


PHP tutorial: PHP singleton mode combined with command chain mode_PHP tutorial
Beginners definitely have a lot of confusion about design patterns. Today is the weekend, so I took some time to write an article about single-piece mode combined with command chain mode to create the core of the system. This may be confusing to some people. I said that the content of the article is too simple. This is a tutorial for beginners. Because time is tight (I have to go shopping with my wife, haha), there are irregularities in design, irregularity in code writing, bugs, etc. I still hope to leave it alone. The hero points it out so that everyone can make progress together. My level is limited. ^_^
I believe that everyone has read many books or articles about applying design patterns in PHP, but few of them directly give examples. Most of them feel confused after reading them. Without project practice, it is very difficult to It’s hard to figure out the design pattern part.
In order to avoid the code being too complicated, no exception handling and other contents were added.
For the basic knowledge of singleton mode and command chain mode, you can google it yourself. I won’t go into details. Let’s look at the example directly:
/**@author:NoAngels*@time:08,08 Month 30*/interface IRunAction{//Get the method defined in the class that can be run in the APPstatic function LoadActions();//The entry function in the class To call other functions in this class, use function runAction($action, $args);}/**The core part of the APP class system*/class APP{ static private $__instance = null;static private $__commands = array();static private $__flag = 1;private function __construct(){}//single piece mode Design to get the only instance of this classstatic function Load(){if(self::$__instance == null) self::$__instance = new APP;return self::$__instance;}//Add the name to $__instance of APP. Every time you add a new command, check whether an instance of this class has been added before//If so, ignore the operation, if not, add it inpublic function addCommand($cmdName){foreach(self::$__commands as $cmd){if(strtolower(get_class($cmd)) == strtolower(get_class($cmdName))){ self::$__flag = 0;break;}}if(self::$__flag == 1) self::$__commands[] = $cmdName;self::$__flag = 1;}//The core part of the command chain pattern design calls the entry function of the instance//First check whether calling this operation is allowed in the class, if not, prompt Undefined operation exitpublic function runCommand($action, $args){self::$__flag = 0;foreach(self::$__commands as $cmd){if(in_array ($action, $cmd->LoadActions())){self::$__flag = 1;$cmd->runAction($action, $args);} }if(self::$__flag == 0){self::$__flag = 1;exit("undefined action by action : $action");} }//To delete an instance of a class, just specify the name of the classpublic function removeCommand($className){foreach(self::$__commands as $key=>$cmd) {if(strtolower(get_class($cmd)) == strtolower($className)){unset(self::$__commands[$key]);}} }//For everyone to test to see if the addition and deletion are successfulpublic function viewCommands(){echo(count(self::$__commands));}}//Class User implements the interface IRunActionclass User implements IRunAction{//Define the operations that can be calledstatic private $__actions = array('addUser', 'modifyUser', 'removeUser'); //Get the callable operations. In the actual process, do not directly design $__actions as a public call//Instead, design a LoadActions function to get the value of $__actionsstatic public function LoadActions( ){return self::$__actions;}//Run the specified functionpublic function runAction($action, $args){//It’s okay to use this function if you don’t understand it Refer to the manualcall_user_func(array($this,$action), $args);}//Just a test functionprotected function addUser($name){echo($name );}}//Class Test similar to Userclass Test implements IRunAction{static private $__actions = array('addTest', 'modifyTest', 'removeTest'); static public function LoadActions(){return self::$__actions;}public function runAction($action, $args){call_user_func(array($this,$action ), $args);}protected function addTest($name){echo($name);}}//The following is the test code APP::Load()->addCommand(new User);APP::Load()->addCommand(new User);APP::Load()->addCommand(new User) ;APP::Load()->addCommand(new User);APP::Load()->runCommand('addUser', 'NoAngels');APP::Load( )->addCommand(new Test);APP::Load()->runCommand('addTest', null);
/*
*@author:NoAngels
*@time:08年08月30日
*/
interface IRunAction{
//获取类中定义的可以被APP中run的方法
static function LoadActions();
//类中的入口函数调用该类中其他函数用
function runAction($action, $args);
}
/*
*APP类系统的核心部分
*/
class APP{
static private $__instance = null;
static private $__commands = array();
static private $__flag = 1;
private function __construct(){}
//单件模式设计获取该类的唯一实例
static function Load(){
if(self::$__instance == null) self::$__instance = new APP;
return self::$__instance;
}
//添加命名到APP的$__instance中每次添加新命令的时候检查是否之前已经添加过一个该类的实例
//如果有就忽略操作如果没有就添加进来
public function addCommand($cmdName){
foreach(self::$__commands as $cmd){
if(strtolower(get_class($cmd)) == strtolower(get_class($cmdName))){
self::$__flag = 0;
break;
}
}
if(self::$__flag == 1) self::$__commands[] = $cmdName;
self::$__flag = 1;
}
//命令链模式设计的核心部分调用实例的入口函数
//首先检查是否在类中允许调用该操作如果没有就提示未定义操作退出
public function runCommand($action, $args){
self::$__flag = 0;
foreach(self::$__commands as $cmd){
if(in_array($action, $cmd->LoadActions())){
self::$__flag = 1;
$cmd->runAction($action, $args);
}
}
if(self::$__flag == 0){
self::$__flag = 1;
exit("undefined action by action : $action");
}
}
//删除某个类的实例,只要指定类的名字即可
public function removeCommand($className){
foreach(self::$__commands as $key=>$cmd){
if(strtolower(get_class($cmd)) == strtolower($className)){
unset(self::$__commands[$key]);
}
}
}
//供大家测试用看看是否添加以及删除成功
public function viewCommands(){
echo(count(self::$__commands));
}
}
//类User实现接口IRunAction
class User implements IRunAction{
//定义可以调用的操作
static private $__actions = array('addUser', 'modifyUser', 'removeUser');
//获取可以调用的操作,实际过程中不要直接就爱你个$__actions设计成public调用
//而应该设计一个LoadActions函数获取$__actions的值
static public function LoadActions(){
return self::$__actions;
}
//运行指定函数
public function runAction($action, $args){
//不明白这个函数使用的可以参看手册
call_user_func(array($this,$action), $args);
}
//测试函数而已
protected function addUser($name){
echo($name);
}
}
//类Test同类User
class Test implements IRunAction{
static private $__actions = array('addTest', 'modifyTest', 'removeTest');
static public function LoadActions(){
return self::$__actions;
}
public function runAction($action, $args){
call_user_func(array($this,$action), $args);
}
protected function addTest($name){
echo($name);
}
}
//以下是测试代码
APP::Load()->addCommand(new User);
APP::Load()->addCommand(new User);
APP::Load()->addCommand(new User);
APP::Load()->addCommand(new User);
APP::Load()->runCommand('addUser', 'NoAngels');
APP::Load()->addCommand(new Test);
APP::Load()->runCommand('addTest', null);
The APP class is designed using the singleton model, which is the core part of the system. I believe you will know by looking at the code that the Load method is to load the APP class instance, which is equivalent to the getInstance static method in some books. It has addCommand, runCommand, There are three public methods of removeCommand. runCommand is the core part. It is also the core startup program of the command chain mode. Please see the source code for the specific implementation. The code is already very clear, so I won’t go into details here.
Classes User and Test are implemented Interface IRunAction, both classes define a static private variable $__actions, which is an array that contains operations that can be called by the APP's runCommand function.
The following is the operating process of the system:
APP startup
-------addCommand, add the class to which the operation to be run belongs to the APP. If the added class is designed in singleton mode, you can add addCommand(SingletonClass:: Load()). Otherwise, you can adjust it as follows
addCommand(new someClass)
-------runCommand. Run operations. For example, there is an operation addUser in the User class. I can directly enable runCommand($acttion, $args). Loop through the APP $__commands array, if an instance of a class has the operation, call the runAction function of the instance. If you do not add an instance of a class using addCommand, it will prompt an undefined operation and exit.
In the class RunAction in User and class Test calls call_user_func, a very commonly used function. Call the corresponding function in this class.
Tips: This is the end of the explanation and examples. How you understand it and how to use this idea depends on your own understanding. Everything must be done by yourself. (ps: It can be made into a single unit in the framework. Entry file, whether to implement MVC or not depends on what you think.)
The actual operation effect is as follows:
Limited to language proficiency, if you don’t understand anything, please contact me.
I will write some articles for you when I have time.
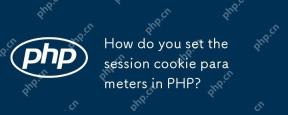
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
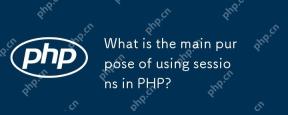
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
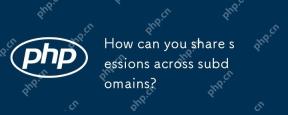
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
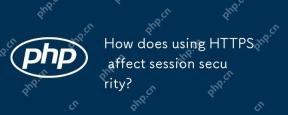
HTTPS significantly improves the security of sessions by encrypting data transmission, preventing man-in-the-middle attacks and providing authentication. 1) Encrypted data transmission: HTTPS uses SSL/TLS protocol to encrypt data to ensure that the data is not stolen or tampered during transmission. 2) Prevent man-in-the-middle attacks: Through the SSL/TLS handshake process, the client verifies the server certificate to ensure the connection legitimacy. 3) Provide authentication: HTTPS ensures that the connection is a legitimate server and protects data integrity and confidentiality.
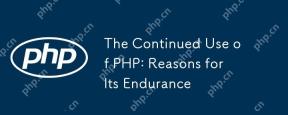
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
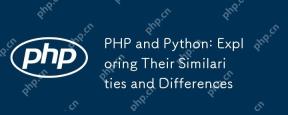
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
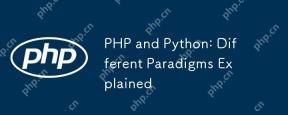
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
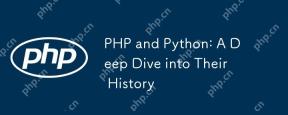
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
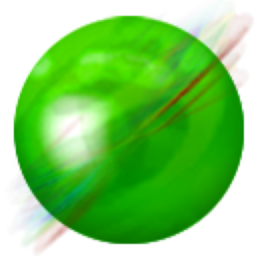
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
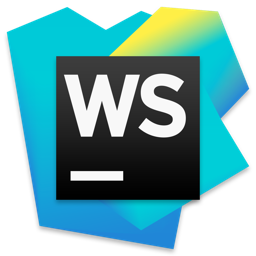
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor