Python provides a variety of ways to download files from the Internet, which can be downloaded via HTTP using the urllib
package or the requests
library. This tutorial will explain how to use these libraries to download files from URLs from Python.
requests
Library
requests
is one of the most popular libraries in Python. It allows sending HTTP/1.1 requests without manually adding query strings to URLs or form encoding of POST data.
requests
The library can perform many functions, including:
- Add form data
- Add multi-part file
- Access Python response data
Send a request
First of all, you need to install the library, the method is very simple:
pip install requests
To test whether the installation is successful, you can simply enter the following command in the Python interpreter:
import requests
If the installation is successful, no error will occur.
HTTP requests include:
- GET
- POST
- PUT
- DELETE
- OPTIONS
- HEAD
Send a GET request
Sending a request is very simple, as shown below:
import requests req = requests.get("https://www.google.com")
The above command will get the Google page and store the information in status_code
.
import requests req = requests.get("https://www.google.com") req.status_code 200 # 200 表示请求成功
What if you want to know the encoding type of Google web page?
req.encoding 'ISO-8859-1'
Maybe I would like to know what the response is:
req.text
This is just a truncated part of the response content.
<code>'<meta content="Search the world\'s information, including webpages, imag...'</code>
Send POST request
Simply put, POST requests are used to create or update data, especially for form submissions.
Suppose there is a registration form and you need to enter your email address and password. When you click the Register Submit button, the POST request looks like this:
data = {" email req='requests.post("http://www.google.com",' params="data)</pre"> <p>Send PUT request</p> <p>PUT request is similar to a POST request and is used to update data. For example, the following API shows how to issue a PUT request: </p> <pre class="brush:php;toolbar:false">data = {"name": "tutsplus", "telephone": "12345"} r.put("http://www.contact.com", params=data)
Send DELETE request
As the name implies, a DELETE request is used to delete data. Here is an example of a DELETE request:
data = {'name': 'Tutsplus'} url = "https://www.contact.com/api/" response = requests.delete(url, params=data)
urllib
Package
urllib
package collects multiple modules for processing URLs:
-
urllib.error
Contains the exception raised byurllib.parse
to parse URL -
robots.txt
File
urllib.request
As shown below:
import urllib.request with urllib.request.urlopen('http://python.org/') as response: html = response.read()
If you want to retrieve and store Internet resources, you can do it through the urlretrieve()
function:
import urllib.request filename, headers = urllib.request.urlretrieve('http://python.org/') html = open(filename)
Use Python to download images
In this example, the requests
library and urllib
module are used to download this example image.
url = 'https://www.python.org/static/opengraph-icon-200x200.png' # 使用 urllib 下载 # 导入 urllib 库 import urllib.request # 将网络对象复制到本地文件 urllib.request.urlretrieve(url, "python.png") # 使用 requests 下载 # 导入 requests 库 import requests # 以二进制格式下载 url 内容 r = requests.get(url) # open 方法打开系统上的文件并写入内容 with open("python1.png", "wb") as code: code.write(r.content)
Use Python to download PDF files
In this example, a PDF file about Google Trends is downloaded.
url = 'https://static.googleusercontent.com/media/www.google.com/en//googleblogs/pdfs/google_predicting_the_present.pdf' # 使用 urllib 下载 # 导入 urllib 包 import urllib.request # 将网络对象复制到本地文件 urllib.request.urlretrieve(url, "tutorial.pdf") # 使用 requests 下载 # 导入 requests 库 import requests # 以二进制格式下载文件内容 r = requests.get(url) # open 方法打开系统上的文件并写入内容 with open("tutorial1.pdf", "wb") as code: code.write(r.content)
Use Python to download Zip files
In this example, the contents of the GitHub repository are downloaded and the files are stored locally.
pip install requests
Use Python to download videos
In this example, a video lecture will be downloaded.
import requests
Use Python to download CSV files
You can also use the requests
and urllib
libraries to download CSV files and use the csv
module to process the response. Let's use some example CSV address data.
import requests req = requests.get("https://www.google.com")
Conclusion
This tutorial introduces the most commonly used file download methods and the most common file formats. Although less code is written when using the urllib
module, the .netrc
module is more recommended due to its simplicity, popularity, and many additional features including: keep-alive and connection pooling, sessions with cookie persistence, browser-style SSL verification, automatic content decoding, authentication, automatic decompression, Unicode response body, HTTP(S) proxy support, multipart file upload, streaming download, connection timeout, chunked request, requests
support).
The above is the detailed content of How to Download Files in Python. For more information, please follow other related articles on the PHP Chinese website!
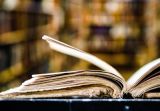
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
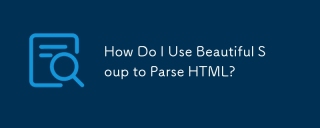
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
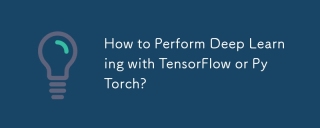
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
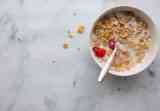
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
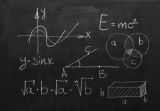
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
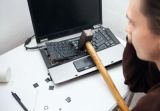
In this tutorial you'll learn how to handle error conditions in Python from a whole system point of view. Error handling is a critical aspect of design, and it crosses from the lowest levels (sometimes the hardware) all the way to the end users. If y
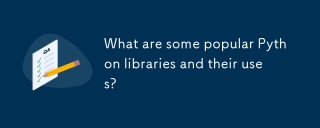
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
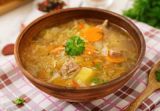
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
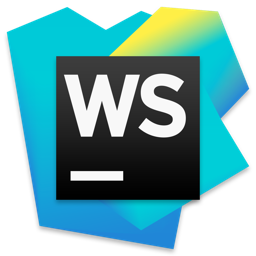
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
