


How do I use profiling tools like pprof to identify performance bottlenecks in Go?
This article explains using Go's pprof for performance analysis. It details profiling steps (instrumentation, profiling, analysis) and interpreting results from various views (top, flat, call graph). Common pitfalls like insufficient warm-up and mi
How to Use pprof to Identify Performance Bottlenecks in Go
Profiling with pprof
is a powerful technique for identifying performance bottlenecks in Go applications. The process generally involves three main steps: instrumenting your code, running your application under profiling, and then analyzing the profile data.
1. Instrumentation: You need to enable profiling in your Go application. This is typically done using the net/http/pprof
package. Include this package in your code and then start the profiling server:
import ( "log" "net/http" _ "net/http/pprof" // Import the pprof package ) func main() { // ... your application code ... log.Println("Starting pprof server on :6060") log.Fatal(http.ListenAndServe(":6060", nil)) }
This starts a simple HTTP server on port 6060 exposing various profiling endpoints.
2. Running the Profile: Run your application with a representative workload. While your application is running, you can then use your browser or command line tools to access the profile data. For example, to get a CPU profile, navigate to http://localhost:6060/debug/pprof/profile
in your browser. This will download a profile file (usually a pprof
file). For other types of profiles (like memory profiles), use different endpoints (e.g., /debug/pprof/heap
for heap profiles). You can also use the go tool pprof
command directly to generate profiles without using the web interface.
3. Analyzing the Profile: Once you have the profile file, use the go tool pprof
command to analyze it. For example:
go tool pprof -http=:8080 profile.pprof
This will open a web interface that allows you to visualize the profile data. You can navigate through different views (e.g., call graph, top, flat) to identify functions consuming the most CPU time or memory. The "top" view is often a good starting point, showing the functions consuming the most resources. The call graph provides a visual representation of the call stack and allows you to identify bottlenecks in the context of the application's execution flow.
Common Pitfalls to Avoid When Using pprof for Go Performance Analysis
Several common pitfalls can lead to inaccurate or misleading results when using pprof
for Go performance analysis:
- Insufficient Warm-up: Don't start profiling immediately after launching your application. Allow sufficient time for the application to warm up and reach a steady state. Initial startup overhead can skew the results.
- Unrepresentative Workload: Profile your application under a workload that accurately reflects its typical usage. Using a trivial or unrealistic workload can lead to inaccurate conclusions about performance bottlenecks.
- Ignoring Context: Don't just look at the top-level functions. Dive deeper into the call graph to understand the context of the bottlenecks. A seemingly insignificant function might be called millions of times within a critical loop.
- Misinterpreting Results: Understand the different types of profiles and their limitations. CPU profiles show CPU usage, while memory profiles show memory allocation. Choosing the wrong profile type can lead to incorrect interpretations.
- Sampling Rate: The sampling rate affects the accuracy and detail of the profile. A higher sampling rate provides more detailed information but generates larger profiles and might slow down the application. A lower sampling rate might miss less frequent but significant bottlenecks. Experiment to find a good balance.
-
Not considering external factors: Network I/O, database calls, and other external factors can significantly impact performance.
pprof
helps identify bottlenecks within your application, but it's crucial to consider these external factors as well.
How to Interpret the Output of pprof to Effectively Debug Performance Issues
Interpreting pprof
output requires understanding its various views and metrics. The most common views are:
- Top: Shows the functions consuming the most CPU time or memory, ranked in descending order. This provides a quick overview of the major performance hotspots.
- Flat: Similar to "top," but shows only the cumulative time spent in each function, without considering its callees.
- Call Graph: A graphical representation of the call stack, showing how functions call each other and the time spent in each function. This is crucial for understanding the context of bottlenecks and identifying chains of expensive calls.
- Source View: Shows the source code with annotations indicating the time spent on each line. This helps pinpoint specific code sections causing performance issues.
When interpreting the data, pay attention to:
- Cumulative time: The total time spent in a function, including the time spent in its callees.
