“The power of the network lies in its universality. Accessible to everyone is crucial, whether disabled or not.” – Tim Berners-Lee
Accessibility is crucial in website development. As video content becomes increasingly popular, the demand for subtitle content is also growing. WebVTT is a technology that, as a subtitle format, can be easily integrated into existing Web APIs, thus solving the problem of subtitle content.
This article will discuss this. Of course, WebVTT is subtitles at the most basic level, but it can be implemented in a number of ways, making the video (and the subtitle content itself) easier to access to users.
Introduction to WebVTT format
First: WebVTT is a file type that contains the text "WebVTT" and a subtitle line with a timestamp. Examples are as follows:
<code>WEBVTT 00:00:00.000 --> 00:00:03.000 - [鸟鸣声] - 真美好的一天! 00:00:04.000 --> 00:00:07.000 - [溪流潺潺] - 的确如此! 00:00:08.000 --> 00:00:10.000 - 你好!</code>
A little weird, but it makes sense, right? As you can see, the first line is "WEBVTT", followed by the time range of the third line (0 to 3 seconds in this case). Time range is required. Otherwise, the WebVTT file simply won't work, and it won't even display or log errors to inform you. Finally, each line below the time range represents the subtitles contained within that range.
Note that you can include multiple subtitles in a single time range. A hyphen can be used to indicate the beginning of a line, but this is not necessary, it is more of a style issue.
The time range can be in one of two formats: hh:mm:ss.tt or mm:ss.tt. Each section follows certain rules:
- Hours (hh): at least two digits
- Minutes (mm): Between 00 and 59 (inclusive)
- Seconds: between 00 and 59 (inclusive)
- Milliseconds (tt): between 000 and 999 (inclusive)
At first this seemed quite daunting. You might be wondering, who can type and adjust all of this manually. Fortunately, there are tools that simplify this process. For example, YouTube can automatically add video subtitles to you using voice recognition, and also lets you download subtitles as VTT files! But that's not all. WebVTT can also be used with YouTube by uploading your VTT file to your YouTube video.
Once this file is created, we can embed it into the HTML5 video element.
<code><video autoplay="autoplay" controls="controls" height="150" width="300"><track default="" kind="captions" label="English" src="your_caption_file.vtt" srclang="en"></track></video></code>
The tag is a bit like a script that "plays" with the video. We can use multiple tracks in the same video element. The default property indicates that the track will be enabled automatically.
By the way, let's take a look at all the properties:
- srclang indicates the language of the track.
- kind represents the type of track, there are five types:
- Subtitles are usually translations and descriptions of different parts of the video.
- Describes helping users with visual impairment understand what is happening in videos.
- Subtitles provide an alternative to audio for hearing-impaired users.
- Metadata is a track used by the script and cannot be seen by the user.
- Chapters help navigate video content.
- label is the title of the text track that appears in the subtitle track
- src is the source file for the track. Unless crossorigin is specified, it cannot come from cross-origin.
Although WebVTT is designed for video, you can still place audio files by placing them<video></video>
Use it in the element with audio.
Deeply explore the structure of WebVTT files
MDN provides excellent documentation and outlines the body structure of a WebVTT file, which contains up to six components. Here is a breakdown of MDN:
- Optional byte order marking (BOM)
- String "WEBVTT"
- Optional text title to the right of WebVTT.
- WebVTT must have at least one space after it.
- You can use it to add descriptions to the file.
- You can use anything other than a newline or string "-->" in the text title.
- A blank line is equivalent to two consecutive newline characters.
- Zero or more prompts or comments.
- Zero or more blank lines.
Note: BOM is a unicode character that indicates the unicode encoding of the text file.
Bold, italic and underlined – Oh my god!
We can definitely use some inline HTML format in WebVTT files! These are all familiar to everyone: , and . You use them exactly the same way you are in HTML.
<code>WEBVTT 00:00:00.000 --> 00:00:03.000 align:start这是<b>粗体文本</b>00:00:03.000 --> 00:00:06.000 align:middle这是<i>斜体文本</i>00:00:06.000 --> 00:00:09.000 vertical:rl align:middle这是<u>下划线文本</u></code>
Prompt settings
Prompt settings are optional text strings used to control the location of subtitles. It's a bit like positioning elements in CSS, such as being able to place subtitles on video.
For example, we can place the subtitles to the right of the prompt time, control whether the subtitles are displayed horizontally or vertically, and define how the subtitles are aligned and vertically.
Here are the settings we can use.
Setting 1: Row
line controls the position of subtitles on the y-axis. If vertical is specified (we will discuss later), the line will instead indicate where the subtitles will be displayed on the x-axis.
Integers and percentages are fully acceptable units when specifying line values. In case of using integers, the distance of each row will be equal to the height of the first row (from a horizontal perspective). Therefore, for example, suppose that the height of the first line of the subtitle is equal to 50px, the specified line value is 2, and the direction of the subtitle is horizontal. This means that the subtitles will be positioned down from the top by 100px (50px times 2), equaling at most the coordinates of the video boundary. If we use a negative integer, it will move up from the bottom as the value decreases (or, if vertical:lr is specified, we will move from right to left and vice versa). Be careful here, as subtitles may be placed off-screen and are inconsistent in different browsers. The greater the ability, the greater the responsibility!
In the case of percentage, the value must be between 0-100% (inclusive) (sorry, there is no 200% giant value here). A higher value will move the subtitles from top to bottom unless vertical:lr or vertical:rl is specified, in which case the subtitles will move on the x-axis accordingly.
As the value increases, the subtitles will appear below the video boundary. As the value decreases (including negative values), the subtitles will appear above.
It's hard to imagine this without an example, right? Here is how this is converted to code:
<code>00:00:00.000 --> 00:00:03.000 line:50%此字幕应水平放置在屏幕的大致中心。</code>
<code>00:00:03.000 --> 00:00:06.000 vertical:lr line:50%此字幕应垂直放置在屏幕的大致中心。</code>
<code>00:00:06.000 --> 00:00:09.000 vertical:rl line:-1此字幕应垂直放置在视频的左侧。</code>
<code>00:00:09.000 --> 00:00:12.000 line:0字幕应水平放置在屏幕顶部。</code>
Setting 2: Vertical
vertical Indicates that the subtitles will be displayed vertically and moved in the direction specified by the line settings. Some languages do not display from left to right, but need to display from top to bottom.
<code> 00:00:00.000 --> 00:00:03.000 vertical:rl此字幕应垂直显示。</code>
<code>00:00:00.000 --> 00:00:03.000 vertical:lr此字幕应垂直显示。</code>
Setting 3: Position
position Specifies the position where the subtitles will be displayed on the x-axis. If vertical is specified, position will instead specify the position where the subtitles will be displayed on the y-axis. It must be an integer between 0% and 100% (inclusive).
<code>00:00:00.000 --> 00:00:03.000 vertical:rl position:100%此字幕将垂直显示并在底部。 00:00:03.000 --> 00:00:06.000 vertical:rl position:0%此字幕将垂直显示并在顶部。</code>
At this point, you may notice that line and position are similar to CSS flexbox properties align-items and justify-content, while vertical is very similar to flex-direction. One trick to remember the WebVTT direction is that line specifies a position perpendicular to the text stream, and position specifies a position parallel to the text stream. This is why if we specify vertical, line will suddenly move along the horizontal axis and position will move along the vertical axis.
Setting 4: Size
size Specifies the width of the subtitle. If vertical is specified, it will instead set the height of the subtitles. Like other settings, it must be an integer between 0% and 100% (inclusive).
<code>00:00:00.000 --> 00:00:03.000 vertical:rl size:50%此字幕将垂直填充屏幕的一半。</code>
<code>00:00:03.000 --> 00:00:06.000 position:0%此字幕将水平填充整个屏幕。</code>
Setting 5: Align
align Specifies where the text will appear in the horizontal direction. If vertical is specified, it will instead control vertical alignment.
