


What are the benefits of using logging?
Logging is a crucial aspect of software development that offers numerous benefits, enhancing the overall quality and maintainability of applications. Here are some key advantages of using logging:
- Debugging and Troubleshooting: Logging provides a detailed record of the application's execution, which is invaluable for identifying and resolving issues. By examining log files, developers can trace the sequence of events leading up to an error, making it easier to pinpoint the root cause of problems.
- Monitoring and Performance Analysis: Logs can be used to monitor the health and performance of an application. By analyzing log data, developers and system administrators can identify bottlenecks, track resource usage, and optimize the application's performance.
- Auditing and Compliance: In many industries, logging is essential for meeting regulatory requirements. Logs can serve as an audit trail, documenting user actions, system changes, and other critical events that need to be tracked for compliance purposes.
- Error Tracking and Notification: Logging can be configured to send notifications when specific events occur, such as errors or critical system failures. This allows for proactive monitoring and faster response times to issues that could impact the application's availability or performance.
- Historical Data and Analysis: Logs provide a historical record of the application's behavior over time. This data can be analyzed to identify trends, understand user behavior, and make informed decisions about future development and improvements.
- Flexibility and Customization: Logging frameworks are highly customizable, allowing developers to tailor the logging output to their specific needs. This includes setting different log levels (e.g., debug, info, warning, error), filtering log messages, and directing logs to various outputs (e.g., files, console, remote servers).
What are some best practices for implementing logging in Python applications?
Implementing logging effectively in Python applications involves following several best practices to ensure that logs are useful, manageable, and do not negatively impact the application's performance. Here are some key best practices:
-
Use the Built-in
logging
Module: Python'slogging
module is a powerful and flexible tool for logging. It is recommended to use this module instead of custom solutions or print statements, as it provides a standardized way to handle logs. - Configure Logging Early: Configure logging at the beginning of your application. This ensures that all parts of the application can use the logging system from the start. You can configure logging in a separate configuration file or directly in your code.
- Set Appropriate Log Levels: Use different log levels (DEBUG, INFO, WARNING, ERROR, CRITICAL) to categorize log messages based on their importance. This allows you to filter and manage logs more effectively.
- Use Descriptive Log Messages: Ensure that log messages are clear and descriptive. Include relevant context such as user IDs, transaction IDs, or other identifiers that can help in tracing issues.
-
Log Exceptions Properly: When logging exceptions, use the
logging.exception()
method or pass the exception as an argument tologging.error()
. This ensures that the full stack trace is included in the log, which is crucial for debugging. - Avoid Excessive Logging: Be mindful of the volume of logs generated. Excessive logging can lead to performance issues and make it harder to find relevant information. Log only what is necessary and useful.
- Use Structured Logging: Consider using structured logging formats like JSON, which make it easier to parse and analyze log data programmatically.
- Centralize Log Management: For larger applications, consider centralizing log management using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or other log aggregation solutions. This allows for easier monitoring and analysis of logs across multiple servers or services.
How can logging improve the debugging and maintenance of Python code?
Logging plays a critical role in improving the debugging and maintenance of Python code by providing several key benefits:
- Detailed Error Tracking: Logging allows developers to capture detailed information about errors, including the full stack trace, the state of the application at the time of the error, and any relevant context. This makes it easier to diagnose and fix issues.
- Historical Context: Logs provide a historical record of the application's behavior, which is invaluable for understanding how issues developed over time. This historical context can help developers identify patterns or recurring issues that need to be addressed.
- Reduced Debugging Time: With comprehensive logs, developers can quickly identify the sequence of events leading up to an error. This reduces the time spent on debugging, as developers can focus on the relevant parts of the code rather than guessing where the problem might be.
- Improved Code Maintenance: Logging helps in maintaining code by providing insights into how different parts of the application interact. This can be particularly useful when refactoring or adding new features, as developers can use logs to ensure that changes do not introduce unintended side effects.
- Proactive Issue Detection: By monitoring logs, developers can detect issues before they become critical. For example, logs can reveal performance degradation or unusual patterns of behavior that might indicate an impending problem.
- Enhanced Collaboration: Logs can serve as a communication tool among team members. When multiple developers are working on a project, logs provide a common reference point for discussing issues and solutions.
How can you implement effective logging strategies in Python?
Implementing effective logging strategies in Python involves a combination of using the right tools, following best practices, and tailoring the logging system to the specific needs of your application. Here are some steps to implement effective logging:
-
Configure the Logging System: Start by configuring the logging system using the
logging
module. You can do this in a configuration file or directly in your code. Here's an example of basic configuration:import logging logging.basicConfig( level=logging.INFO, format='%(asctime)s - %(name)s - %(levelname)s - %(message)s', filename='app.log', filemode='a' )
-
Use Loggers: Create loggers for different parts of your application. This allows you to control logging at a more granular level. For example:
logger = logging.getLogger(__name__) logger.info('This is an info message')
-
Implement Log Levels: Use different log levels to categorize messages based on their importance. This helps in filtering logs and focusing on critical issues:
logger.debug('This is a debug message') logger.info('This is an info message') logger.warning('This is a warning message') logger.error('This is an error message') logger.critical('This is a critical message')
-
Log Exceptions: When an exception occurs, use
logging.exception()
to log the full stack trace:try: # Some code that might raise an exception result = 10 / 0 except ZeroDivisionError: logger.exception('Division by zero occurred')
-
Use Structured Logging: Consider using structured logging formats like JSON to make log analysis easier. You can use libraries like
python-json-logger
to achieve this:import logging from pythonjsonlogger import jsonlogger logger = logging.getLogger(__name__) logHandler = logging.StreamHandler() formatter = jsonlogger.JsonFormatter() logHandler.setFormatter(formatter) logger.addHandler(logHandler) logger.info('This is an info message')
-
Centralize Log Management: For larger applications, consider using centralized log management solutions like ELK Stack. This involves setting up log forwarding to a centralized server where logs can be aggregated and analyzed:
import logging import logging.handlers logger = logging.getLogger(__name__) handler = logging.handlers.SysLogHandler(address=('logserver', 514)) logger.addHandler(handler) logger.info('This is an info message')
By following these steps and best practices, you can implement an effective logging strategy that enhances the debugging, monitoring, and maintenance of your Python applications.
The above is the detailed content of What are the benefits of using logging? How can you implement effective logging strategies in Python?. For more information, please follow other related articles on the PHP Chinese website!
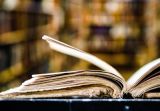
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
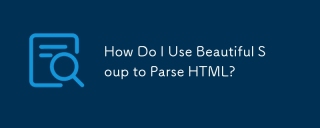
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
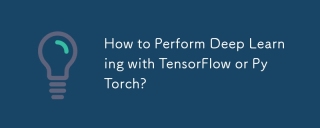
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
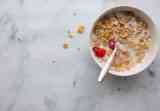
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
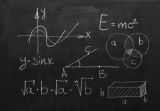
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
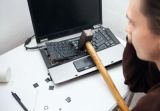
In this tutorial you'll learn how to handle error conditions in Python from a whole system point of view. Error handling is a critical aspect of design, and it crosses from the lowest levels (sometimes the hardware) all the way to the end users. If y
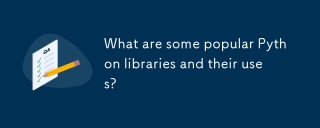
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
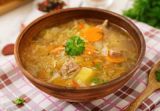
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
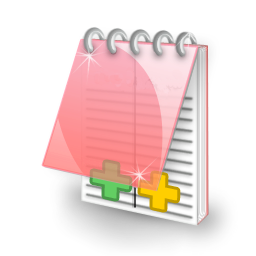
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
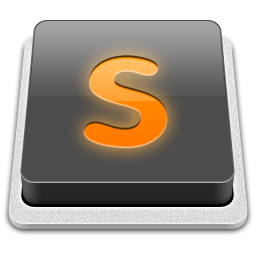
SublimeText3 Mac version
God-level code editing software (SublimeText3)
