How to build applications using .NET? Building applications with .NET can be achieved through the following steps: 1) Understand the basics of .NET, including C# language and cross-platform development support; 2) Learn core concepts such as components and working of the .NET ecosystem; 3) Master basic and advanced usage, from simple console applications to complex Web APIs and database operations; 4) Be familiar with common errors and debugging techniques, such as configuration and database connection issues; 5) Application performance optimization and best practices, such as asynchronous programming and caching.
introduction
When we talk about modern software development, the .NET ecosystem is undoubtedly the best among them. As an all-round development platform, .NET provides comprehensive support from desktop applications to mobile applications to cloud services. Today, I will take you into a deep dive into how to build applications with .NET, from basic to advanced, and gradually reveal the charm of this powerful platform. After reading this article, you will learn how to use the .NET ecosystem to build efficient and scalable applications, and learn some of the experience and skills I have personally accumulated during the development process.
Review of basic knowledge
.NET is a software framework developed by Microsoft that helps developers create and run applications. It includes a variety of programming languages such as C#, VB.NET, and F#, of which C# is the most commonly used. .NET provides rich class libraries and APIs, supporting cross-platform development, allowing developers to write code at once and run on multiple operating systems.
C# is a modern, object-oriented programming language that combines the power of C and C while simplifying syntax. It supports garbage collection, type safety and exception handling, allowing developers to focus more on business logic than underlying details.
Core concept or function analysis
Definition and role of .NET ecosystem
The .NET ecosystem is a comprehensive development platform that includes multiple components such as .NET Framework, .NET Core (now renamed .NET 5), ASP.NET, Xamarin, etc. Its function is to provide a unified development environment that supports multiple application types, from traditional desktop applications to modern cloud-native applications.
// C# example: Simple console application using System; <p>namespace HelloWorld { class Program { static void Main(string[] args) { Console.WriteLine("Hello, .NET World!"); } } }</p>
This simple example shows how to write a console application using C#. Through the Console.WriteLine
method, we can easily output information to the console.
How it works
The .NET ecosystem works based on the Common Language Runtime (CLR) and the Common Language Infrastructure (CLI). CLR is responsible for managing memory, handling exceptions, and executing code, while the CLI provides a set of standards that enable code written in different languages to run seamlessly on the .NET platform.
During development, the .NET compiler compiles C# code into an intermediate language (IL), and CLR then converts IL into machine code at runtime. This approach makes .NET applications cross-platform capability because ILs can be executed in CLRs on different operating systems.
Example of usage
Basic usage
Let's look at a more complex example of creating a simple Web API using ASP.NET Core.
// ASP.NET Core Web API example using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; <p>namespace MyWebApi { public class Startup { public void ConfigureServices(IServiceCollection services) { services.AddControllers(); }</p><pre class='brush:php;toolbar:false;'> public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } } public class Program { public static void Main(string[] args) { CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>(); }); }
}
This example shows how to set up an ASP.NET Core Web API project, including configuration services and middleware. With AddControllers
method, we can easily add controller support, while UseEndpoints
defines the routing of the API.
Advanced Usage
Now, let's see how to use Entity Framework Core for database operations, a more advanced usage.
// Example using Microsoft.EntityFrameworkCore; using System.Collections.Generic; using System.Linq; <p>namespace MyWebApi.Models { public class MyDbContext : DbContext { public DbSet<user> Users { get; set; }</user></p><pre class='brush:php;toolbar:false;'> protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) { optionsBuilder.UseSqlServer("Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;"); } } public class User { public int Id { get; set; } public string Name { get; set; } } public class UserService { private readonly MyDbContext _context; public UserService(MyDbContext context) { _context = context; } public List<User> GetAllUsers() { return _context.Users.ToList(); } public void AddUser(User user) { _context.Users.Add(user); _context.SaveChanges(); } }
}
In this example, we use Entity Framework Core to connect to the SQL Server database and define a User
model and a UserService
class to manage user data. With DbContext
and DbSet
, we can easily perform CRUD operations.
Common Errors and Debugging Tips
Common errors when developing with .NET include configuration issues, dependency injection errors, and database connection issues. Here are some debugging tips:
- Configuration issues : Make sure your
appsettings.json
file is configured correctly, especially connection strings and environment variables. - Dependency injection error : Check the
ConfigureServices
method in yourStartup.cs
file to make sure all services are registered correctly. - Database connection problem : Use Entity Framework Core's migration feature to manage database schemas to ensure that the database connection string is correct.
Performance optimization and best practices
Performance optimization and best practices are crucial in .NET development. Here are some suggestions:
- Asynchronous programming : Use the
async/await
keyword to write asynchronous code to improve application responsiveness and throughput.
// Asynchronous programming example public async Task <list> > GetAllUsersAsync() { return await _context.Users.ToListAsync(); } </list>
- Caching : Use in-memory cache or distributed cache to reduce the number of database queries and improve application performance.
// Cache example using Microsoft.Extensions.Caching.Memory; <p>public class UserService { private readonly IMemoryCache _cache; private readonly MyDbContext _context;</p><pre class='brush:php;toolbar:false;'> public UserService(IMemoryCache cache, MyDbContext context) { _cache = cache; _context = context; } public async Task<List<User>> GetAllUsersAsync() { string cacheKey = "allUsers"; if (!_cache.TryGetValue(cacheKey, out List<User> users)) { users = await _context.Users.ToListAsync(); var cacheEntryOptions = new MemoryCacheEntryOptions() .SetSlidingExpiration(TimeSpan.FromMinutes(5)); _cache.Set(cacheKey, users, cacheEntryOptions); } return users; }
}
Code readability : Follow the naming convention, use meaningful variable names and method names, write clear comments, and improve the maintainability of the code.
Unit Testing : Write unit tests to ensure the correctness and reliability of the code, especially when refactoring.
// Unit test example using Xunit; <p>public class UserServiceTests { [Fact] public async Task GetAllUsersAsync_ReturnsAllUsers() { // Arrange var context = new MyDbContext(); var service = new UserService(context);</p><pre class='brush:php;toolbar:false;'> // Act var users = await service.GetAllUsersAsync(); // Assert Assert.NotNull(users); Assert.NotEmpty(users); }
}
During my development career, I have found the flexibility and power of the .NET ecosystem to enable me to quickly build and deploy applications of all types. From simple console applications to complex microservice architectures, .NET provides a wealth of tools and libraries to support my development needs. I hope this article can help you better understand and utilize the .NET ecosystem and build efficient and scalable applications.
The above is the detailed content of C# .NET: Building Applications with the .NET Ecosystem. For more information, please follow other related articles on the PHP Chinese website!
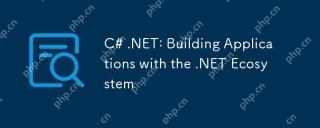
How to build applications using .NET? Building applications using .NET can be achieved through the following steps: 1) Understand the basics of .NET, including C# language and cross-platform development support; 2) Learn core concepts such as components and working principles of the .NET ecosystem; 3) Master basic and advanced usage, from simple console applications to complex WebAPIs and database operations; 4) Be familiar with common errors and debugging techniques, such as configuration and database connection issues; 5) Application performance optimization and best practices, such as asynchronous programming and caching.
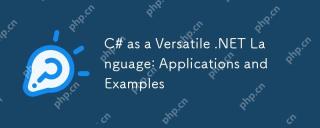
C# is widely used in enterprise-level applications, game development, mobile applications and web development. 1) In enterprise-level applications, C# is often used for ASP.NETCore to develop WebAPI. 2) In game development, C# is combined with the Unity engine to realize role control and other functions. 3) C# supports polymorphism and asynchronous programming to improve code flexibility and application performance.
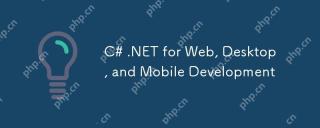
C# and .NET are suitable for web, desktop and mobile development. 1) In web development, ASP.NETCore supports cross-platform development. 2) Desktop development uses WPF and WinForms, which are suitable for different needs. 3) Mobile development realizes cross-platform applications through Xamarin.
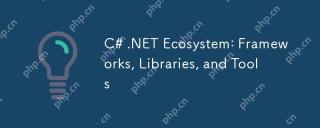
The C#.NET ecosystem provides rich frameworks and libraries to help developers build applications efficiently. 1.ASP.NETCore is used to build high-performance web applications, 2.EntityFrameworkCore is used for database operations. By understanding the use and best practices of these tools, developers can improve the quality and performance of their applications.

How to deploy a C# .NET app to Azure or AWS? The answer is to use AzureAppService and AWSElasticBeanstalk. 1. On Azure, automate deployment using AzureAppService and AzurePipelines. 2. On AWS, use Amazon ElasticBeanstalk and AWSLambda to implement deployment and serverless compute.
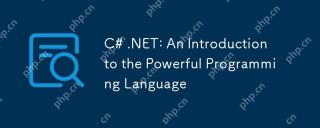
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
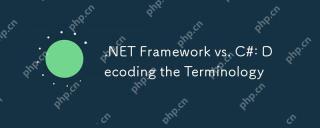
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
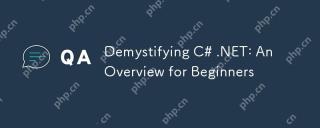
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
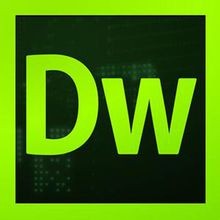
Dreamweaver CS6
Visual web development tools
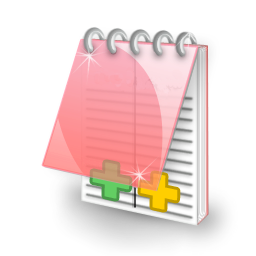
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
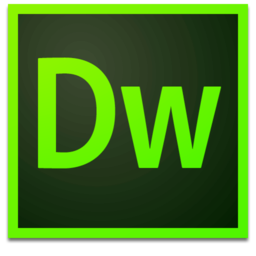
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