The values we have are: start, middle, end, left, and right. If no vertical is specified, the alignment is exactly what they sound like. If vertical is specified, they will actually become top, middle (vertical), and bottom. Using start and end instead of left and right is a more flexible way to allow alignment of plain text values based on unicode-bidi CSS attributes.
Note that align is not affected by vertical:lr or vertical:rl .
<code>WEBVTT 00:00:00.000 --> 00:00:03.000 align:start此字幕将出现在屏幕左侧。 00:00:03.000 --> 00:00:06.000 align:middle此字幕将水平位于屏幕中央。 00:00:06.000 --> 00:00:09.000 vertical:rl align:middle此字幕将垂直位于屏幕中央。 00:00:09.000 --> 00:00:12.000 vertical:rl align:end此字幕将垂直位于屏幕的右下角,而不管vertical:lr 或vertical:rl 的方向如何。 00:00:12.000 --> 00:00:15.000 vertical:lr align:end此字幕将垂直位于屏幕底部,而不管vertical:lr 或vertical:rl 的方向如何。 00:00:12.000 --> 00:00:15.000 align:left此字幕将出现在屏幕左侧。 00:00:12.000 --> 00:00:15.000 align:right此字幕将出现在屏幕右侧。</code>
WebVTT Comments
WebVTT comments are text strings that are only visible when reading file source text, just like the comments we think of in HTML, CSS, JavaScript, and any other language. Comments can contain line breaks, but not empty lines (essentially two-line newlines).
<code>WEBVTT 00:00:00.000 --> 00:00:03.000 - [鸟鸣声] - 真美好的一天! NOTE 这是一个注释。观看字幕的任何人都不会看到它。 00:00:04.000 --> 00:00:07.000 - [溪流潺潺] - 的确如此! 00:00:08.000 --> 00:00:10.000 - 你好!</code>
When parsing and rendering the subtitle file, the highlighted lines above will be completely hidden in front of the user. Comments can also be multi-line.
There are three very important characters/strings to be noted and they cannot be used for comments:. As an alternative, you can use escape characters.
Some other interesting WebVTT features
We'll get a quick look at some very clever ways to customize and control subtitles, but at least at the time of writing, these methods lack consistent browser support.
Yes, we can style the subtitles!
In fact, WebVTT subtitles can be styled. For example, to set the background of the subtitle to red, set the background property on the ::cue pseudo-element:
<code>video::cue { background: red; }</code>
Remember we can use some inline HTML format in WebVTT files? Well, we can choose them, too. For example, select the italic ( ) element:
<code>video::cue(i) { color: yellow; }</code>
It turns out that WebVTT files support style blocks, which is very similar to HTML files:
<code>WEBVTT STYLE ::cue { color: blue; font-family: "Source Sans Pro", sans-serif; }</code>
Elements can also be accessed through their prompt identifier. Note that prompt identifiers use the same escape mechanism as HTML.
<code>WEBVTT STYLE ::cue(#middle\ cue\ identifier) { text-decoration: underline; } ::cue(#cue\ identifier\ \33) { font-weight: bold; color: red; } first cue identifier 00:00:00.000 --> 00:00:02.000你好,世界! middle cue identifier 00:00:02.000 --> 00:00:04.000此提示标识符将带有下划线! cue identifier 3 00:00:04.000 --> 00:00:06.000这个不会受到影响,就像第一个一样!</code>
Different types of tags
Many tags can be used to format subtitles. There is a warning. These tags cannot be used in elements with a kind attribute chapters. Here are some formatted tags you can use.
class tags
We can use the class tag to define classes in WebVTT tags, and we can use CSS to select these classes. Suppose we have a class .yellowish which turns the text yellow. We can use this tag in subtitles. We can control many styles in this way, such as fonts, font colors and background colors.
