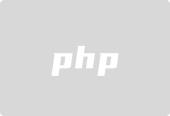
Implementing concurrent HashSet in .NET Framework?
Introduction:
The .NET Framework does not provide a built-in implementation of a concurrent HashSet, which can be a challenge for developers working with concurrent data structures. This article explores the need for concurrent HashSet and examines various ways to implement thread-safe access to HashSet in the .NET Framework.
Custom thread safety implementation:
One approach is to create a custom thread-safe HashSet implementation. This can be achieved by wrapping an existing HashSet in a synchronization mechanism (such as a lock). The following code snippet demonstrates this implementation:
<code class="language-C#">public class ConcurrentHashSet<T>
{
private readonly HashSet<T> _hashSet = new HashSet<T>();
private readonly object _syncRoot = new object();
public bool Add(T item)
{
lock (_syncRoot)
{
return _hashSet.Add(item);
}
}
public bool Remove(T item)
{
lock (_syncRoot)
{
return _hashSet.Remove(item);
}
}
// 其他操作可以类似地实现
}</code>
Use ConcurrentDictionary:
Another approach is to utilize the ConcurrentDictionary class in the System.Collections.Concurrent namespace. While it doesn't provide exactly the same functionality as HashSet, it provides a thread-safe dictionary with similar semantics. By using key-value pairs (where keys are elements in a HashSet and values are dummy values) we can achieve concurrent implementation:
<code class="language-C#">private ConcurrentDictionary<T, byte> _concurrentDictionary = new ConcurrentDictionary<T, byte>();
public bool Add(T item)
{
byte dummyValue = 0;
return _concurrentDictionary.TryAdd(item, dummyValue);
}
public bool Remove(T item)
{
byte dummyValue;
return _concurrentDictionary.TryRemove(item, out dummyValue);
}
// 其他操作可以类似地实现</code>
Note:
When choosing a method, consider the following factors:
-
Concurrency Safety: Both methods provide thread-safe access to the underlying data structures.
-
Performance: In some cases, a custom implementation may have better performance than ConcurrentDictionary.
-
Simplicity: ConcurrentDictionary provides a simpler and more direct implementation.
Applicability of -
HashSet: ConcurrentDictionary does not inherit from HashSet, so some HashSet-specific functionality may be lost.
Conclusion:
The need for concurrent HashSets in the .NET Framework can be solved by implementing a custom thread-safe wrapper or using ConcurrentDictionary. The choice between the two depends on the specific requirements of the application and the trade-offs mentioned above.
The above is the detailed content of How to Implement a Concurrent HashSet in the .NET Framework?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn