}
The above introduces how merry lonely christmas PHP sends data to MySQL, including the content of merry lonely christmas. I hope it will be helpful to friends who are interested in PHP tutorials.
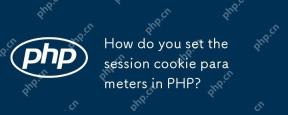
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
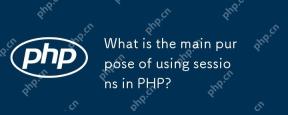
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
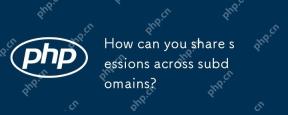
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
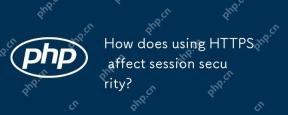
HTTPS significantly improves the security of sessions by encrypting data transmission, preventing man-in-the-middle attacks and providing authentication. 1) Encrypted data transmission: HTTPS uses SSL/TLS protocol to encrypt data to ensure that the data is not stolen or tampered during transmission. 2) Prevent man-in-the-middle attacks: Through the SSL/TLS handshake process, the client verifies the server certificate to ensure the connection legitimacy. 3) Provide authentication: HTTPS ensures that the connection is a legitimate server and protects data integrity and confidentiality.
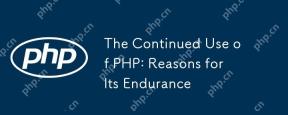
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
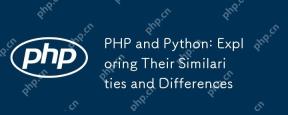
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
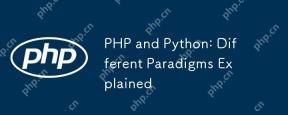
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
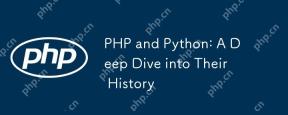
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
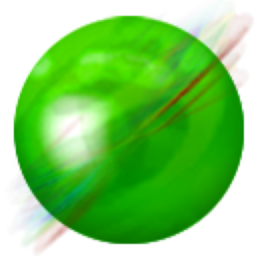
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
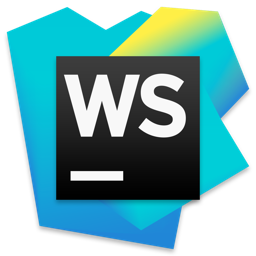
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor