


How do you handle scalability challenges in Python applications?
Handling scalability challenges in Python applications involves a multi-faceted approach that addresses both the code and the infrastructure. Here are some key strategies:
- Code Optimization: Ensuring that the Python code is efficient and optimized is crucial. This includes using appropriate data structures, minimizing loops, and leveraging built-in functions and libraries that are optimized for performance.
-
Caching: Implementing caching mechanisms can significantly reduce the load on your application by storing the results of expensive operations and reusing them when needed. Python offers various caching libraries like
dogpile.cache
andRedis
. - Database Optimization: Efficient database queries and indexing can greatly improve the performance of your application. Consider using ORM tools like SQLAlchemy with care to avoid generating inefficient queries.
- Load Balancing: Distributing incoming requests across multiple servers can help manage high traffic. Tools like Nginx can be used to set up load balancing.
-
Asynchronous Programming: Using asynchronous programming models, such as those provided by
asyncio
, can help handle a large number of concurrent connections more efficiently. - Horizontal Scaling: Adding more machines to your infrastructure to handle increased load. This can be facilitated by containerization technologies like Docker and orchestration tools like Kubernetes.
- Microservices Architecture: Breaking down your application into smaller, independent services can improve scalability by allowing each service to scale independently based on demand.
By implementing these strategies, you can effectively manage and improve the scalability of your Python applications.
What are the best practices for optimizing Python code to improve scalability?
Optimizing Python code for scalability involves several best practices that can significantly enhance the performance and efficiency of your applications. Here are some key practices:
- Use Appropriate Data Structures: Choose the right data structure for your task. For example, use sets for membership testing and dictionaries for fast lookups.
-
Avoid Unnecessary Loops: Minimize the use of loops where possible. Use list comprehensions, generator expressions, or built-in functions like
map()
,filter()
, andreduce()
to process data more efficiently. - Leverage Built-in Functions and Libraries: Python's built-in functions and standard libraries are often optimized for performance. Use them instead of writing custom implementations.
-
Profile Your Code: Use profiling tools like
cProfile
orline_profiler
to identify bottlenecks in your code. This helps you focus your optimization efforts on the parts of the code that need it most. - Use Cython or Numba for Performance-Critical Code: For computationally intensive parts of your code, consider using Cython or Numba to compile Python code to C, which can significantly improve performance.
-
Implement Caching: Use caching to store the results of expensive operations. Libraries like
functools.lru_cache
can be used for simple caching needs. - Optimize Database Queries: Ensure that your database queries are efficient. Use indexing, avoid N 1 query problems, and consider using database-specific optimizations.
-
Asynchronous Programming: Use asynchronous programming techniques to handle I/O-bound operations more efficiently. Libraries like
asyncio
can help manage concurrent operations without blocking.
By following these best practices, you can optimize your Python code to improve its scalability and performance.
How can asynchronous programming in Python help with handling high loads?
Asynchronous programming in Python can significantly help with handling high loads by allowing your application to manage multiple tasks concurrently without blocking. Here's how it works and its benefits:
- Non-Blocking I/O Operations: Asynchronous programming allows your application to perform I/O operations (like reading from a database or making an API call) without waiting for the operation to complete. This means your application can continue processing other tasks while waiting for I/O operations to finish.
- Efficient Resource Utilization: By not blocking on I/O operations, asynchronous programming allows your application to make better use of system resources. This is particularly beneficial for handling a large number of concurrent connections, as it can keep more connections active at the same time.
- Scalability: Asynchronous programming can help your application scale more efficiently. Since it can handle more concurrent operations with fewer resources, you can serve more users without needing to add more hardware.
- Improved Responsiveness: Applications using asynchronous programming tend to be more responsive, as they can quickly switch between tasks and handle user requests more efficiently.
-
Use of
asyncio
: Python'sasyncio
library provides a robust framework for writing asynchronous code. It allows you to define coroutines usingasync
andawait
keywords, making it easier to write and maintain asynchronous code. -
Asynchronous Web Frameworks: Frameworks like
aiohttp
andFastAPI
leverage asynchronous programming to build scalable web applications. These frameworks can handle a high number of concurrent requests efficiently.
By leveraging asynchronous programming, you can build Python applications that are better equipped to handle high loads and scale more effectively.
What tools and frameworks can be used to monitor and manage scalability in Python applications?
Monitoring and managing scalability in Python applications requires the use of various tools and frameworks that can help you track performance, identify bottlenecks, and scale your application effectively. Here are some key tools and frameworks:
-
Monitoring Tools:
- Prometheus: An open-source monitoring and alerting toolkit that can be used to collect metrics from your Python applications. It integrates well with Grafana for visualization.
- New Relic: A comprehensive monitoring tool that provides detailed insights into your application's performance, including response times, throughput, and error rates.
- Datadog: Offers real-time monitoring and analytics for your applications, with support for custom metrics and dashboards.
-
Logging and Tracing:
- ELK Stack (Elasticsearch, Logstash, Kibana): A powerful combination for log analysis and visualization. It can help you track and analyze logs from your Python applications.
- Jaeger: An open-source, end-to-end distributed tracing system that can help you understand the flow of requests through your application and identify performance bottlenecks.
-
Profiling Tools:
- cProfile: A built-in Python profiler that can help you identify which parts of your code are consuming the most time.
- line_profiler: A more detailed profiler that can show you the time spent on each line of your code.
-
Load Testing Tools:
- Locust: An open-source load testing tool that allows you to define user behavior in Python code and simulate thousands of concurrent users.
- Apache JMeter: A popular open-source tool for load testing and performance measurement.
-
Containerization and Orchestration:
- Docker: Allows you to containerize your Python applications, making it easier to deploy and scale them.
- Kubernetes: An orchestration platform that can manage the deployment, scaling, and operation of containerized applications.
-
Scalability Frameworks:
- Celery: A distributed task queue that can help you offload and manage background tasks, improving the scalability of your application.
- Gunicorn: A WSGI HTTP server for Unix that can be used to run Python web applications, with support for multiple workers to handle concurrent requests.
By using these tools and frameworks, you can effectively monitor and manage the scalability of your Python applications, ensuring they can handle increased loads and perform optimally.
The above is the detailed content of How do you handle scalability challenges in Python applications?. For more information, please follow other related articles on the PHP Chinese website!
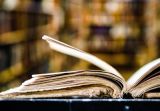
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
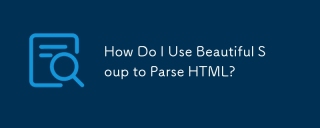
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
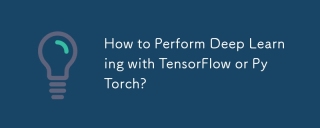
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
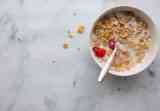
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
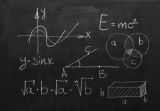
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
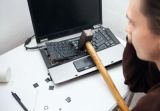
In this tutorial you'll learn how to handle error conditions in Python from a whole system point of view. Error handling is a critical aspect of design, and it crosses from the lowest levels (sometimes the hardware) all the way to the end users. If y
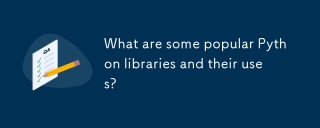
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
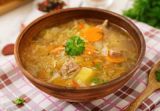
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
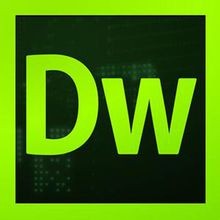
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
