Laravel's Versatility: From Simple Sites to Complex Systems
The Laravel development project was chosen because of its flexibility and power to suit the needs of different sizes and complexities. Laravel provides routing system, Eloquent ORM, Artisan command line and other functions, supporting the development of from simple blogs to complex enterprise-level systems.
introduction
You ask me why I chose Laravel to develop projects from simple websites to complex systems? The answer is simple: Laravel's flexibility and power have allowed me to cope with project needs of all sizes and complexities. This article will take you into the versatility of Laravel, how to start with a simple blog website and gradually expand to complex enterprise-level application systems. After reading this article, you will learn how to use the features of Laravel to build different types of projects and master some tips and best practices that are very useful in actual development.
Laravel's basics
Laravel is an open source framework based on PHP, known for its elegant syntax and rich features. Its design philosophy is to enable developers to build elegant web applications faster. Before using Laravel, it is necessary to understand some basic PHP knowledge, such as variables, functions, classes, and objects. Laravel also provides a powerful ORM (Object Relational Mapping) tool Eloquent, making database operations very simple and intuitive.
Analysis of the core functions of Laravel
Router and Controller
Laravel's routing system is very powerful. It allows you to define the URL structure of your application and map these URLs to the corresponding controller method. This makes your application logic clearer and easier to manage.
// Define a simple route Route::get('/', function () { return view('welcome'); }); // Use controller Route::get('/users', [UserController::class, 'index']);
The routing system can not only handle simple GET requests, but also handle various HTTP methods such as POST, PUT, DELETE, etc. You can use controllers to organize your code logic to make your application structure clearer.
Eloquent ORM
Eloquent ORM is a highlight of Laravel, which provides a simple and elegant way to interact with the database. You can define models to represent database tables, and use these models to add, delete, modify and search data.
// User model class User extends Model { protected $fillable = ['name', 'email', 'password']; } // Get all users $users = User::all(); // Create a new user $user = User::create([ 'name' => 'John Doe', 'email' => 'john@example.com', 'password' => bcrypt('password') ]);
Eloquent can not only handle basic CRUD operations, but also supports complex query and relationship management, such as one-to-one, one-to-many, many-to-many and other relationships.
Art Command Line
Laravel's Artisan is a powerful tool that can help you generate code, manage database migrations, run tests, and more. With Artisan, you can quickly generate controllers, models, migration files, etc., thereby improving development efficiency.
# Generate a new controller php artisan make:controller UserController # Run database migration php artisan migrate
The Artisan command line tool not only helps you quickly generate code, but also allows you to customize commands to meet specific needs.
Example of usage
Build a simple blog site
Let's start with a simple blog site. With Laravel, you can quickly build a blog with basic functions, including the creation, editing and deletion of articles.
// Article model class Post extends Model { protected $fillable = ['title', 'content']; public function user() { return $this->belongsTo(User::class); } } // Article Controller class PostController extends Controller { public function index() { $posts = Post::with('user')->latest()->paginate(10); return view('posts.index', compact('posts')); } public function create() { return view('posts.create'); } public function store(Request $request) { $post = auth()->user()->posts()->create($request->all()); return redirect()->route('posts.show', $post); } }
This simple blog site shows the basic use of Laravel, including the combination of models, controllers, and views. As you can see, Laravel makes the code very concise and easy to understand.
Extend to complex enterprise-level systems
Laravel is still competent when your project needs become more complex. Suppose you need to build an enterprise-level CRM system, you can use Laravel's queue system to handle asynchronous tasks and use event listeners to implement complex business logic.
// Define a queue task class SendWelcomeEmail implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; protected $user; public function __construct(User $user) { $this->user = $user; } public function handle() { Mail::to($this->user->email)->send(new WelcomeEmail($this->user)); } } // Trigger queue task when user registration Event::listen('user.registered', function ($user) { SendWelcomeEmail::dispatch($user); });
Through the queue system, you can hand over time-consuming tasks to the background to process, thereby improving the application's response speed and user experience. Event listeners can help you implement complex business logic, such as sending welcome emails when users register.
FAQs and debugging tips
During the development process using Laravel, you may encounter some common problems, such as migration failures, routing conflicts, etc. Here are some debugging tips:
- Migration failed : Check your migration file for syntax errors and make sure the database connection is configured correctly. You can use
php artisan migrate:rollback
command to roll back the migration and then rerunphp artisan migrate
. - Routing conflict : Check whether your route definition is duplicated and make sure that the URI and method of each route are unique. You can use
php artisan route:list
command to view all defined routes.
Performance optimization and best practices
Performance optimization and best practices are very important in real projects. Laravel provides a variety of ways to optimize your application performance.
- Caching : Use Laravel's cache system to cache frequently accessed data to reduce the number of database queries.
// Cache query result $posts = Cache::remember('posts', 3600, function () { return Post::with('user')->latest()->paginate(10); });
- Database query optimization : Avoid N 1 query problems and use Eloquent's
with
method to preload the associated data.
// Preload user data $posts = Post::with('user')->latest()->paginate(10);
- Code readability and maintenance : Keep the code neat and readable, using comments and documentation to explain complex logic. Follow Laravel's naming conventions and code style guide.
// Good code comments/** * Fetch and paginate the latest posts with their associated users. * * @return \Illuminate\Pagination\LengthAwarePaginator */ public function index() { $posts = Post::with('user')->latest()->paginate(10); return view('posts.index', compact('posts')); }
My experience when using Laravel development projects tells me that it is very important to keep the code concise and maintainable. By using Laravel's various functions properly, you can build applications that are both efficient and easy to maintain. I hope this article will help you better understand Laravel's versatility and flexibly apply this knowledge in actual projects.
The above is the detailed content of Laravel's Versatility: From Simple Sites to Complex Systems. For more information, please follow other related articles on the PHP Chinese website!

React,Vue,andAngularcanbeintegratedwithLaravelbyfollowingspecificsetupsteps.1)ForReact:InstallReactusingLaravelUI,setupcomponentsinapp.js.2)ForVue:UseLaravel'sbuilt-inVuesupport,configureinapp.js.3)ForAngular:SetupAngularseparately,servethroughLarave

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.

ToscaleaLaravelapplicationeffectively,focusondatabasesharding,caching,loadbalancing,andmicroservices.1)Implementdatabaseshardingtodistributedataacrossmultipledatabasesforimprovedperformance.2)UseLaravel'scachingsystemwithRedisorMemcachedtoreducedatab

Toovercomecommunicationbarriersindistributedteams,use:1)videocallsforface-to-faceinteraction,2)setclearresponsetimeexpectations,3)chooseappropriatecommunicationtools,4)createateamcommunicationguide,and5)establishpersonalboundariestopreventburnout.The

LaravelBladeenhancesfrontendtemplatinginfull-stackprojectsbyofferingcleansyntaxandpowerfulfeatures.1)Itallowsforeasyvariabledisplayandcontrolstructures.2)Bladesupportscreatingandreusingcomponents,aidinginmanagingcomplexUIs.3)Itefficientlyhandleslayou

Laravelisidealforfull-stackapplicationsduetoitselegantsyntax,comprehensiveecosystem,andpowerfulfeatures.1)UseEloquentORMforintuitivebackenddatamanipulation,butavoidN 1queryissues.2)EmployBladetemplatingforcleanfrontendviews,beingcautiousofoverusing@i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
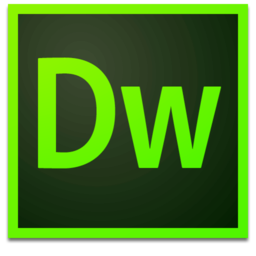
Dreamweaver Mac version
Visual web development tools
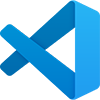
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
