


Parallel Processing with Asynchronous Lambda Functions
Combining parallel processing and asynchronous lambda functions requires careful consideration. This article addresses the challenges of using async
methods within Parallel.ForEach
and offers effective solutions.
The Problem with Parallel.ForEach and Async Lambdas
Parallel.ForEach
launches background threads that don't inherently wait for task completion. This creates problems when using await
inside the lambda expression. The following example illustrates this issue:
var bag = new ConcurrentBag<object>(); Parallel.ForEach(myCollection, async item => { // some pre-processing var response = await GetData(item); bag.Add(response); // some post-processing }); var count = bag.Count; // count will be 0
The count
will be 0 because the main thread continues execution before the background threads complete their asynchronous operations. Using Wait()
as a workaround, as shown below, negates the benefits of await
and requires explicit exception handling:
var bag = new ConcurrentBag<object>(); Parallel.ForEach(myCollection, item => { // some pre-processing var responseTask = GetData(item); responseTask.Wait(); var response = responseTask.Result; bag.Add(response); // some post-processing }); var count = bag.Count;
Solution Using Task.WhenAll
A more efficient solution involves leveraging Task.WhenAll
to manage the asynchronous operations:
var bag = new ConcurrentBag<object>(); var tasks = myCollection.Select(async item => { // some pre-processing var response = await GetData(item); bag.Add(response); // some post-processing }); await Task.WhenAll(tasks); var count = bag.Count; // count will be correct
This approach allows each item to be processed asynchronously, and Task.WhenAll
ensures all tasks complete before proceeding.
Advanced Solution: ForEachAsync
For more sophisticated asynchronous parallel processing, explore Stephen Toub's blog post on ForEachAsync
. This provides a robust and flexible solution for handling complex scenarios.
The above is the detailed content of How Can I Properly Use Asynchronous Lambda Functions with Parallel.ForEach?. For more information, please follow other related articles on the PHP Chinese website!
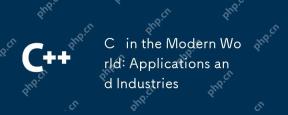
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
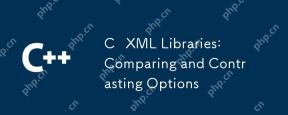
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
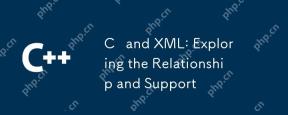
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
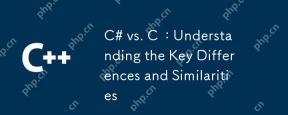
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
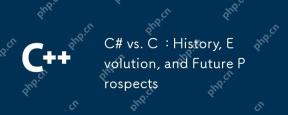
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
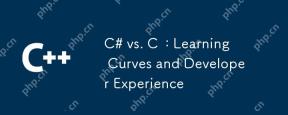
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
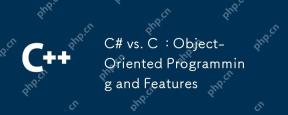
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
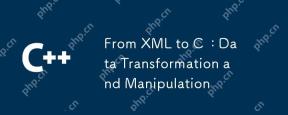
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
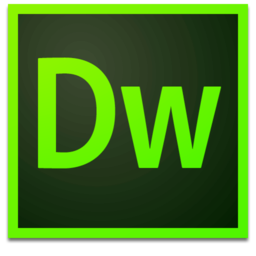
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
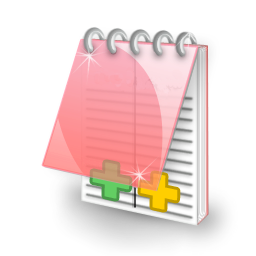
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function