QR codes have become an essential tool in today’s digital world, bridging the gap between physical and digital spaces. Whether you’re sharing a website link, contact information, or even Wi-Fi credentials, QR codes make it effortless. In this article, we’ll dive into a Python-based QR code generator, explain the code step-by-step, and show you how to create your own custom QR codes. Let’s get started!
Why Use Python to Generate QR Codes?
Python is a versatile programming language that makes it easy to automate tasks, including generating QR codes. With just a few lines of code, you can create QR codes that are not only functional but also visually appealing. The best part? You can customize the colors and size to match your brand or personal style.
Step-by-Step Guide to Generating QR Codes
Below, we’ll walk you through the Python code for generating QR codes and explain how to use it effectively.
1. Install the Required Libraries
Before you start, you’ll need to install the necessary Python libraries. Open your terminal or Jupyter Notebook and run the following command:
!pip install qrcode[pil]
This command installs the qrcode library, which is used to generate QR codes, and PIL (Pillow), which handles image processing.
2. Import the Required Modules
Once the libraries are installed, import the necessary modules in your Python script or notebook:
import qrcode from PIL import Image from IPython.display import display
- qrcode: The main library for generating QR codes.
- PIL.Image: Used to handle image creation and manipulation.
- IPython.display: Helps display the QR code directly in a Jupyter Notebook.
3. Define the QR Code Generation Function
Next, we define a function called generate_qr_code that takes three parameters:
- link: The URL or text you want to encode in the QR code.
- fill_color: The color of the QR code (default is black).
- back_color: The background color of the QR code (default is white).
Here’s the function:
def generate_qr_code(link, fill_color='black', back_color='white'): """ Generates a QR code from the given link and displays it in the notebook. :param link: The URL or text to encode in the QR code. :param fill_color: The color of the QR code (default is 'black'). :param back_color: The background color of the QR code (default is 'white'). """ # Create a QR code instance qr = qrcode.QRCode( version=1, # Controls the size of the QR Code (1 is the smallest, 40 is the largest) error_correction=qrcode.constants.ERROR_CORRECT_L, # Error correction level box_size=10, # Size of each box in the QR code border=4, # Border size around the QR code ) # Add data to the QR code qr.add_data(link) qr.make(fit=True) # Create an image from the QR code instance img = qr.make_image(fill_color=fill_color, back_color=back_color) # Display the image in the notebook display(img)
4. Customize and Generate Your QR Code
To generate a QR code, simply call the generate_qr_code function. Here’s how you can do it:
!pip install qrcode[pil]
5. Run the Code
- Save the script as qr_code_generator.py.
- Run the script in your terminal or Jupyter Notebook.
- Enter the link you want to encode when prompted.
- Optionally, customize the fill color and background color.
- Voilà! Your QR code will be generated and displayed.
How Does the Code Work?
Let’s break down the key components of the code:
-
QRCode Instance: The qrcode.QRCode class is used to create a QR code object. You can customize its size, error correction level, and border.
- version: Controls the size of the QR code (1 is the smallest, 40 is the largest).
- error_correction: Determines how much of the QR code can be damaged while still being readable. ERROR_CORRECT_L allows for about 7% damage recovery.
- box_size: Defines the size of each "box" in the QR code.
- border: Specifies the width of the border around the QR code.
Adding Data: The add_data method encodes the provided link or text into the QR code.
Creating the Image: The make_image method generates the QR code as an image, with customizable colors.
Displaying the Image: The display function shows the QR code directly in your Jupyter Notebook.
Customization Options
One of the best features of this QR code generator is its flexibility. You can:
- Change the fill color and background color to match your branding.
- Adjust the box size and border to make the QR code larger or smaller.
- Use different error correction levels depending on your needs.
Practical Applications
Here are some ways you can use this QR code generator:
- Marketing: Share your website, social media profiles, or promotional offers.
- Contactless Payments: Generate QR codes for payment links.
- Wi-Fi Sharing: Create QR codes that automatically connect users to your Wi-Fi network.
- Event Management: Use QR codes for ticketing or check-ins.
Conclusion
Generating QR codes with Python is simple, fast, and highly customizable. With the code provided in this article, you can create QR codes for any purpose, whether personal or professional. So why wait? Start generating your own QR codes today and unlock a world of possibilities!
Pro Tip: Bookmark this article for future reference, and share it with your friends who might find it useful. Happy coding! ?
For Non-Tech You Can directly copy and Paste in Google Colab
!pip install qrcode[pil]
Author Credits:

ChemEnggCalc - Learn Chemical Engineers Calculations with Tools & Tech
Learn Chemical Engineers Calculations with Tools & Tech
The above is the detailed content of Create Stunning QR Codes in Seconds with Python – Here's How!. For more information, please follow other related articles on the PHP Chinese website!
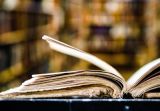
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
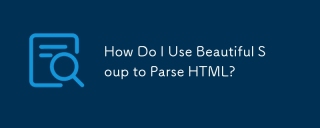
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
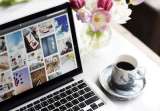
Dealing with noisy images is a common problem, especially with mobile phone or low-resolution camera photos. This tutorial explores image filtering techniques in Python using OpenCV to tackle this issue. Image Filtering: A Powerful Tool Image filter
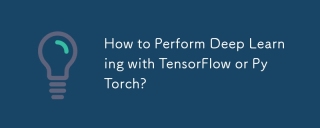
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
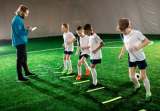
Python, a favorite for data science and processing, offers a rich ecosystem for high-performance computing. However, parallel programming in Python presents unique challenges. This tutorial explores these challenges, focusing on the Global Interprete
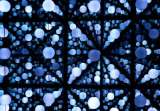
This tutorial demonstrates creating a custom pipeline data structure in Python 3, leveraging classes and operator overloading for enhanced functionality. The pipeline's flexibility lies in its ability to apply a series of functions to a data set, ge
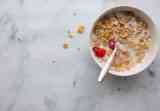
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
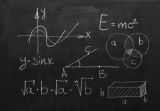
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
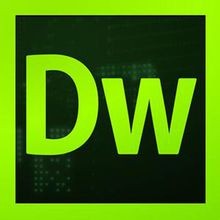
Dreamweaver CS6
Visual web development tools
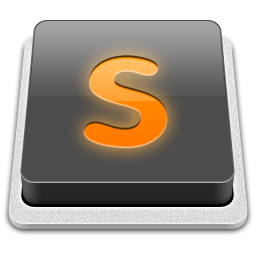
SublimeText3 Mac version
God-level code editing software (SublimeText3)
