


PHP design pattern series specification specification mode, specification mode_PHP tutorial
Specification specification mode of PHP design pattern series, specification mode
1. Pattern definition
The specification mode is an extension of the combination mode and is often used in framework development (rarely used in project-level development). Here is a brief introduction.
Specification mode can be considered an extension of the combination mode. Sometimes certain conditions in the project determine the business logic, and these conditions can be extracted and combined in a certain relationship (AND, OR, NOT) to flexibly customize the business logic. In addition, in applications such as query and filtering, the entire implementation logic can be simplified by predefining multiple conditions and then using a combination of these conditions to process the query or filtering instead of using logical judgment statements.
Each condition here is a specification, and multiple specifications/conditions are connected in series to form a combined specification with a certain logical relationship.
2. UML class diagram
3. Sample code
Item.php
<?php namespace DesignPatterns\Behavioral\Specification; class Item { protected $price; /** * An item must have a price * * @param int $price */ public function __construct($price) { $this->price = $price; } /** * Get the items price * * @return int */ public function getPrice() { return $this->price; } }
SpecificationInterface.php
<?php namespace DesignPatterns\Behavioral\Specification; /** * 规格接口 */ interface SpecificationInterface { /** * 判断对象是否满足规格 * * @param Item $item * * @return bool */ public function isSatisfiedBy(Item $item); /** * 创建一个逻辑与规格(AND) * * @param SpecificationInterface $spec */ public function plus(SpecificationInterface $spec); /** * 创建一个逻辑或规格(OR) * * @param SpecificationInterface $spec */ public function either(SpecificationInterface $spec); /** * 创建一个逻辑非规格(NOT) */ public function not(); }
AbstractSpecification.php
<?php namespace DesignPatterns\Behavioral\Specification; /** * 规格抽象类 */ abstract class AbstractSpecification implements SpecificationInterface { /** * 检查给定Item是否满足所有规则 * * @param Item $item * * @return bool */ abstract public function isSatisfiedBy(Item $item); /** * 创建一个新的逻辑与规格(AND) * * @param SpecificationInterface $spec * * @return SpecificationInterface */ public function plus(SpecificationInterface $spec) { return new Plus($this, $spec); } /** * 创建一个新的逻辑或组合规格(OR) * * @param SpecificationInterface $spec * * @return SpecificationInterface */ public function either(SpecificationInterface $spec) { return new Either($this, $spec); } /** * 创建一个新的逻辑非规格(NOT) * * @return SpecificationInterface */ public function not() { return new Not($this); } }
Plus.php
<?php namespace DesignPatterns\Behavioral\Specification; /** * 逻辑与规格(AND) */ class Plus extends AbstractSpecification { protected $left; protected $right; /** * 在构造函数中传入两种规格 * * @param SpecificationInterface $left * @param SpecificationInterface $right */ public function __construct(SpecificationInterface $left, SpecificationInterface $right) { $this->left = $left; $this->right = $right; } /** * 返回两种规格的逻辑与评估 * * @param Item $item * * @return bool */ public function isSatisfiedBy(Item $item) { return $this->left->isSatisfiedBy($item) && $this->right->isSatisfiedBy($item); } }
Either.php
<?php namespace DesignPatterns\Behavioral\Specification; /** * 逻辑或规格 */ class Either extends AbstractSpecification { protected $left; protected $right; /** * 两种规格的组合 * * @param SpecificationInterface $left * @param SpecificationInterface $right */ public function __construct(SpecificationInterface $left, SpecificationInterface $right) { $this->left = $left; $this->right = $right; } /** * 返回两种规格的逻辑或评估 * * @param Item $item * * @return bool */ public function isSatisfiedBy(Item $item) { return $this->left->isSatisfiedBy($item) || $this->right->isSatisfiedBy($item); } }
Not.php
<?php namespace DesignPatterns\Behavioral\Specification; /** * 逻辑非规格 */ class Not extends AbstractSpecification { protected $spec; /** * 在构造函数中传入指定规格 * * @param SpecificationInterface $spec */ public function __construct(SpecificationInterface $spec) { $this->spec = $spec; } /** * 返回规格的相反结果 * * @param Item $item * * @return bool */ public function isSatisfiedBy(Item $item) { return !$this->spec->isSatisfiedBy($item); } }
PriceSpecification.php
<?php namespace DesignPatterns\Behavioral\Specification; /** * 判断给定Item的价格是否介于最小值和最大值之间的规格 */ class PriceSpecification extends AbstractSpecification { protected $maxPrice; protected $minPrice; /** * 设置最大值 * * @param int $maxPrice */ public function setMaxPrice($maxPrice) { $this->maxPrice = $maxPrice; } /** * 设置最小值 * * @param int $minPrice */ public function setMinPrice($minPrice) { $this->minPrice = $minPrice; } /** * 判断给定Item的定价是否在最小值和最大值之间 * * @param Item $item * * @return bool */ public function isSatisfiedBy(Item $item) { if (!empty($this->maxPrice) && $item->getPrice() > $this->maxPrice) { return false; } if (!empty($this->minPrice) && $item->getPrice() < $this->minPrice) { return false; } return true; } }
4. Test code
Tests/SpecificationTest.php
<?php namespace DesignPatterns\Behavioral\Specification\Tests; use DesignPatterns\Behavioral\Specification\PriceSpecification; use DesignPatterns\Behavioral\Specification\Item; /** * SpecificationTest 用于测试规格模式 */ class SpecificationTest extends \PHPUnit_Framework_TestCase { public function testSimpleSpecification() { $item = new Item(100); $spec = new PriceSpecification(); $this->assertTrue($spec->isSatisfiedBy($item)); $spec->setMaxPrice(50); $this->assertFalse($spec->isSatisfiedBy($item)); $spec->setMaxPrice(150); $this->assertTrue($spec->isSatisfiedBy($item)); $spec->setMinPrice(101); $this->assertFalse($spec->isSatisfiedBy($item)); $spec->setMinPrice(100); $this->assertTrue($spec->isSatisfiedBy($item)); } public function testNotSpecification() { $item = new Item(100); $spec = new PriceSpecification(); $not = $spec->not(); $this->assertFalse($not->isSatisfiedBy($item)); $spec->setMaxPrice(50); $this->assertTrue($not->isSatisfiedBy($item)); $spec->setMaxPrice(150); $this->assertFalse($not->isSatisfiedBy($item)); $spec->setMinPrice(101); $this->assertTrue($not->isSatisfiedBy($item)); $spec->setMinPrice(100); $this->assertFalse($not->isSatisfiedBy($item)); } public function testPlusSpecification() { $spec1 = new PriceSpecification(); $spec2 = new PriceSpecification(); $plus = $spec1->plus($spec2); $item = new Item(100); $this->assertTrue($plus->isSatisfiedBy($item)); $spec1->setMaxPrice(150); $spec2->setMinPrice(50); $this->assertTrue($plus->isSatisfiedBy($item)); $spec1->setMaxPrice(150); $spec2->setMinPrice(101); $this->assertFalse($plus->isSatisfiedBy($item)); $spec1->setMaxPrice(99); $spec2->setMinPrice(50); $this->assertFalse($plus->isSatisfiedBy($item)); } public function testEitherSpecification() { $spec1 = new PriceSpecification(); $spec2 = new PriceSpecification(); $either = $spec1->either($spec2); $item = new Item(100); $this->assertTrue($either->isSatisfiedBy($item)); $spec1->setMaxPrice(150); $spec2->setMaxPrice(150); $this->assertTrue($either->isSatisfiedBy($item)); $spec1->setMaxPrice(150); $spec2->setMaxPrice(0); $this->assertTrue($either->isSatisfiedBy($item)); $spec1->setMaxPrice(0); $spec2->setMaxPrice(150); $this->assertTrue($either->isSatisfiedBy($item)); $spec1->setMaxPrice(99); $spec2->setMaxPrice(99); $this->assertFalse($either->isSatisfiedBy($item)); } }
The above content is the specification pattern of the PHP design pattern series shared by the editor of Bangkejia. I hope this article can help everyone.
Articles you may be interested in:
- php design pattern Composite (combination mode)
- php design pattern Template (template mode)
- php design pattern Command (command mode)
- php design mode Singleton (single case mode)
- php design mode Observer (observer mode)
- php design mode Strategy (strategy mode)
- php design mode Interpreter (interpreter mode)
- php design mode Factory (factory mode)
- php design mode Facade (appearance mode)
- php design Mode Delegation (Delegation mode)
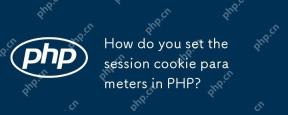
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
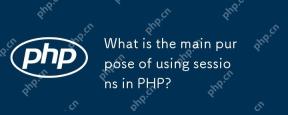
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
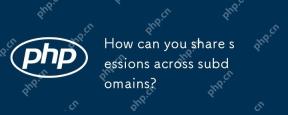
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.
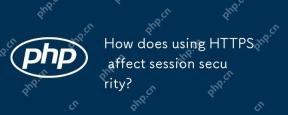
HTTPS significantly improves the security of sessions by encrypting data transmission, preventing man-in-the-middle attacks and providing authentication. 1) Encrypted data transmission: HTTPS uses SSL/TLS protocol to encrypt data to ensure that the data is not stolen or tampered during transmission. 2) Prevent man-in-the-middle attacks: Through the SSL/TLS handshake process, the client verifies the server certificate to ensure the connection legitimacy. 3) Provide authentication: HTTPS ensures that the connection is a legitimate server and protects data integrity and confidentiality.
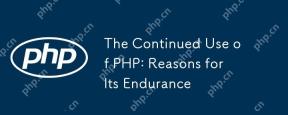
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
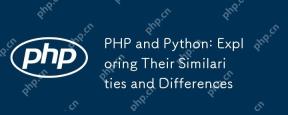
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
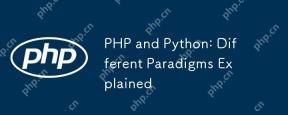
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
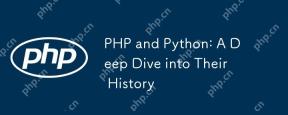
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
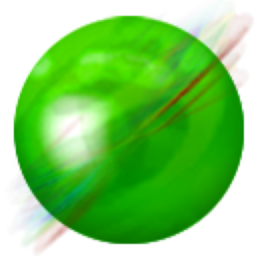
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
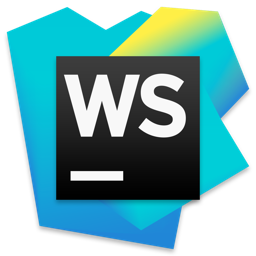
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor