Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
introduction
In the programming world, session management of PHP is a common and important topic. Today we will discuss an interesting question: What happens if session_start()
is called multiple times? The answer to this question not only helps us understand PHP's session mechanism, but also allows us to better write efficient and stable code. Through this article, you will learn about the basic principles of session management, the consequences of multiple calls session_start()
, and how to avoid potential problems in actual projects.
Review of basic knowledge
In PHP, session is a way to store and track user data. session allows us to keep the user's status between different page requests. session_start()
is a key function to start the session. It checks whether a session already exists, and if not, a new session is created.
Core concept or function analysis
Function and definition of session_start()
The function of session_start()
function is to initialize or restore a session. After calling this function, PHP will automatically generate a unique session ID and create a session file associated with this ID on the server side to store session data.
<?php session_start(); // You can now use the $_SESSION hyperglobal variable $_SESSION['username'] = 'exampleUser'; ?>
How session_start()
works
When session_start()
is called, PHP will perform the following steps:
- Check session ID : PHP will check whether a valid session ID already exists. If it exists, it will try to read the corresponding session data from the server side.
- Create a new session : If no valid session ID is found, PHP will generate a new session ID and create a new session file on the server side.
- Register session variable : Once session is started, the
$_SESSION
hyperglobal variable will be registered, allowing us to read and write session data.
Example of usage
Basic usage
In most cases, we only need to call session_start()
once at the beginning of the script:
<?php session_start(); // You can now use $_SESSION $_SESSION['counter'] = isset($_SESSION['counter']) ? $_SESSION['counter'] 1 : 1; echo "This page has been viewed " . $_SESSION['counter'] . " times."; ?>
Advanced Usage
In some complex scenarios, we may need to call session_start()
in different parts of the script, for example, processing session data in an AJAX request:
<?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { session_start(); // Process session data $_SESSION['last_action'] = time(); } ?>
Common Errors and Debugging Tips
Multiple calls to session_start()
can cause some common problems:
- Warning message : PHP will issue a warning that the session has been started.
- Data overwrite : If
session_start()
is called multiple times at different locations, it may cause unexpected overwrite of session data.
Debugging Tips:
- Use the
session_status()
function to check the current status of the session to avoid repeated callssession_start()
.
<?php if (session_status() === PHP_SESSION_NONE) { session_start(); } ?>
Performance optimization and best practices
Multiple calls session_start()
can have a negative impact on performance, as each call involves file I/O operations. To optimize performance, we should:
- Make sure
session_start()
is called only once : callsession_start()
once at the beginning of the script, and then use$_SESSION
if needed. - Use
session_status()
: Usesession_status()
to check whether the session has been started, avoiding unnecessary calls.
In actual projects, good programming habits and best practices can help us avoid problems caused by multiple calls to session_start()
. For example, make sure that session management logic is centered in the core part of the application rather than spread out in different scripts.
In-depth thinking and suggestions
When dealing with the problem of calling session_start()
multiple times, we need to consider the following points:
- Performance impact : Multiple calls to
session_start()
will increase the burden on the server, especially in the case of high concurrency. This is because each call may involve file read and write operations, increasing I/O overhead. - Data consistency : If
session_start()
is called multiple times at different locations, it may cause inconsistency in session data, especially in multi-threaded or multi-process environments. - Debugging difficulty : Multiple calls to
session_start()
may make debugging more complicated because it may mask other potential problems.
To avoid these problems, I suggest:
- Centralized management session : Concentrate session management logic in one place to ensure
session_start()
is called only once. - Use session_status() : Use
session_status()
to check whether the session has been started, avoiding unnecessary calls. - Logging : During development, the session start and shutdown status is recorded for easy debugging and monitoring.
Through these methods, we can better manage sessions, avoid problems caused by multiple calls session_start()
, and improve code maintainability and performance.
The above is the detailed content of What happens if session_start() is called multiple times?. For more information, please follow other related articles on the PHP Chinese website!
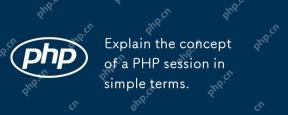
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
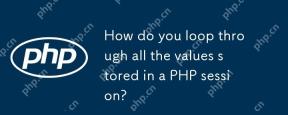
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
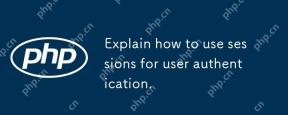
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
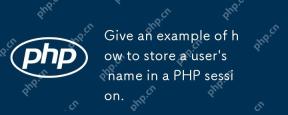
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
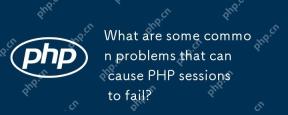
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
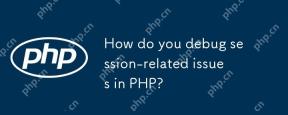
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
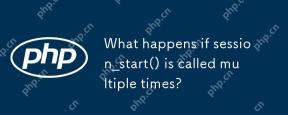
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
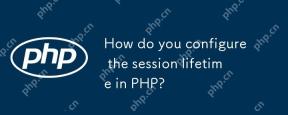
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
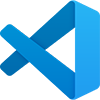
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