<code>/* 我们的CSS 文件*/ .yellowish { color: yellow; } .redcolor { color: red; }</code>
<code>WEBVTT 00:00:00.000 --> 00:00:03.000此文本应为黄色。此文本将为默认颜色。 00:00:03.000 --> 00:00:06.000此文本应为红色。此文本将为默认颜色。</code>
Timestamp tag
If you want to make subtitles appear at a specific time, you need to use a timestamp tag. They are like fine-tuning subtitles to exact points in time. The time of the tag must be within a given time range of the subtitles, and each timestamp tag must be later than the previous one.
<code>WEBVTT 00:00:00.000 --> 00:00:07.000此文本将显示超过6 秒。</code>
voice tag
voice tags are concise because they help identify who is talking.
<code>WEBVTT 00:00:00.000 --> 00:00:03.000鲍勃,你今天过得怎么样? 00:00:03.000 --> 00:00:06.000很好,你呢?</code>
ruby tags
Ruby tags are a way to display small comment characters above subtitles.
<code>WEBVTT 00:00:00.000 --> 00:00:05.000</code> This subtitle will have text displayed above it<rt> This text will be displayed above the subtitles.</rt>
in conclusion
That’s all about WebVTT! This is a very useful technology that provides an opportunity to greatly improve accessibility of your website, especially if you are using videos. Try writing some subtitles yourself to get a better idea of it!
The above is the detailed content of Improving Video Accessibility with WebVTT. For more information, please follow other related articles on the PHP Chinese website!
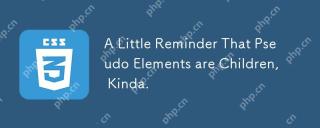
Here's a container with some child elements:
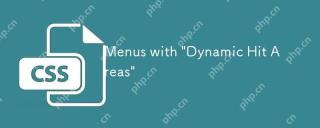
Flyout menus! The second you need to implement a menu that uses a hover event to display more menu items, you're in tricky territory. For one, they should
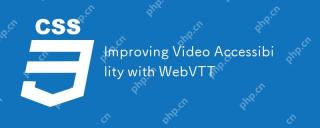
"The power of the Web is in its universality. Access by everyone regardless of disability is an essential aspect."- Tim Berners-Lee
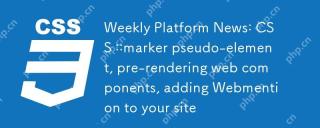
In this week's roundup: datepickers are giving keyboard users headaches, a new web component compiler that helps fight FOUC, we finally get our hands on styling list item markers, and four steps to getting webmentions on your site.
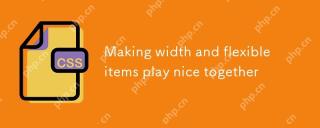
The short answer: flex-shrink and flex-basis are probably what you’re lookin’ for.
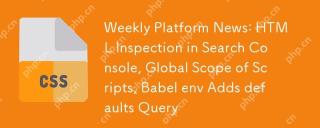
In this week's look around the world of web platform news, Google Search Console makes it easier to view crawled markup, we learn that custom properties
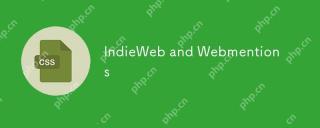
The IndieWeb is a thing! They've got a conference coming up and everything. The New Yorker is even writing about it:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
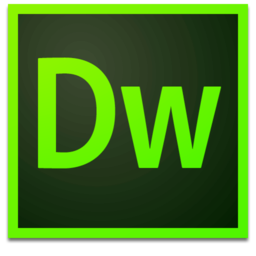
Dreamweaver Mac version
Visual web development tools
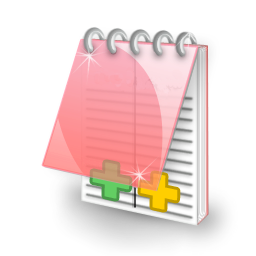
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor