


Comprehensive list of commonly used PHP codes (essential for beginners)_PHP tutorial
1. Connect the MYSQL database code
$connec=mysql_connect("localhost","root","root") or die("Cannot connect to the database server: ".mysql_error());
mysql_select_db("liuyanben",$connec) or die ("Cannot select database: ".mysql_error());
mysql_query("set names 'gbk'");
?>
2. Read the database and implement loop output
$sql="select * from liuyan order by ly_id desc";
$conn=mysql_query($sql,$connec) ;
while($rs=mysql_fetch_array($conn)){
?>
The content of the loop...
}
?>
3. How to implement paging, including two functions and two calls
1) Two functions
//Paging function
function genpage (&$sql,$page_size=2)
{
global $prepage,$nextpage,$pages,$sums; //out param
$page = $_GET["page"];
$eachpage = $page_size;
$pagesql = strstr($sql," from ");
$pagesql = "select count(*) as ids ".$pagesql;
$conn = mysql_query( $pagesql) or die(mysql_error());
if($rs = mysql_fetch_array($conn)) $sums = $rs[0];
$pages = ceil(($sums-0.5)/$ eachpage)-1;
$pages = $pages>=0?$pages:0;
$prepage = ($page>0)?$page-1:0;
$nextpage = ($ page$startpos = $page*$eachpage;
$sql .=" limit $startpos,$eachpage ";
}
/ / Show paging
function showpage()
{
global $page,$pages,$prepage,$nextpage,$queryString; //param from genpage function
$shownum =10/2;
$startpage = ($page>=$shownum)?$page-$shownum:0;
$endpage = ($page+$shownum
echo "Total".($pages+1)."Pages: ";
if($page>0)echo "Homepage a>";
if($startpage>0)
echo " ... ?";
for($i=$startpage;$i{
if($i==$ page) echo " [".($i+1)."] ";
else echo " ". ($i+1)." ";
}
if($endpageecho "? ... ";
if($pageecho "Last page";
}
//Show pagination with categories
function showpage1()
{
$fenlei =$_GET["fenleiid"];
global $page,$pages,$prepage,$nextpage,$queryString; //param from genpage function
$shownum =10/2;
$startpage = ($page>=$shownum)?$page-$shownum:0;
$endpage = ($page+$shownum
echo " Total ".($pages+1)." pages: ";
if($page>0)echo "Homepage ";
if($startpage>0)
echo " ... ?";
for($i=$startpage;$i{
if($i== $page) echo " [".($i+1)."] ";
else echo " ".($i+1)." ";
}
if($endpageecho "? ... ";
if($pageecho "Last page& amp; gt;";
}
?>
2) Two Call
First
$sql="select * from liuyan order by ly_id desc";
genpage($sql); //Just add this line to the normal code It’s OK.
$conn=mysql_query($sql,$connec);
while($rs=mysql_fetch_array($conn)){
?>
Second
}
?>
showpage(); //Show page
?>
mysql_close();
?>
4. The server side includes
5. How to write a record into the database, and then Prompt and jump to the page
$ly_title=$_POST["ly_title"];
$ly_content=$_POST["ly_content"];
$ly_time=$_POST[" ly_time"];
$ly_author=$_POST["ly_author"];
$ly_email=$_POST["ly_email"];
$sql="insert into liuyan(ly_title,ly_content,ly_time,ly_author ,ly_email) values('".$ly_title."','".$ly_content."','".$ly_time."','".$ly_author."','".$ly_email."') ";
mysql_query($sql,$connec);
echo("");
?>
6. A dialog box pops up and a page jump occurs
echo("");
?>
7. Information viewing Page (conditionally read database)
1) Conditionally read database
$sql="select * from liuyan where ly_id=$_GET[id]";
$ conn=mysql_query($sql,$connec);
$rs=mysql_fetch_array($conn);
?>
2) Output a field
=$rs[ly_title ]?>
3) Close the database
mysql_close();
?>
8. Update a record in the database and Make prompt jump
$ly_title=$_POST["ly_title"];
$ly_content=$_POST["ly_content"];
$ly_time=$_POST["ly_time "];
$ly_author=$_POST["ly_author"];
$ly_email=$_POST["ly_email"];
$sql="update liuyan set ly_title='$ly_title',ly_content= '$ly_content',ly_time='$ly_time',ly_author='$ly_author',ly_email='$ly_email' where ly_id=$_GET[id]";
mysql_query($sql,$connec);
echo("");
?>
9. How to delete a record in the database
$sql="delete from liuyan where ly_id=$_GET[id]";
mysql_query($sql,$connec);
echo("");
?>
10. How to log in as a member Verification
session_start();
$username=$_POST["username"];
$password=$_POST["password"];
$sql=" select * from admin where username='".$username."' && password='".$password."'";
$result=mysql_query($sql,$connec);
if($row =mysql_fetch_array($result)){
session_register("admin");
$admin=$username;
echo("");}
else
{
echo("& gt;");
}
mysql_close();
?>
11. How to check SESSION (production of background check page)
session_start();
if(!isset($_SESSION[" admin"])){
header("location:login.php");
exit;
}
?>
12. Verify that the username and password are filled in (javascript)
13. How to call the editor in PHP
1) Place the editor folder in the background management folder.
2) Use the following statements to perform the import operation.
Note: The name of the eWebEditorPHP38 editor folder.
The content in id=content is the name of the hidden field above
14. Loop output (can achieve column splitting)
1) First insert a row and a column table
$i=1;
?>
Other tables and outputs to be looped |
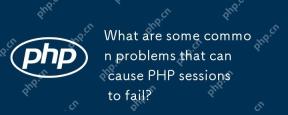
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
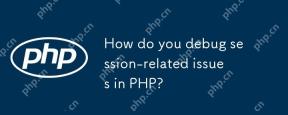
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
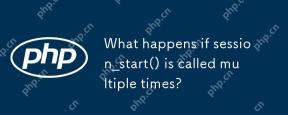
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
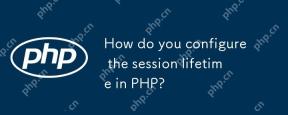
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.
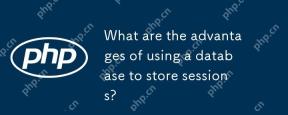
The main advantages of using database storage sessions include persistence, scalability, and security. 1. Persistence: Even if the server restarts, the session data can remain unchanged. 2. Scalability: Applicable to distributed systems, ensuring that session data is synchronized between multiple servers. 3. Security: The database provides encrypted storage to protect sensitive information.
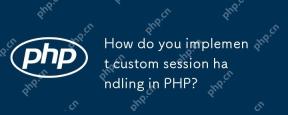
Implementing custom session processing in PHP can be done by implementing the SessionHandlerInterface interface. The specific steps include: 1) Creating a class that implements SessionHandlerInterface, such as CustomSessionHandler; 2) Rewriting methods in the interface (such as open, close, read, write, destroy, gc) to define the life cycle and storage method of session data; 3) Register a custom session processor in a PHP script and start the session. This allows data to be stored in media such as MySQL and Redis to improve performance, security and scalability.
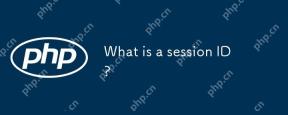
SessionID is a mechanism used in web applications to track user session status. 1. It is a randomly generated string used to maintain user's identity information during multiple interactions between the user and the server. 2. The server generates and sends it to the client through cookies or URL parameters to help identify and associate these requests in multiple requests of the user. 3. Generation usually uses random algorithms to ensure uniqueness and unpredictability. 4. In actual development, in-memory databases such as Redis can be used to store session data to improve performance and security.
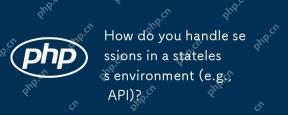
Managing sessions in stateless environments such as APIs can be achieved by using JWT or cookies. 1. JWT is suitable for statelessness and scalability, but it is large in size when it comes to big data. 2.Cookies are more traditional and easy to implement, but they need to be configured with caution to ensure security.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