- Self time: The time spent only within the function itself, excluding the time spent in its callees.
- Number of calls: The frequency with which a function is called. A function with a high number of calls, even if its self time is low, can still contribute significantly to overall performance issues.
By analyzing these metrics across different views, you can effectively identify and debug performance bottlenecks.
Which Profiling Techniques are Most Suitable for Different Types of Performance Bottlenecks
Go offers several profiling techniques beyond CPU and memory profiling:
-
CPU Profiling: Ideal for identifying bottlenecks related to excessive computation. Use
pprof
's CPU profile for this. -
Memory Profiling: Useful for identifying memory leaks, excessive allocations, or inefficient memory usage. Use
pprof
's heap profile for this. -
Block Profiling: Identifies contention points due to blocking operations (e.g., mutexes, channels). This helps optimize concurrency. Use
go tool pprof
with the block profile. -
Mutex Profiling: Focuses specifically on mutex contention. Use
go tool pprof
with the mutex profile. -
Trace Profiling: Provides a detailed trace of the application's execution, including function calls, timings, and context switches. This is more resource-intensive but offers a comprehensive view of the execution flow. Use
go tool trace
for this.
The choice of profiling technique depends on the suspected type of bottleneck:
- Slow response times: Start with CPU profiling.
- High memory usage: Use memory profiling.
- Concurrency issues: Use block or mutex profiling.
- Complex performance problems requiring a detailed view: Use trace profiling.
Often, a combination of profiling techniques is necessary for a thorough analysis. Start with simpler techniques like CPU and memory profiling, and then resort to more advanced techniques like trace profiling if needed. Remember to always profile with a representative workload and analyze the results carefully to identify the root cause of the performance problem.
The above is the detailed content of How do I use profiling tools like pprof to identify performance bottlenecks in Go?. For more information, please follow other related articles on the PHP Chinese website!
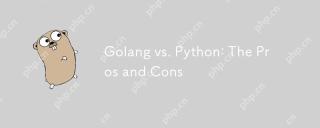
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
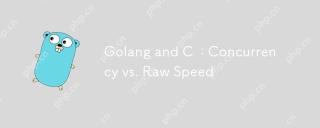
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
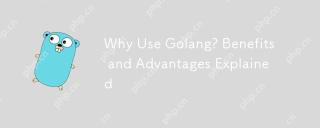
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
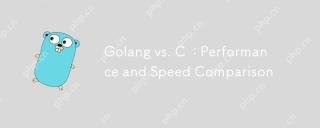
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
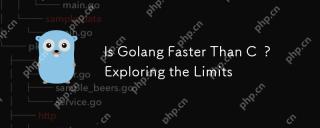
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
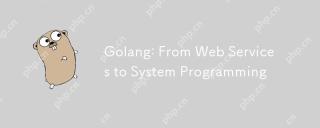
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
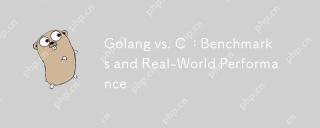
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
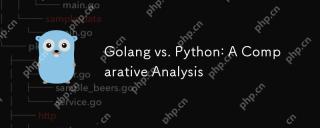
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
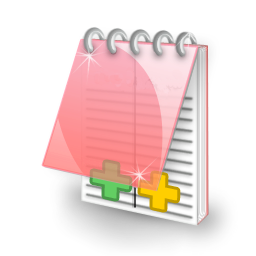
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
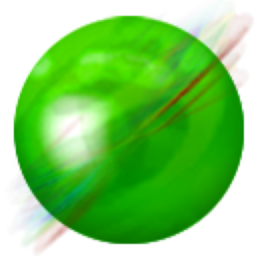
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
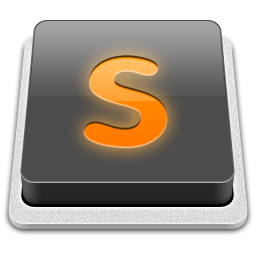
SublimeText3 Mac version
God-level code editing software (SublimeText3)